素晴らしい本Learning Three.js- The JavaScript 3D Library for WebGLの無料翻訳を始めたいと思います。 この本は初心者だけでなく、その分野の専門家にとっても興味深いものになると確信しています。 まあ、私は長い間導入部を引きずりません、私たちがすぐにできることの例を挙げます:
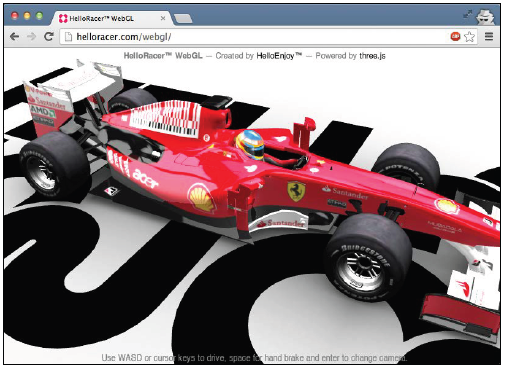
HTMLページ構造を作成する
最初に行う必要があるのは、すべての例の基礎として使用できる空のHTMLページを作成することです。 このHTML構造は次のように表されます。
<!DOCTYPE html> <html> <head> <title>Example 01.01 - Basic skeleton</title> <script type="text/javascript" src="../libs/three.js"> </script> <script type="text/javascript" src="../libs/jquery-1.9.0.js"> </script> <style> body{ /* set margin to 0 and overflow to hidden, to use the complete page */ margin: 0; overflow: hidden; } </style> </head> <body> <!--Div which will hold the Output --> <div id="WebGL-output"> </div> <!--Javascript code that runs our Three.js examples --> <script type="text/javascript"> // once everything is loaded, we run our Three.js stuff. $(function () { // here we'll put the Three.js stuff }); </script> </body> </html>
このリストは、非常に単純なHTMLページの構造を示しており、いくつかの要素しかありません。 ブロックでは、例で使用する外部JavaScriptライブラリをロードします。 たとえば、上記のリストでは、
Three.js
と
jquery-1.9.0.js
2つのライブラリを接続しようとしています。 また、このブロックでは、CSS設計のための数行を規定しています。 前のスニペットでは、JavaScriptコードを確認できます。 この小さなコードでは、ページが完全に読み込まれたときに
JQuery
を使用して名前のないJavaScript関数を呼び出します。 すべてのThree.jsコードをこの関数内に配置します。
Three.jsには2つのバージョンがあります。
- Three.min.js:通常、Three.jsサイトを開くときにこのライブラリを使用します。 これは、通常のThree.jsライブラリの半分のサイズであるUglifyJSを使用して作成されたThree.jsの最小化バージョンです。 ここで使用されるすべての例とコードは、2013年8月にリリースされた
Three.js r60
プロジェクトに基づいています。 - Three.js:これは通常のThree.jsライブラリです。 コードが読みやすくなるため、デバッグがはるかに簡単になるため、このライブラリを例で使用します。
ブラウザで作成したばかりのページを開いても、それほどショックを受けることはありません。 予想どおり、表示されるのは空白のページのみです。
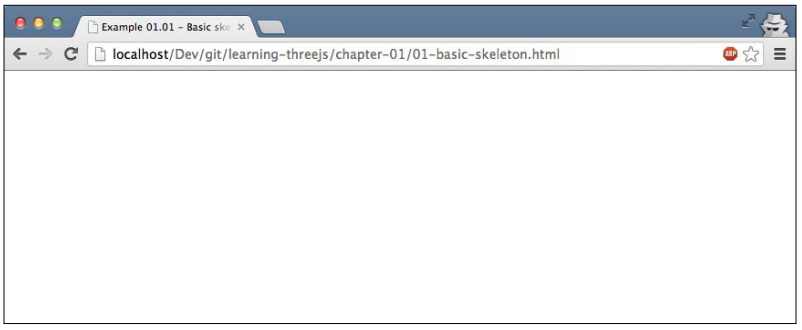
次のセクションでは、3Dオブジェクトの最初のペアをシーンに追加する方法を学習します。
3Dオブジェクトのレンダリングと表示
この時点で、最初のシーンを作成し、いくつかのオブジェクトとカメラを追加します。 最初の例には、次のオブジェクトが含まれます。
プレーンは、メインサイトとして機能する2次元の長方形です。 シーンの中央に灰色の長方形として表示されます。
キューブは、赤でレンダリングする3次元のキューブです。
球体は3次元の球体で、青でレンダリングします。
カメラ-出力に表示されるものを決定します。
軸はx、y、zです。 これは、オブジェクトがレンダリングされる場所を確認するのに便利なデバッグツールです。
まず、コードでどのように見えるかを見てから、それを理解してみましょう。
