
A guide to using mergeMap and forkJoin instead of simple subscriptions for multiple API requests.
In this article, I will show two approaches to handling multiple queries in Angular using mergeMap and forkJoin.
Content:
- Problem
- subscribe
- mergeMap
- forkJoin
- Combining mergeMap and forkJoin
- Compare subscribe with mergeMap and forkJoin
Problem
In web application development, we often need more than one API call. You go to the page, make several requests, get the necessary data and the results of some of these requests are required for subsequent calls.
When we make such multiple requests, it is important to process them efficiently while maintaining a high level of performance and code quality.
I will show you a simple application where we need to make 3 requests to the test API ( https://jsonplaceholder.typicode.com ):
- Log in and request user information
- Based on the user information, we get a list of user posts
- Based on user information, we get a list of albums created by the user
subscribe is the usual way to handle requests in Angular, but there are more efficient methods. First, we will solve the problem using subscribe, and then we will improve the solution using mergeMap and forkJoin.
subscribe
Pretty simple way. We make the first request to the API. Then, in the nested subscription, so that you can use the first answer, we make two more requests to the API.

mergeMap
This operator is best used when we need to manually control the order of requests.
So when do we use mergeMap?
When the result of the first request to the API we need in order to do the following.

Look at an example, we see that for the second request we need userId from the response of the first call.
Note:
- flatMap - alias for mergeMap
- mergeMap supports multiple active internal subscriptions at the same time, so you can create a memory leak with such long-lived subscriptions
forkJoin
This operator is suitable if we need to make several queries and the result of each is important. That is, you can group several queries, run them in parallel and return only one observable.
So when do we use forkJoin?
When requests can be executed in parallel and not depend on each other.
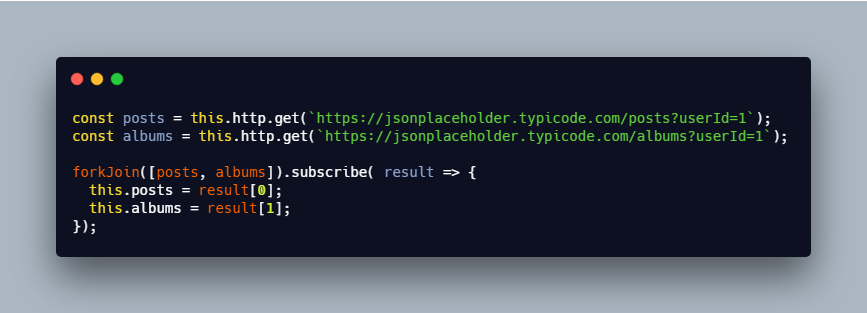
Combining mergeMap and forkJoin
Usually in development, we are faced with a situation when you need to make several requests that depend on the result of the execution of some other request. Let's see how this can be done using mergeMap and forkJoin.
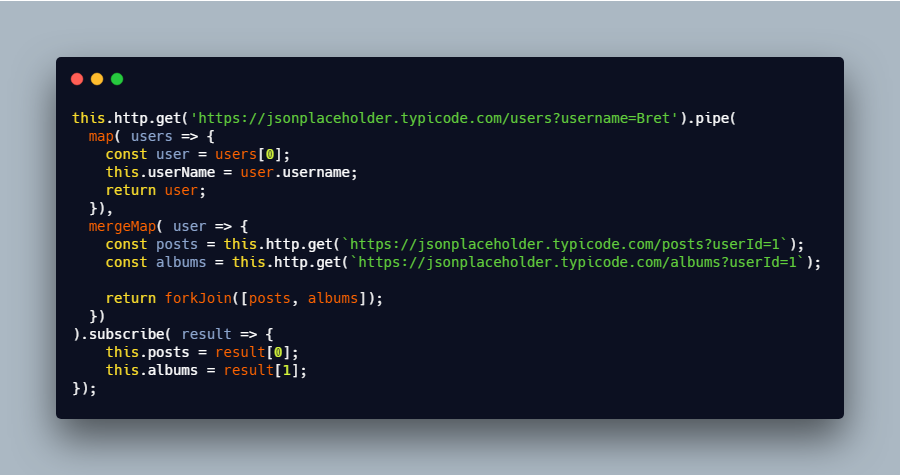
So we avoided nested subscriptions and broke the code into several small methods.
Comparing a regular subscription with mergeMap and forkJoin
The only difference I noticed is HTML parsing.
Let's look at the time that parsing took when using a regular subscription:
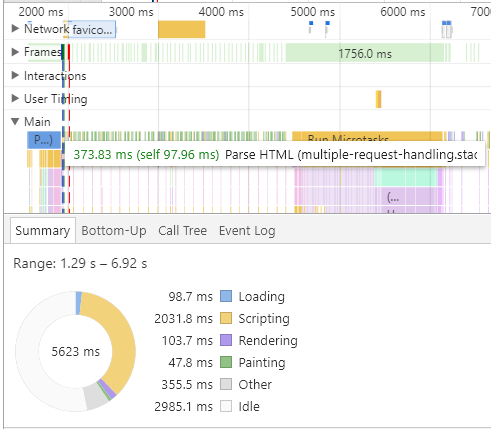
Now let's see how much HTML is parsed using mergeMap and forkJoin

I compared the result several times and came to the conclusion that parsing with mergeMap and forkJoin is always faster but the difference is not very big (~ 100ms).
The most important thing is a way to make the code more understandable and readable.
Summarizing
We can use RxJS to handle multiple requests in Angular. This helps us write more readable and supported code. Well, and as a bonus, we see a slight increase in performance if we use RxJS methods instead of regular subscriptions.
Hope the article was helpful! Follow me on Medium and Twitter . Feel free to comment and ask questions. I'll be glad to help!
Sources here .