How to create your first JavaFX application.
This is the second post in the JavaFX series. You can read the first article on how to set up your JavaFx development environment.
All posts in the JavaFX series:
- JavaFX Tutorial: Getting Started
- JavaFX Tutorial: Hello World!
- JavaFX Tutorial: FXML and SceneBuilder
- JavaFX Tutorial: Basic Layouts
- JavaFX Tutorial: Advanced Layouts
- JavaFX Tutorial: CSS styling
- JavaFX Weaver: Integrating JavaFX and Spring Boot Applications
Application structure
Each application consists of a hierarchy of several main components: Stages (windows), (scenes) and nodes (nodes). Let's look at each of them.
Stage
Stage is essentially a window. Your application may have several stage components, but at least one must be required.
Scene
Scene displays the contents of the scene (stage). Each stage component can contain multiple scene components that can be switched. Imagine the theatrical stage, on which several scenes are replaced during the performance.
Node
Each stage component can contain various components called nodes. Nodes can be controls, such as buttons or labels, or even layouts, which can contain multiple nested components. Each scene can have one sub-node, but it can be a layout that can contain several components. Nesting can be multi-level - layouts can contain other layouts and common components.
Summary
Each application can have several windows (Stage). Each Stage component can switch multiple Scene scenes. Scenes contain nodes — layouts and common components.
This hierarchy can be visualized as follows:
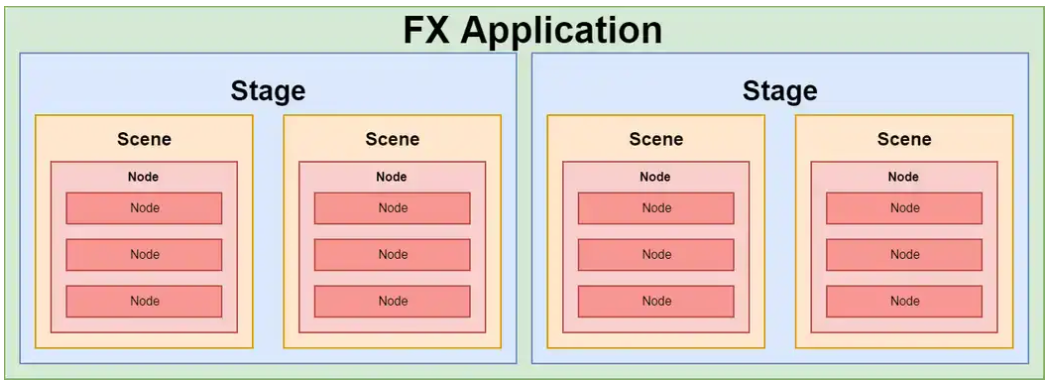
Now let's look at a specific example - a real application.
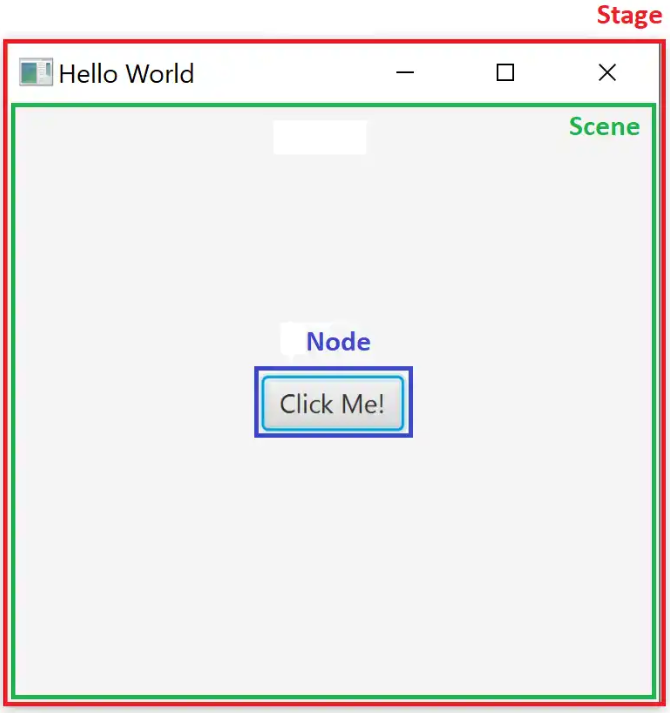
Class Application
Time to start programming. If you followed the previous article , you have all the necessary dependencies ready.
