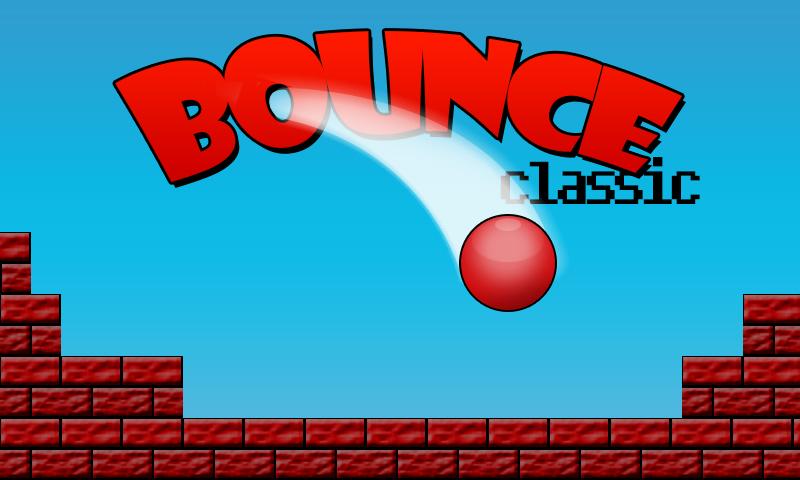
順番に始めましょう。 不要なものをすべてペイントするのではなく、特定の瞬間にコメントするコードを単純に調べます。
1つ目は、メインの実行可能ファイルであるindex.htmlです。
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> <meta name="viewport" content="width=device-width,user-scalable=no"/> <title>Bounce</title> </head> <body> <script type="text/javascript" src="point.js"></script> <script type="text/javascript" src="init.js"></script> <script type="text/javascript" src="menu.js"></script> <script type="text/javascript" src="game.js"></script> <script type="text/javascript"> game.startLoop('menu'); </script> </body> </html>
→ githubで見る
ここでは、すべてが明確だと思います。ページフレームを作成し、いくつかのJavaScriptファイルを接続しました。
- point.js-PointJSエンジン
- init.js-すべての初期化+すべてのグローバル変数
- menu.js-PointJSのプラグイン-メニュー
- game.js-ゲームの仕組みを説明するファイル
init.jsファイルを検討してください。
// , pjs var pjs = new PointJS('2D', 400, 400, { backgroundColor : '#C9D6FF' }); // pjs.system.initFullPage(); // var log = pjs.system.log; // var game = pjs.game; // var point = pjs.vector.point; // var camera = pjs.camera; / var brush = pjs.brush; / var OOP = pjs.OOP; / var math = pjs.math; / // var key = pjs.keyControl.initKeyControl(); var mouse = pjs.mouseControl.initMouseControl(); // var score = 0; var record = 0;
→ githubで見る
これでコードが判明しましたが、これで十分です。
ここで、ゲームのメニューを作成する必要があります。「メニュー」サイクルからゲームを開始するため、これに必要なものはすべて、接続したmenu.jsファイルにあります。対応するゲームサイクルを開始するだけです。同じmenu.jsファイル、次を追加するだけです:
game.newLoopFromClassObject('menu', new Menu(pjs, { name : 'Bounce', // ( ) author : 'SkanerSoft', // radius : 15, // items : { // , : loopName : game : ' ', // game about : ' ', // about } }));
→ githubでmenu.jsファイル全体を見る
実行すると、起動されたメニューが表示されます。

今最も難しいのはゲームプレイです。 ここではすべてが非常に単純ですが、完全ではありません。 コードをブロックに分割します。
最初に行う必要があるのは、ゲームループの宣言です。 独自のスコープを持つ独立したオブジェクトとして使用し、グローバルにする必要がないため(ゲームの状態データは他のゲームサイクルでは表示されません)、単純な構造を使用します。
game.newLoopFromConstructor('game', function () { /*your code*/ });
ここで、ゲームループの名前とコンストラクターを設定できます。
それでは、コンストラクターの内部に移動して、内部のレベルのロジック(メカニクス)を記述しましょう。 現在、必要なループイベントは、updateのみです。 私たちは彼と一緒に仕事をします。
game.newLoopFromConstructor('game', function () { // var map = { width : 50, // height : 50, // source : [ // ( ) '', '', ' 0-', ' | P 0000', // P - ' 00000 000 00000', ' 0 0| |0 |', '0000000 000000W00 000000000000', ' 000 0W00 0 ', ' 0W0 0 ', ' 0W | 0 ', ' 000000 ', ] }; // () var plStartPosition = false; var walls = []; // (, ) var cells = []; // (, ) var waters = []; // ( ), // OOP.forArr - OOP.forArr(map.source, function (string, Y) { // (Y - ) OOP.forArr(string, function (symbol, X) { // (X - ) if (!symbol || symbol == ' ') return; // - // if (symbol == 'P') { // // plStartPosition = point(map.width*X, map.height*Y); // , } else if (symbol == 'W') { // waters.push(game.newRectObject({ // w : map.width, h : map.height, // x : map.width*X, y : map.height*Y, // fillColor : '#084379', // alpha : 0.5 // })); } else if (symbol == '|') { // () cells.push(game.newRectObject({ w : map.width/2, h : map.height, x : map.width*X, y : map.height*Y, fillColor : '#FFF953', userData : { active : true // , - } })); } else if (symbol == '-') { // cells.push(game.newRectObject({ w : map.width, h : map.height/2, x : map.width*X, y : map.height*Y, fillColor : '#FFF953', userData : { active : true } })); } else if (symbol == '0') { // walls.push(game.newRectObject({ w : map.width, h : map.height, x : map.width*X, y : map.height*Y, fillColor : '#B64141' })); } }); }); // // , , // , var player = game.newCircleObject({ radius : 20, fillColor : '#FF9191', position : plStartPosition ? plStartPosition : point(0, 0) }); player.gr = 0.5; // player.speed = point(0, 0); // // this.update = function () { game.clear(); // player.draw(); // player.speed.y += player.gr; // // , , if (key.isDown('RIGHT')) player.speed.x = 2; else if (key.isDown('LEFT')) player.speed.x = -2; else player.speed.x = 0; // OOP.drawArr(walls, function (wall) { if (wall.isInCameraStatic()) { // ( ) // wall.drawStaticBox(); if (wall.isStaticIntersect(player)) { // // ( ) // Y if (player.x+player.w > wall.x+wall.w/4 && player.x < wall.x+wall.w-wall.w/4) { if (player.speed.y > 0 && player.y+player.h < wall.y+wall.h/2) { // if (key.isDown('UP')) // "" player.speed.y = -10; // else { // "" player.y = wall.y - player.h; player.speed.y *= -0.3; if (player.speed.y > -0.3) player.speed.y = 0; // } } else if (player.speed.y < 0 && player.y > wall.y+wall.h/2) { // player.y = wall.y+wall.h; // ( ) player.speed.y *= -0.1; // } } // , X if (player.y+player.h > wall.y+wall.h/4 && player.y < wall.y+wall.h-wall.h/4) { if (player.speed.x > 0 && player.x+player.w < wall.x+wall.w/2) { // player.x = wall.x-player.w; // player.speed.x = 0; // } if (player.speed.x < 0 && player.x > wall.x+wall.w/2) { // player.x = wall.w+wall.x; // player.speed.x = 0; // } } } } }); // () OOP.drawArr(cells, function (cell) { if (cell.active) { // if (cell.isStaticIntersect(player)) { // cell.active = false; // cell.fillColor = '#9A9A9A'; // score++; // } } }); // , // var onWater = false; // OOP.drawArr(waters, function (water) { // , if (onWater) return; // // () // // , // , // if (water.isStaticIntersect(player) && player.y+player.h/2 > water.y) { player.speed.y -= 0.9; // onWater = true; } }); // if (player.speed.y) { player.y += player.speed.y; } if (player.speed.x) { player.x += player.speed.x; } // brush.drawTextS({ // text : 'Score: '+score, // size : 30, // color : '#FFFFFF', // strokeColor : '#002C5D', // strokeWidth : 1, // x : 10, y : 10, // style : 'bold' // }); camera.follow(player, 50); // }; });
→ githubでファイルを見る
さて、実行してゲームに入ると、次のように表示されます。

したがって、ゲームの単純なメカニズムを構築し、新しい要素を追加することはそれほど難しくなく、処理に合わせるのも同様です。
物理アルゴリズム自体を変更したり、係数を変更したりして、衝突フィールドを狭めたり広げたりすることができます。 また、キャラクターの速度を基本として、動的にすることもできます。
→ githubでBounceプロジェクトのソースコードを表示する
→ 実例を実行する
20分のゲームの物理アルゴリズムを開発するビデオ
ゲーム物理学
新しいオブジェクトを使用したゲームの追加:
新しいオブジェクトを使用したゲームの追加: