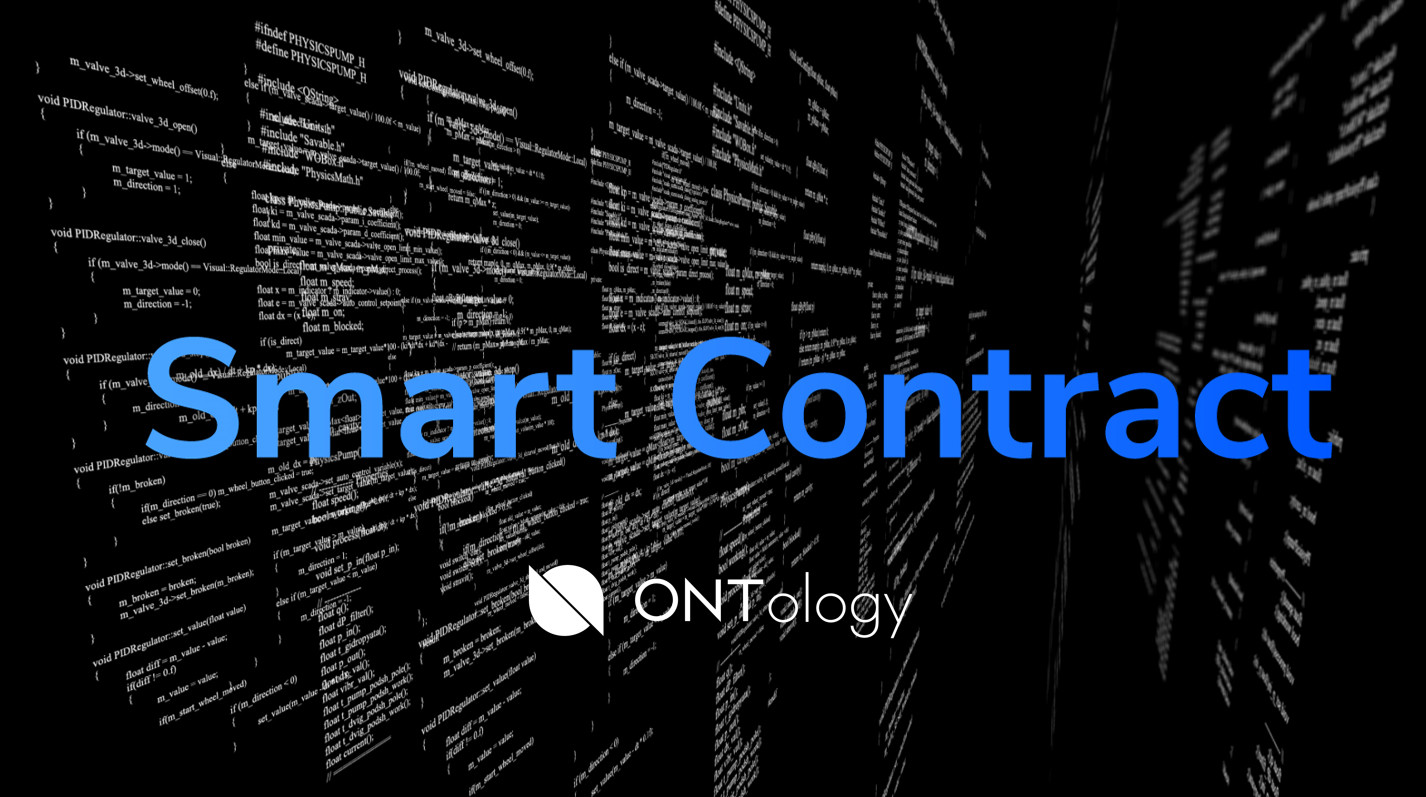
This is the second part of a series of tutorials on creating smart contracts in Python on the Ontology blockchain network. In a previous article, we met with the Ontology Blockchain & Block API smart contract API .
Today we will discuss how to use the second module — the Storage API . The Storage API has five related APIs that allow adding, deleting and modifying persistent storage in smart contracts on the blockchain.
