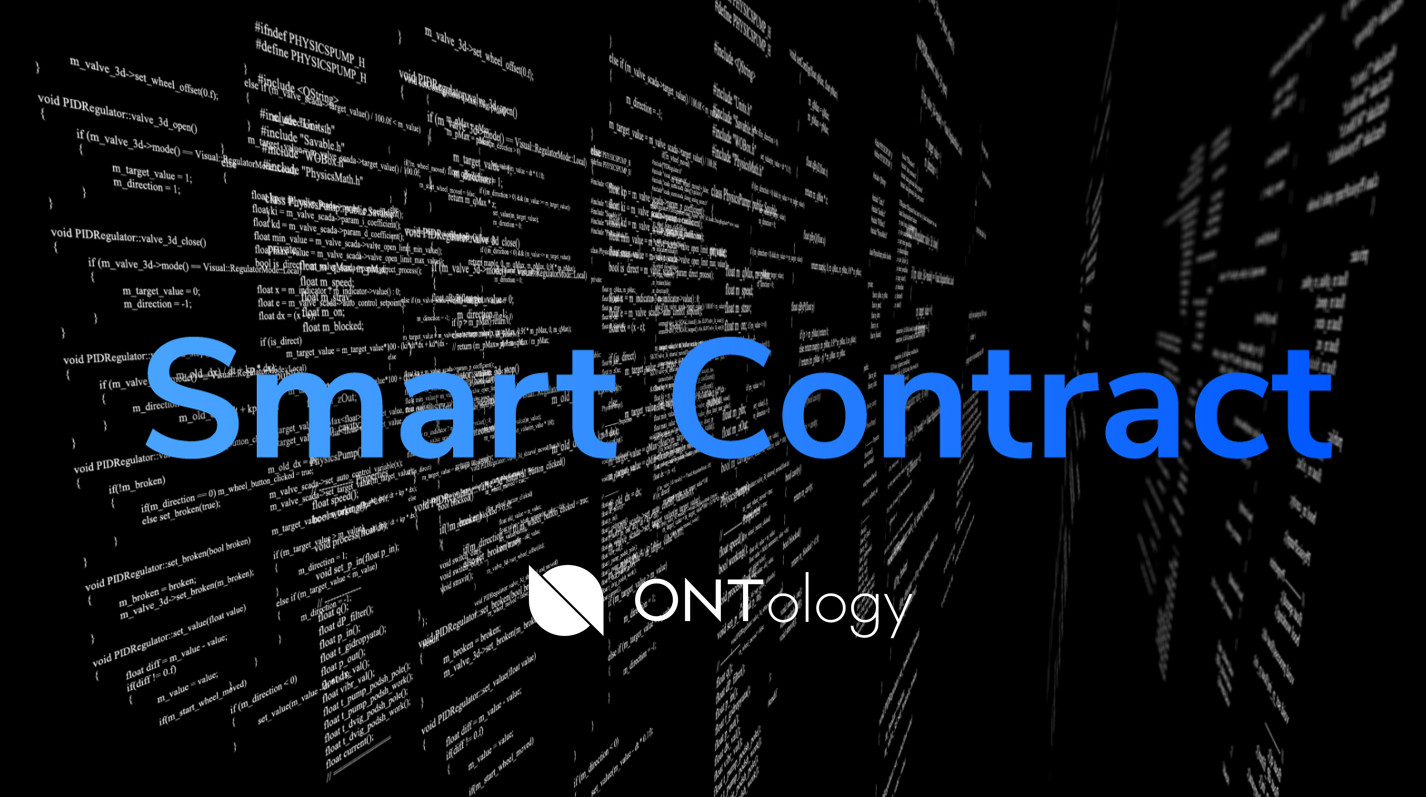
This is the first part of a series of tutorials on creating smart contracts in Python on the Ontology blockchain network using the SmartX smart contract development tool .
In this article, we will begin our introduction to the Ontology Smart Contract API. Ontology Smart Contract API is divided into 7 modules:
- Blockchain & Block API,
- Storage API
- Runtime API
- Native API
- Upgrade API
- Execution Engine API and
- Static & Dynamic Call API.
The Blockchain & Block API is an essential part of the Ontology smart contract system. The Blockchain API supports basic blockchain request operations, such as obtaining the current block height, while the Block API supports basic block request operations, such as requesting the number of transactions for a given block.
Let's get started!
To get started, create a new contract in SmartX , and then follow the instructions below.
1. How to use the Blockchain API
Links to smart contract features are identical to Python links. You can enter the appropriate functions as needed. For example, the following statement introduces GetHeight — a function to get the current block height, and GetHeader — a function to get the block header.
from ontology.interop.System.Blockchain import GetHeight, GetHeader
Getheight
GetHeight is used to get the last block sequence number in the blockchain, as shown in the example below. In the last example, for convenience, we will skip the Main function, but you can add it if necessary.
from ontology.interop.System.Runtime import Notify from ontology.interop.System.Blockchain import GetHeight def Main(operation): if operation == 'demo': return demo() return False def demo(): height=GetHeight() Notify(height) # print height return height #return height after running the function
Getheader
GetHeader, used to get the title of the block, the parameter is the serial number of the block in the blockchain. Example:
from ontology.interop.System.Runtime import Notify from ontology.interop.System.Blockchain import GetHeader def demo(): block_height=10 header=GetHeader(block_height) Notify(header) return header
GetTransactionByHash
GetTransactionByHash is used to obtain a transaction through a hash of transactions. The transaction hash is sent to GetTransactionByHash as parameters in the bytearray format. The key to this function is to convert the hash of the transaction in hex format to the hash of the transaction in bytearray format. This is an important step. Otherwise, you would get an error that indicates that there is no block with such a block hash. Let's take a transaction hash in hex format, as an example, to convert it to bytearray format. An example is as follows:
9f270aa3a4c13c46891ff0e1a2bdb3ea0525669d414994aadf2606734d0c89c1
First reverse the transaction hash:
c1890c4d730626dfaa9449419d662505eab3bda2e1f01f89463cc1a4a30a279
Developers can take this step with the Hex Number (little endian) Number conversion tool provided by SmartX.