<script type="text/javascript"> $(function () { var scene = new THREE.Scene(); var camera = new THREE.PerspectiveCamera(45 , window.innerWidth / window.innerHeight , 0.1, 1000); var renderer = new THREE.WebGLRenderer(); renderer.setClearColorHex(0xEEEEEE); renderer.setSize(window.innerWidth, window.innerHeight); var axes = new THREE.AxisHelper( 20 ); scene.add(axes); var planeGeometry = new THREE.PlaneGeometry(60,20,1,1); var planeMaterial = new THREE.MeshBasicMaterial({color: 0xcccccc}); var plane = new THREE.Mesh(planeGeometry,planeMaterial); plane.rotation.x=-0.5*Math.PI; plane.position.x = 15; plane.position.y = 0; plane.position.z = 0; scene.add(plane); var cubeGeometry = new THREE.CubeGeometry(4,4,4); var cubeMaterial = new THREE.MeshBasicMaterial( {color: 0xff0000, wireframe: true}); var cube = new THREE.Mesh(cubeGeometry, cubeMaterial); cube.position.x = -4; cube.position.y = 3; cube.position.z = 0; scene.add(cube); var sphereGeometry = new THREE.SphereGeometry(4,20,20); var sphereMaterial = new THREE.MeshBasicMaterial( {color: 0x7777ff, wireframe: true}); var sphere = new THREE.Mesh(sphereGeometry,sphereMaterial); sphere.position.x = 20; sphere.position.y = 4; sphere.position.z = 2; scene.add(sphere); camera.position.x = -30; camera.position.y = 40; camera.position.z = 30; camera.lookAt(scene.position); $("#WebGL-output").append(renderer.domElement); renderer.render(scene, camera); });
この例をブラウザで開くと、私たちが望んでいたものに似たものが表示されますが、それでも理想からはほど遠いでしょう。
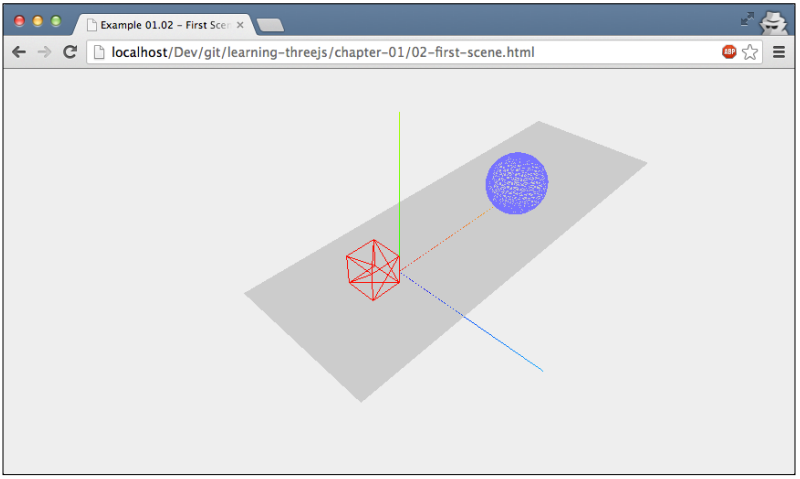
シーンをきれいにする前に、私たちがやったことをステップバイステップで見ていきましょう。
var scene = new THREE.Scene(); var camera = new THREE.PerspectiveCamera(45 , window.innerWidth / window.innerHeight , 0.1, 1000); var renderer = new THREE.WebGLRenderer(); renderer.setClearColorHex(0xEEEEEE); renderer.setSize(window.innerWidth, window.innerHeight);
この例では、シーン、カメラ、およびビジュアライザーを定義しました。 シーン変数は、表示するすべてのオブジェクトを保存および追跡するために使用されるコンテナです。 この例では、表示したい球体と立方体が少し後で追加されます。 このスニペットでは、カメラ変数も作成しました。 この変数は、シーンをレンダリングするときに表示されるものを決定します。 次に、可視化(レンダリング)のオブジェクトを定義します。 レンダリングは、ブラウザ内のシーンがどのように見えるかを計算する役割を果たします。 また、ビデオカードを使用してシーンをレンダリングする
WebGLRenderer
オブジェクトも作成しました。
ここでは、
setClearColorHex()
関数を使用して、レンダラーの背景色をほぼ白(0XEEEEEE)に設定し、
setSize()
関数を使用してレンダリングされたシーンのサイズも設定します。
これまでのところ、空のシーン、
render
、
camera
などの基本的な要素しかありませんでした。 ただし、視覚化するものはまだありません。 次のコードは、補助軸と平面を追加します。