Each JavaFX application must have a main class that extends the class:
javafx.application.Application
In addition, you must override the abstract method from the Application class:
public void start(Stage primaryStage) throws Exception
Cass main looks something like this:
import javafx.application.Application; import javafx.stage.Stage; public class Main extends Application { @Override public void start(Stage primaryStage) throws Exception { // TODO implement me! } }
Main method
To run a JavaFx application, the main () method is not necessary. You can package the jar executable using the JavaFX Packager Tool . However, it is much more convenient to have a main method.
At the same time, the application is not only easier to run, but you can transfer command line parameters to it as usual.
Inside the main () method, the application can be launched using the method:
Application.launch()
It is easy to see that this is a static method in the Application class. We did not specify the main class, but JavaFX can determine this automatically depending on the class that calls this method.
Stage setup
Now we know how to start our application using the main () method. However, nothing will happen if we do this. We need a window that we want to show. The window is called stage, remember? In fact, we already have the primary stage passed to the start method as an input parameter:
public void start (Stage primaryStage)
We can use this component of stage. The only problem is that it is hidden by default. Fortunately, we can easily show it using the primaryStage.show () method:
@Override public void start(Stage primaryStage) throws Exception { primaryStage.show(); }
Now, when you start the application, you should see the following window:

Not very impressive, right? First, let's add a nice signature to our window.
primaryStage.setTitle("Hello world Application");
To make the window look even better, let's add a beautiful icon to the top panel of the window:
InputStream iconStream = getClass().getResourceAsStream("/icon.png"); Image image = new Image(iconStream); primaryStage.getIcons().add(image);
You can add several icons representing the application. More precisely, the same icon of different sizes. This will allow you to use an icon of a suitable size depending on the context of the application.
Now you can configure the properties and behavior of the Stage object, for example:
- Set position using setX () and setY ()
- Set initial size with setWidth () and setHeight ()
- Limit maximum window sizes with setMaxHeight () and setMaxWidth () or disable resizing with setResizable (false)
- Set window mode always on top using setAlwaysOnTop ()
- Set fullscreen mode using setFullScreen ()
- And much more
Now we have a window with such a fantastic name, but it is still empty. You already know that you cannot add components directly to the Stage (window). You need a scene.
However, to start the scene designer, you must specify a child node. Let's first create a simple label. Then we create a scene with this label as a child node.
Label helloWorldLabel = new Label("Hello world!"); Scene primaryScene = new Scene(helloWorldLabel);
You have noticed that Scene only allows one child component. What if we need more? You must use a layout, which is a component that can include several children and place them on the screen, depending on the layout used. We will cover layouts later in this series of articles.
To make the application a little more visually appealing, let's place a label in the center of the screen.
helloWorldLabel.setAlignment(Pos.CENTER);
Finally, we need to install Scene for Stage, which we already have:
@Override public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("Hello world Application"); primaryStage.setWidth(300); primaryStage.setHeight(200); InputStream iconStream = getClass().getResourceAsStream("/icon.png"); Image image = new Image(iconStream); primaryStage.getIcons().add(image); Label helloWorldLabel = new Label("Hello world!"); helloWorldLabel.setAlignment(Pos.CENTER); Scene primaryScene = new Scene(helloWorldLabel); primaryStage.setScene(primaryScene); primaryStage.show(); }
Now our window contains a scene with the label component:
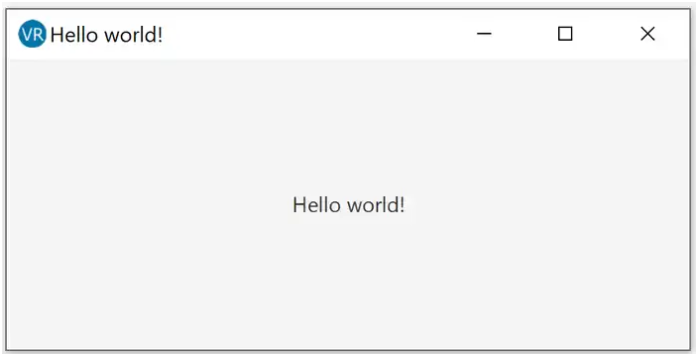
What's next
In the next step of this series, we will look at how to implement our GUI in XML, not in Java.