Below is a brief description of these five APIs:
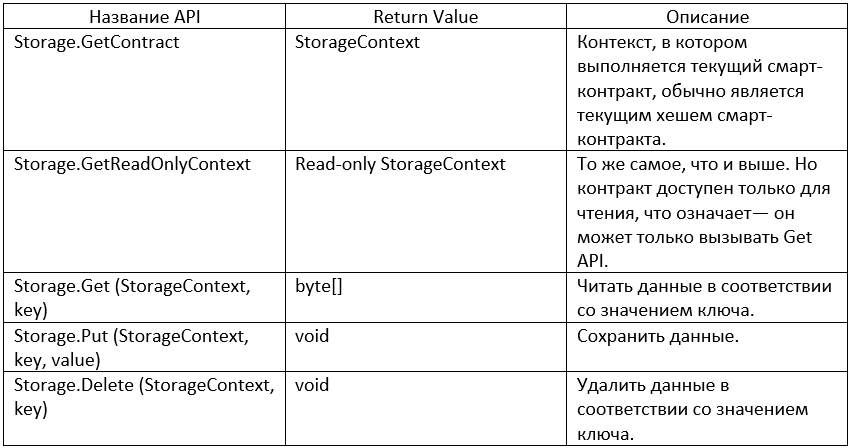
Let's take a closer look at how to use these five APIs.
0. Create a new SmartX contract
1. How to use Storage API
GetContext & GetReadOnlyContext
GetContext and GetReadOnlyContext get the context in which the current smart contract is executed. Return value is the inverse of the current hash of the smart contract. As the name suggests, GetReadOnlyContext takes a read-only mode context. In the example below, return value is the inverse of the contract hash displayed in the upper right corner.
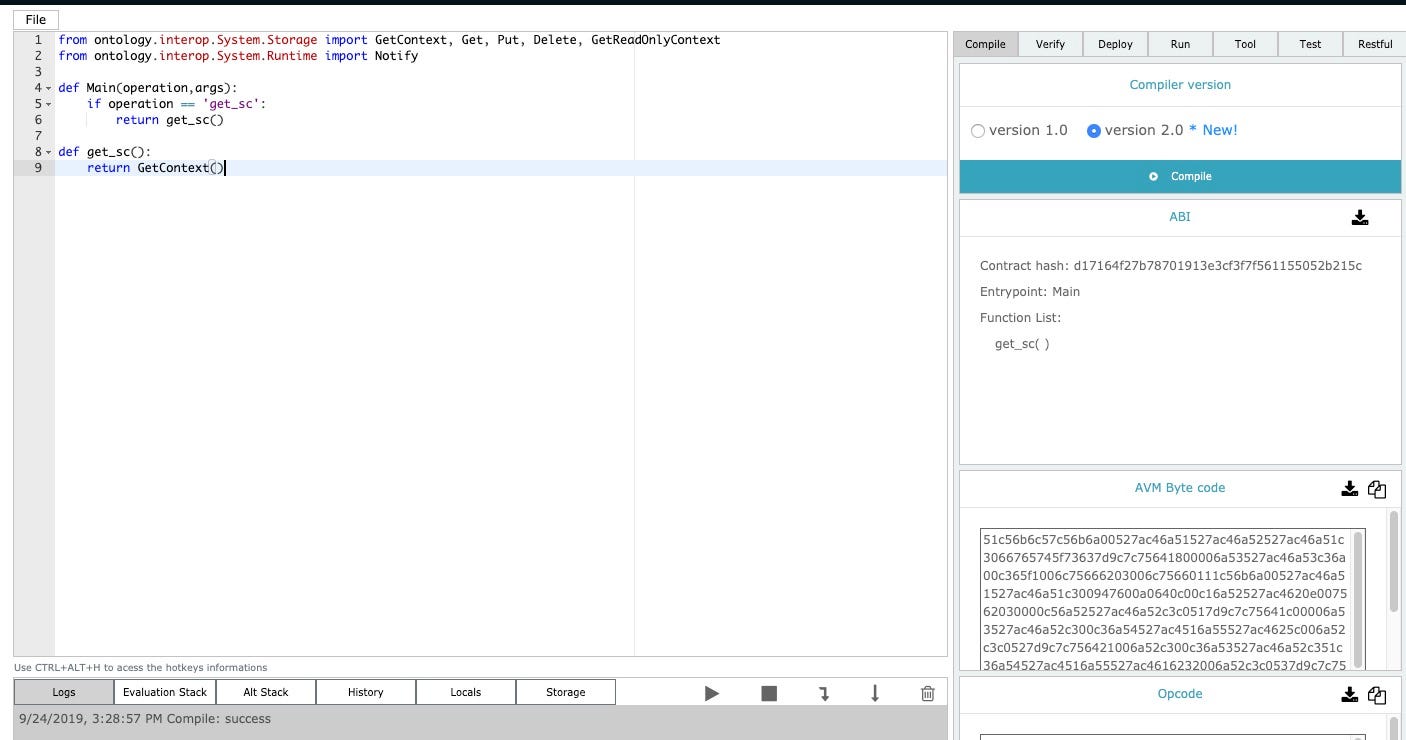
Put
The Put function is responsible for storing data on the blockchain in the form of a dictionary. As shown, Put takes three parameters. GetContext takes the context of the currently running smart contract, key is the key value that is needed to save the data, and value is the value of the data that needs to be saved. Please note that if the key value is already in the repository, the function will update its corresponding value.
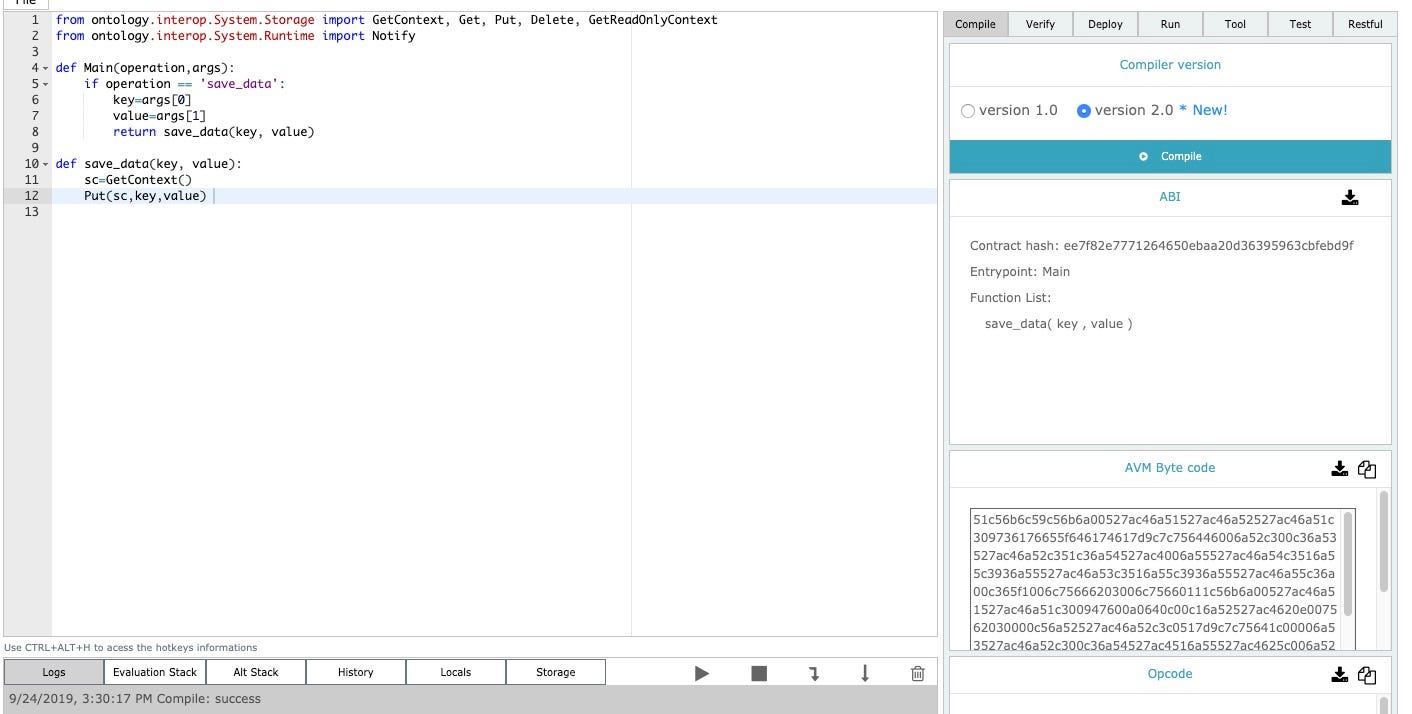
Get
The Get function is responsible for reading data in the current blockchain by means of a key value. In the example below, you can fill in the key value in the parameters panel on the right to execute the function and read the data corresponding to the key value in the blockchain.