var axes = new THREE.AxisHelper( 20 ); scene.add(axes); var planeGeometry = new THREE.PlaneGeometry(60,20); var planeMaterial = new THREE.MeshBasicMaterial( {color: 0xcccccc}); var plane = new THREE.Mesh(planeGeometry,planeMaterial); plane.rotation.x = -0.5*Math.PI; plane.position.x = 15; plane.position.y = 0; plane.position.z = 0; scene.add(plane);
Axesオブジェクトを作成し、
scene.add()
関数を使用してAxesをシーンに追加したことがわかります。 これで平面を作成できます。 これは2つのステップで行われます。 最初に、
new THREE
を使用して平面が表示されることを決定します。 コードには
PlaneGeometry(60,20)
ます。 この場合、プレーンの幅は60、高さは20です。また、Three.jsにプレーンの外観(色や透明度など)を伝える必要があります。 Three.jsでは、マテリアルオブジェクトを作成してこれを行います。 最初の例では、色0xccccccのメインマテリアルを作成します(
MeshBasicMaterial()
メソッドを使用)。 次に、これら2つのオブジェクトを
plane
という名前の1つのMeshオブジェクトに結合します。
plane
をシーンに追加する前に、正しい位置に配置する必要があります。 これを行うには、まずx軸を中心に90度回転してから、
position
プロパティを使用してシーン上の位置を決定します。 最後に、
axes
オブジェクトで行ったように、このオブジェクトをシーンに追加する必要があります。
立方体と球体は同じ方法で追加されますが、
wireframe
プロパティの値は
true
になるため、この例の最後の部分に進みましょう。
camera.position.x = -30; camera.position.y = 40; camera.position.z = 30; camera.lookAt(scene.position); $("#WebGL-output").append(renderer.domElement); renderer.render(scene, camera);
この段階で、視覚化するすべての要素が適切な場所でシーンに追加されました。 カメラが表示するものを決定することは既に述べました。 このコードでは、
position
属性のx、y、zの値を使用してカメラを配置し、シーン上でホバリングを開始します。 カメラがオブジェクトを見ていることを確認するには、
lookAt()
関数を使用してシーンの中心を指すようにします。
マテリアル、ライティング、シャドウを追加する
Three.jsに新しいマテリアルと照明を追加するのは非常に簡単で、前のセクションで行ったのとほぼ同じです。
var spotLight = new THREE.SpotLight( 0xffffff ); spotLight.position.set( -40, 60, -10 ); scene.add(spotLight );
SpotLight()
メソッドは、
spotLight.position.set( -40, 60, -10 )
SpotLight()
spotLight.position.set( -40, 60, -10 )
位置からシーンを照らします。シーンを再度レンダリングすると、前の例と違いがないことが
SpotLight()
ます。 その理由は、異なる材料が光に対して異なる反応をするためです。 前の例でオブジェクトに使用した主なマテリアル(
MeshBasicMaterial
()メソッドを使用)は、シーン内の光源にはまったく反応しません。 オブジェクトを特定の色で視覚化しただけです。 したがって、以下に示すように、平面、球、立方体の材料を変更する必要があります。
var planeGeometry = new THREE.PlaneGeometry(60,20); var planeMaterial = new THREE.MeshLambertMaterial( {color: 0xffffff}); var plane = new THREE.Mesh(planeGeometry,planeMaterial); ... var cubeGeometry = new THREE.CubeGeometry(4,4,4); var cubeMaterial = new THREE.MeshLambertMaterial( {color: 0xff0000}); var cube = new THREE.Mesh(cubeGeometry, cubeMaterial); ... var sphereGeometry = new THREE.SphereGeometry(4,20,20); var sphereMaterial = new THREE.MeshLambertMaterial( {color: 0x7777ff}); var sphere = new THREE.Mesh(sphereGeometry,sphereMaterial);
