詳細はこちら 。
フォームについても同じ原理で動作するライブラリを注目したい。
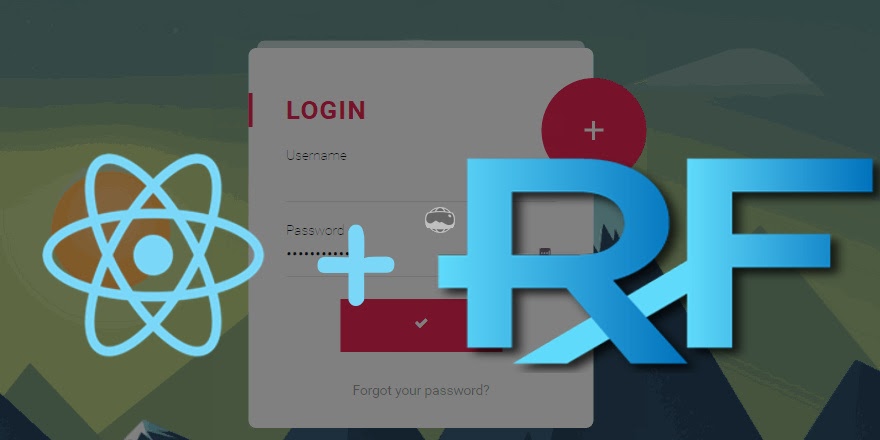
ドキュメントは英語です。 インストール:
npm install redux-form
アプリケーションに接続します。
import { createStore, combineReducers } from 'redux' import { reducer as formReducer } from 'redux-form' const reducers = { // form: formReducer // state form } const reducer = combineReducers(reducers) const store = createStore(reducer)
フォームを作成します。
import React, { Component } from 'react'; // (Field) (reduxForm) import { Field, reduxForm } from 'redux-form'; class Form extends Component { render(){ // handleSubmit // reset , // undefined, const {handleSubmit, reset} = this.props; const submit = (values) => console.log(values); return ( <form onSubmit={handleSubmit(submit)}> {/* , , */} <Field name="title" component="input" type="text"/> <Field name="text" component="input" type="text"/> <div> <button type="button" onClick={reset}> </button> <button type="submit"> </button> </div> </form> ); } } Form = reduxForm({ form: 'post', // state (state.form.post) })(Form); export default Form;
コンポーネントレベルが高いハンドラーをスローする必要がある状況を考えてみましょう。
コンポーネントを作成します。
import React, { Component } from 'react'; import Form from './Form' class EditPost extends Component{ constructor(props) { super(props); } handleSubmit = (values) => { console.log(values); }; render() { let {post, dispatch} = this.props; return ( <div> {/* */} <Form onSubmit={this.handleSubmit} /> </div> ); } }
そして、フォームを変更します。
// <form onSubmit={handleSubmit(submit)}> <form onSubmit={handleSubmit}>
値を設定する必要がある場合、初期化中にactionCreator initializeを使用します。これは、フォームの名前を最初のパラメーターとして使用し、2番目のオブジェクトに値を設定します。 たとえば、IDによる記事の場合:
import React, { Component } from 'react'; // import {initialize} from 'redux-form'; import {connect} from 'react-redux'; import Form from './Form' class EditPost extends Component{ constructor(props) { super(props); // post = {title: " ", text: " "} let {post, initializePost} = this.props; // initializePost(post); } handleSubmit = (values) => { console.log(values); }; render() { return ( <div> <Form onSubmit={this.handleSubmit} /> </div> ); } } // props function mapDispatchToProps(dispatch){ return { initializePost: function (post){ dispatch(initialize('post', post)); } } } // props function mapStateToProps(state, ownProps){ const id = ownProps.params.id; return { post: state.posts[id] } } export default connect(mapStateToProps, mapDispatchToProps)(EditPost);
アクションクリエーターの残りはここで見られます 。
標準フィールドに満足できない場合は、独自のレイアウトとアクションを転送できます。
import React, { Component } from 'react'; import { Field, reduxForm } from 'redux-form'; class Form extends Component { // , renderField = ({ input, label, type}) => ( <div> <label>{label}</label> <div> <input {...input} placeholder={label} type={type}/> </div> </div> ); render(){ const {handleSubmit, reset} = this.props; return ( <form onSubmit={handleSubmit}> {/* */} <Field name="title" component={this.renderField} label="" type="text"/> <Field name="text" component={this.renderField} label="" type="text"/> <div> <button type="button" onClick={reset}> </button> <button type="submit"> </button> </div> </form> ); } } Form = reduxForm({ form: 'post' })(Form); export default Form;