Then convert the result to bytearray format:
{0xc1,0x89,0x0c,0x4d,0x73,0x06,0x26,0xdf,0xaa,0x94,0x49,0x41,0x9d,0x66,0x25,0x05,0xea,0xb3,0xbd,0xa2,0xe1,0xf0,0x1f,0x89,0x46,0x3c,0xc1,0xa4,0xa3,0x0a,0x27,0x9f}
This can be done using the String Byte Array conversion tool provided by SmartX. Finally, convert the resulting bytearray to a similar string:
\xc1\x89\x0c\x4d\x73\x06\x26\xdf\xaa\x94\x49\x41\x9d\x66\x25\x05\xea\xb3\xbd\xa2\xe1\xf0\x1f\x89\x46\x3c\xc1\xa4\xa3\x0a\x27\x9f
The following is an example of a GetTransactionByHash function that takes a transaction using a transaction hash:
from ontology.interop.System.Blockchain import GetTransactionByHash def demo(): # tx_hash="9f270aa3a4c13c46891ff0e1a2bdb3ea0525669d414994aadf2606734d0c89c1" tx_hash=bytearray(b"\xc1\x89\x0c\x4d\x73\x06\x26\xdf\xaa\x94\x49\x41\x9d\x66\x25\x05\xea\xb3\xbd\xa2\xe1\xf0\x1f\x89\x46\x3c\xc1\xa4\xa3\x0a\x27\x9f") tx=GetTransactionByHash(tx_hash) return tx
GetTransactionHeight
GetTransactionHeight is used to get the height of a transaction through a transaction hash. Let's take the hash from the example above:
from ontology.interop.System.Blockchain import GetTransactionHeight def demo(): # tx_hash="9f270aa3a4c13c46891ff0e1a2bdb3ea0525669d414994aadf2606734d0c89c1" tx_hash=bytearray(b"\xc1\x89\x0c\x4d\x73\x06\x26\xdf\xaa\x94\x49\x41\x9d\x66\x25\x05\xea\xb3\xbd\xa2\xe1\xf0\x1f\x89\x46\x3c\xc1\xa4\xa3\x0a\x27\x9f") height=GetTransactionHeight(tx_hash) return height
Getcontract
Developers can use the GetContract function to get a contract through a contract hash. The process for converting the hash of the contract is the corresponding process for converting the hash of the transaction that is mentioned above.
from ontology.interop.System.Blockchain import GetContract def demo(): # contract_hash="d81a75a5ff9b95effa91239ff0bb3232219698fa" contract_hash=bytearray(b"\xfa\x98\x96\x21\x32\x32\xbb\xf0\x9f\x23\x91\xfa\xef\x95\x9b\xff\xa5\x75\x1a\xd8") contract=GetContract(contract_hash) return contract
Getblock
GetBlock is used to get a block. There are two ways to get a specific block.
1. Get the block by block height:
from ontology.interop.System.Blockchain import GetBlock def demo(): block=GetBlock(1408) return block
2. Get block by block hash:
from ontology.interop.System.Blockchain import GetBlock def demo(): block_hash=bytearray(b'\x16\xe0\xc5\x40\x82\x79\x77\x30\x44\xea\x66\xc8\xc4\x5d\x17\xf7\x17\x73\x92\x33\x6d\x54\xe3\x48\x46\x0b\xc3\x2f\xe2\x15\x03\xe4') block=GetBlock(block_hash)
2. How to use Block API
There are three functions available in the Block API: GetTransactions , GetTransactionCount , and GetTransactionByIndex . We will sort them one by one.
Gettransactioncount
GetTransactionCount is used to get the number of transactions for a given block.
from ontology.interop.System.Blockchain import GetBlock from ontology.interop.System.Block import GetTransactionCount def demo(): block=GetBlock(1408) count=GetTransactionCount(block) return count
Gettransactions
Developers can use the GetTransactions function to get all transactions in a given block.
from ontology.interop.System.Blockchain import GetBlock from ontology.interop.System.Block import GetTransactions def demo(): block=GetBlock(1408) txs=GetTransactions(block) return txs
GetTransactionByIndex
GetTransactionByIndex is used to get a specific transaction in this block.
from ontology.interop.System.Blockchain import GetBlock from ontology.interop.System.Block import GetTransactionByIndex def demo(): block=GetBlock(1408) tx=GetTransactionByIndex(block,0) # index starts from 0. return tx
The complete guide can be found on our GitHub .
Afterword
The Blockchain & Block API is an indispensable part of smart contracts, because you can use them to request blockchain data and block data in smart contracts. In the following articles, we will discuss how to use the rest of the APIs and find out their interaction with the Ontology blockchain.
The article was translated by Hashrate & Shares specifically for OntologyRussia. cryAre you a developer? Join our tech community on Discord . In addition, take a look at the Developer Center on our website, where you can find developer tools, documentation and much more.