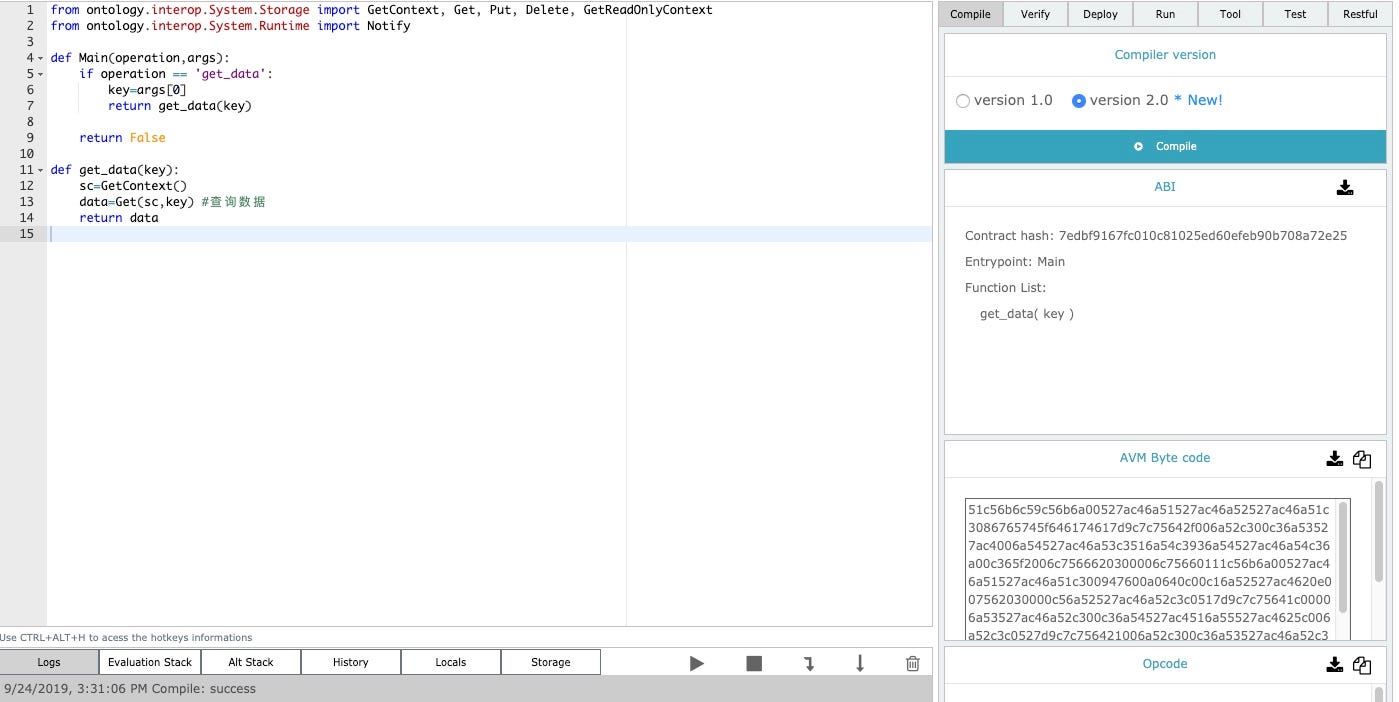
Delete
The Delete function is responsible for deleting data in the blockchain by means of a key value. In the example below, you can fill in the key value to execute the function in the parameters panel on the right and delete the data corresponding to the key value in the blockchain.
