私たちの多くはMicrosoft Visual Studioを使用しており、ほとんどの人がクールなリソースを使用してコードを交換しています。
「だからなぜこのサイトをブラウザにダウンロードし、コードをフォームにコピーしてボタンを押すのに時間を浪費するのか」と考え、スタジオのプラグインを作成して、コードエディターのコンテキストメニューに「PasteBin」アイテムを追加することにしました。 クリックするだけで、ソースコードの選択した部分がリダイレクトされ、その後、PasteBin Webサイトによって作成された一意のURLがクリップボードに表示されます。
仕事ではMicrosoft Visual Studio 2008を使用していますが、2005年にはすべてがまったく同じです。

次に、いくつかの質問が行われ、最終的に、将来のプラグインの空白が表示されます。 プラグインのソースコードには、いくつかの標準機能が含まれている必要があります。 今、私は一貫して、それが何に責任を持ち、どのような順番で呼ばれているのかを説明します。
最初に、標準のConnectクラスのグローバル変数を宣言します 。
// Constants for command properties
private const string CommandName = "PasteBin" ;
private const string CommandCaption = "PasteBin" ;
private const string Url = "http://pastebin.com" ;
private const string RequestParameters = "parent_pid=&format={0}&code2={1}&poster=&paste=Send&expiry=f&email=" ;
// Constants for file formats
private static readonly Dictionary< string , string > FileFormat = new Dictionary< string , string >
{
{ "c" , "c" },
{ "h" , "c" },
{ "cpp" , "cpp" },
{ "cs" , "csharp" }
};
// Variables for IDE and add-in instances
private DTE _applicationObject;
private AddIn _addInInstance;
// Buttons that will be created on built-in command bars of Visual Studio
// We must keep them at class level to remove them when the add-in is unloaded
private CommandBarButton _pasteBinButton;
* This source code was highlighted with Source Code Highlighter .
最初の標準OnConnection関数は、ユーザーインターフェイスでの変更を実装するためにStudioの読み込みのどの段階で必要かを説明します。 この記事には興味がありません。希望する人は、記事の最後に添付される完全なソースコードでそれを見ることができます。 さらに興味深いのは、コンテキストメニューに新しいアイテムを追加するプロセスを直接説明するAddUI関数です(ちなみに、 AddUIは、2番目の標準関数であるOnStartupCompleteによっても呼び出されます。
したがって、 AddUI関数。 最初に、私たちと同じ名前の(決して知らない)チームが既に作成されているかどうかを確認し、作成されていない場合は自分で作成します。
// Try to retrieve the command, just in case it was already created, ignoring the
// exception that would happen if the command was not created yet.
try
{
myCommand = _applicationObject.Commands.Item(_addInInstance.ProgID + "." + CommandName, -1);
}
catch (Exception e)
{
Debug.Write(e.ToString());
}
// Add the command if it does not exist
if (myCommand == null )
{
myCommand = _applicationObject.Commands.AddNamedCommand(_addInInstance,
CommandName, CommandCaption, null , true , 59, ref contextUIGuids,
( int )(vsCommandStatus.vsCommandStatusSupported | vsCommandStatus.vsCommandStatusEnabled));
}
* This source code was highlighted with Source Code Highlighter .
次に、コードエディターのコンテキストメニューへの「ポインター」を取得します。
// Constants for names of built-in command bars of Visual Studio
const string vsCodeWindowCommandbarName = "Code Window" ;
// Retrieve the collection of command bars
// Note:
// - In VS.NET 2002/2003 (which uses the Office.dll reference)
// DTE.CommandBars returns directly a CommandBars type, so a cast
// to CommandBars is redundant
// - In VS 2005 or higher (which uses the new Microsoft.VisualStudio.CommandBars.dll reference)
// DTE.CommandBars returns an Object type, so we do need a cast to CommandBars
var commandBars = (CommandBars)_applicationObject.CommandBars;
// Retrieve some built-in command bars
codeCommandBar = commandBars[vsCodeWindowCommandbarName];
* This source code was highlighted with Source Code Highlighter .