Fieldコンポーネントの詳細をご覧ください 。
Redux-formは3種類の検証をサポートしています。
- 同期検証
- 非同期検証
- 検証を送信
同期および非同期検証の場合、formValidate.jsファイルを作成します。
// export const validate = values => { const errors = {}; if(!values.text){ errors.text = ' !'; } else if (values.text.length < 15) { errors.text = ' 15 !' } // return errors }; const sleep = ms => new Promise(resolve => setTimeout(resolve, ms)) // // redux dispatch export const asyncValidate = (values/*, dispatch */) => { return sleep(1000) // .then(() => { if (!values.title) { // throw {title: ' !'} } else if (values.title.length > 10) { throw {title: ' 10 !'} } }) };
送信中に検証するには、promiseを返すように送信ハンドラーを変更する必要があります。
import React, { Component } from 'react'; // import {initialize, SubmissionError} from 'redux-form'; import {connect} from 'react-redux'; import Form from './Form'; const sleep = ms => new Promise(resolve => setTimeout(resolve, ms)); class EditPost extends Component{ constructor(props) { super(props); } handleSubmit = (values) => { /* erros , - , {title: " "}*/ return sleep(1000) {// } .then(({errors, ...data}) => { if (errors) { // // _error throw new SubmissionError({ ...errors, _error: ' !' }) } else { // , data } }) }; render() { return ( <div> {/* */} <Form onSubmit={this.handleSubmit} /> </div> ); } } function mapDispatchToProps(dispatch){ return { initializePost: function (post){ dispatch(initialize('post', post)); } } } function mapStateToProps(state, ownProps){ const id = ownProps.params.id; return { post: state.posts[id] } } export default connect(mapStateToProps, mapDispatchToProps)(EditPost);
検証を接続し、エラー出力を整理します。
import React, { Component } from 'react'; import { Field, reduxForm } from 'redux-form'; import {validate, asyncValidate} from '../formValidate'; class Form extends Component { renderField = ({ input, label, type, meta: { touched, error, warning }}) => ( <div> <label>{label}</label> <div> <input {...input} placeholder={label} type={type}/> {/* */} {touched && ((error && <div>{error}</div>))} </div> </div> ); render(){ const {handleSubmit, reset, error} = this.props; return ( <form onSubmit={handleSubmit}> {/* */} <Field name="title" component={this.renderField} label="" type="text"/> <Field name="text" component={this.renderField} label="" type="text"/> <div> <button type="button" onClick={reset}> </button> <button type="submit"> </button> {/* */} {error && <div>{error}</div>} </div> </form> ); } } Form = reduxForm({ form: 'post', // validate, asyncValidate })(Form); export default Form;
仕事の例を見てみたい人のために、私たちはこれをします:
git clone https://github.com/BoryaMogila/koa_react_redux.git; git checkout redux-form; npm install; npm run-script run-with-build;
そして、リンクlocalhost(127.0.0.1)でredux-formを使用してCRUDアプリケーションを試します:4000 / app /。
非同期検証では、恥ずかしい思いがする可能性があります。サーバーからの応答の前に送信をクリックすると、送信が機能します。
ドキュメンテーションには、もっと面白くて便利なものがあります。 表示することをお勧めします。
PS:いつものように、コンストラクトを待っています。