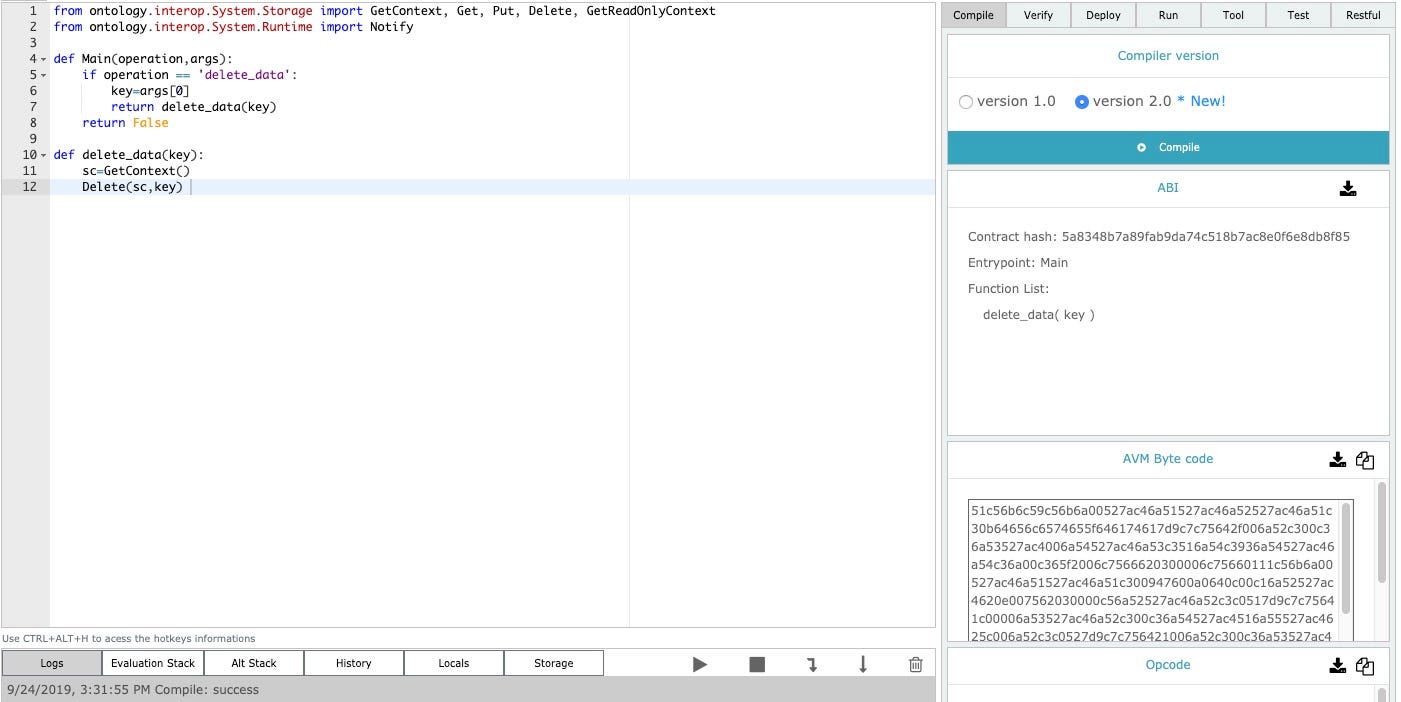
2. Sample Storage API Code
The code below gives a detailed example of using the five APIs: GetContext, Get, Put, Delete and GetReadOnlyContext. You can try running the API data in SmartX .
from ontology.interop.System.Storage import GetContext, Get, Put, Delete, GetReadOnlyContext from ontology.interop.System.Runtime import Notify def Main(operation,args): if operation == 'get_sc': return get_sc() if operation == 'get_read_only_sc': return get_read_only_sc() if operation == 'get_data': key=args[0] return get_data(key) if operation == 'save_data': key=args[0] value=args[1] return save_data(key, value) if operation == 'delete_data': key=args[0] return delete_data(key) return False def get_sc(): return GetContext() def get_read_only_sc(): return GetReadOnlyContext() def get_data(key): sc=GetContext() data=Get(sc,key) return data def save_data(key, value): sc=GetContext() Put(sc,key,value) def delete_data(key): sc=GetContext() Delete(sc,key)
Afterword
Blockchain storage is the core of the entire blockchain system. The Ontology Storage API is easy to use and convenient for developers.
On the other hand, hacker attacks are focused on the storage, for example, the security risk that we mentioned in one of the previous articles — storage injection attack , developers must pay special attention to security when writing code that is associated with the storage. You can find the complete guide on our github here.
In the next article, we will discuss how to use the Runtime API .
The article was translated by Hashrate & Shares specifically for OntologyRussia. cry
Are you a developer? Join our tech community on Discord . Also, check out the Ontology Developer Center for more tools, documentation, and more.
Open tasks for developers. Close the task - get a reward.
Apply for Ontology Student Talent Program
Ontology
Ontology website - GitHub - Discord - Telegram Russian - Twitter - Reddit