そして、その外観を忘れずに、コンテキストメニューでアイテムを説明します-アイコン。
// Create the buttons from the commands
// Note:
// - In VS.NET 2002/2003 (which uses the Office.dll reference)
// Command.AddControl returns directly a CommandBarControl type, so a cast
// to CommandBarControl is redundant
// - In VS 2005 or higher (which uses the new Microsoft.VisualStudio.CommandBars.dll reference)
// Command.AddControl returns an Object type, so we do need a cast to CommandBarControl
string ns = GetType().Namespace;
Assembly currentAssembly = GetType(). Assembly ;
Stream
imgStreamPic = currentAssembly.GetManifestResourceStream(ns + "." + "klipper.png" ),
imgStreamMask = currentAssembly.GetManifestResourceStream(ns + "." + "klipper-mask.png" );
if (imgStreamPic != null && imgStreamMask != null )
{
Image
pasteBinImage = Image.FromStream(imgStreamPic),
pasteBinImageMask = Image.FromStream(imgStreamMask);
// ------------------------------------------------------------------------------------
// Button on the "Code Window" context menu
// ------------------------------------------------------------------------------------
// Add a button to the built-in "Code Window" context menu
_pasteBinButton = (CommandBarButton) myCommand.AddControl(codeCommandBar,
codeCommandBar.Controls.Count + 1);
// Change some button properties
_pasteBinButton.Caption = CommandCaption;
_pasteBinButton.BeginGroup = true ; // Separator line above button
_pasteBinButton.Style = MsoButtonStyle.msoButtonIconAndCaption; // It could be also msoButtonIcon
_pasteBinButton.Picture = (stdole.StdPicture) ImageConverter.ImageToIpicture(pasteBinImage);
_pasteBinButton.Mask = (stdole.StdPicture) ImageConverter.ImageToIpicture(pasteBinImageMask);
}
* This source code was highlighted with Source Code Highlighter .
GetManifestResourceStreamを使用してリソースを取得するには、プロジェクトに必要なファイルを追加し、プロパティでBuild ActionパラメーターをEmbeded Resourceに変更する必要があります。
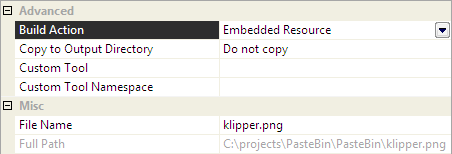
以下は、3つのまったく関係のない関数、 OnDisconnection 、 OnBeginShutdown、およびOnAddInsUpdateです。 しかし、それらの直後に、プロジェクトの中心であるExec関数があります。
コードフラグメントが選択されている場合、ソースコードとUrlEncodeを使用して変換された選択されたテキストを含むアクティブファイルのタイプに基づいて、サイトへのリクエストが生成され、配置されたソースコードへの一意のリンクがサーバーの応答から抽出され、クリップボードに配置されます
public void Exec( string cmdName, vsCommandExecOption executeOption, ref object varIn,
ref object varOut, ref bool handled)
{
handled = false ;
if (executeOption == vsCommandExecOption.vsCommandExecOptionDoDefault)
{
if (cmdName == string .Format( "{0}.{1}" , _addInInstance.ProgID, CommandName))
{
handled = true ;
if (!((TextSelection)_applicationObject.ActiveDocument.Selection).IsEmpty)
{
// parameters: name1=value1&name2=value2
var webRequest = (HttpWebRequest)WebRequest.Create(Url);
webRequest.ContentType = "application/x-www-form-urlencoded" ;
webRequest.Method = "POST" ;
// formatting request string from document type and selection
string request = string .Format(RequestParameters,
FileFormat[_applicationObject.ActiveDocument.Name.Split( '.' )[1]],
System.Web.HttpUtility.UrlEncode(((TextSelection)_applicationObject.ActiveDocument.Selection).Text));
Stream os = null ;
try
{ // send the POST
webRequest.ContentLength = request.Length; //Length of request to send
os = webRequest.GetRequestStream();
os.Write(System.Text. Encoding .GetEncoding( "windows-1251" ).GetBytes(request), 0, request.Length); //Send it
}
catch (WebException ex)
{
MessageBox.Show(ex.Message, "HttpPost: Request error" ,
MessageBoxButtons.OK, MessageBoxIcon.Error);
}
finally
{
if (os != null )
{
os.Close();
}
}
try
{ // get the response
var webResponse = (HttpWebResponse)webRequest.GetResponse();
if (webResponse != null )
{ // copy to clipboard
Clipboard.SetText(webResponse.ResponseUri.ToString());
MessageBox.Show( "Upload successfully. Url is copied in your clipboard." );
}
}
catch (WebException ex)
{
MessageBox.Show(ex.Message, "HttpPost: Response error" ,
MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
}
}
* This source code was highlighted with Source Code Highlighter .
このファイルは、目立たない標準のQueryStatus関数も完了します。
付録A
1. メインファイルConnect.csの内容
2. ImageConverter.cs補助ファイルの内容
上記のソースは、記事で説明されているプラグインを使用してサイトに追加されました。
付録B
記事のファイル
PasteBin.AddInおよびPasteBin.dllファイルは、%USERPROFILE%\ My Documents \ Visual Studio 2008 \ Addinsディレクトリに配置する必要があります。 2008は2005に置き換えることができます。