コードのこのセクションでは、MeshLambertMaterial関数を使用してオブジェクトのマテリアルプロパティを変更しました。 Three.jsは、光源が認識する2つのマテリアル、MeshLambertMaterialとMeshPhongMaterialを提供します。
ただし、次のスクリーンショットに示すように、これはまだ私たちが目指しているものではありません。
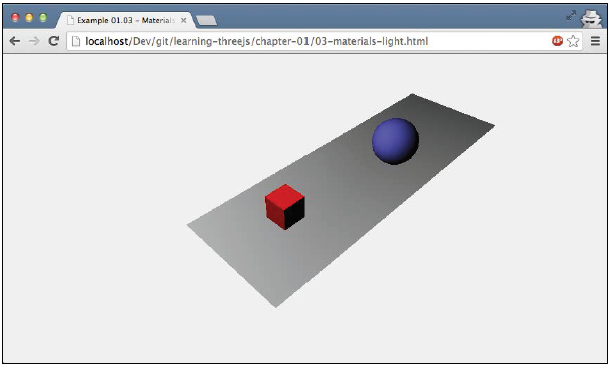
立方体と球体は少し良くなりましたが、今のところ影はありません。 シャドウのレンダリングには多くの処理能力が必要になるため、Three.jsではデフォルトでシャドウが無効になっています。 しかし、それらの組み込みは実際には非常に簡単です。 シャドウの場合、次のコードフラグメントに示すように、いくつかの場所でソースを変更する必要があります。
renderer.setClearColorHex(0xEEEEEE, 1.0); renderer.setSize(window.innerWidth, window.innerHeight); renderer.shadowMapEnabled = true;
最初に行う必要があるのは、シャドウを含めることを
render
伝えることです。 これを行うには、
shadowMapEnabled
プロパティを
true
設定し
true
。 このアクションの結果を見ると、何もわかりません。 これは、どのオブジェクトが影を落とし、どのオブジェクトが当てはまるかを明示的に設定する必要があるためです。 この例では、球と立方体が平面に影を落とすようにします。 これを行うには、適切なプロパティをtrueに設定します。
plane.receiveShadow = true; ... cube.castShadow = true; ... sphere.castShadow = true;
次に、影を表示するために必要なことがもう1つあります。 どの光源が影を引き起こすかを判断する必要があります。 これらのすべてがこれを行うことはできません。これについては次のパートで説明します。この例では、
SpotLight()
メソッドを使用します。 正しいプロパティを設定し、最後に影を視覚化するだけです。
spotLight.castShadow = true;
何が起こったのかは、次のスクリーンショットで確認できます。
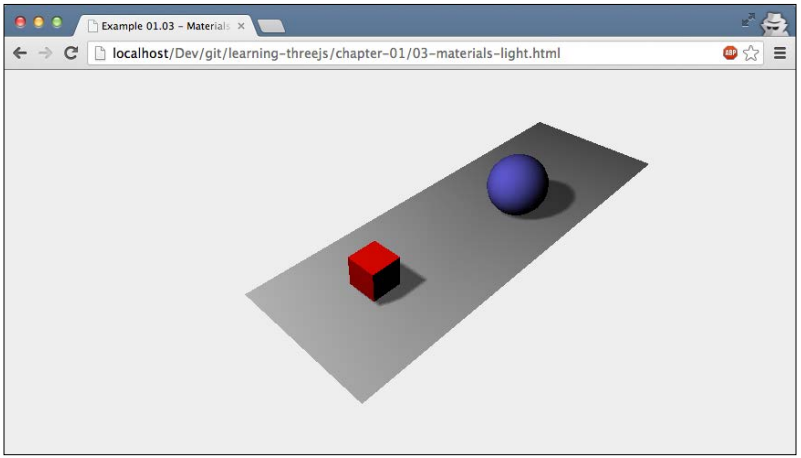
次のセクションでは、簡単なアニメーションを追加します。
アニメーションでシーンを複雑にしましょう
何らかの方法でシーンを復活させたい場合、最初にすべきことは、特定の間隔でシーンを再描画する方法を見つけることです。 これを行うには、
setInterval(function,interval)
を使用し
setInterval(function,interval)
。 この関数を使用して、100ミリ秒ごとに呼び出される関数を定義できます。 この関数の問題は、ブラウザで何が起こっているかを考慮していないことです。 別のタブを表示している場合、この関数は数ミリ秒ごとに呼び出されます。 また、
setInterval()
メソッドは画面の再描画と同期しません。 これはすべて、プロセッサの高負荷と低効率につながる可能性があります。
requestAnimationFrame()メソッドの紹介
幸いなことに、最新のブラウザには
setInterval()
関数に関連する問題の解決策があります:
requestAnimationFrame
()メソッド。 この関数を使用すると、ブラウザで定義された間隔で呼び出される関数を指定できます。 以下に示すように、レンダリングを処理する関数を作成するだけです。
function renderScene() { requestAnimationFrame(renderScene); renderer.render(scene, camera); }
renderScene
()関数で、
requestAnimationFrame
()メソッドを再度呼び出して、進行中のアニメーションを保存します。 唯一必要なのは、シーンを完全に作成した後に
renderer.render()
メソッド呼び出しの場所を変更することです
renderScene
()関数を
renderScene
呼び出してアニメーションを開始します。
... $("#WebGL-output").append(renderer.domElement); renderScene();
このコードを実行すると、まだ何もアニメーション化していないため、前の例と比較して変更は表示されません。 アニメーションを追加する前に、アニメーションが機能するフレームレートに関する情報を提供する小さなヘルパーライブラリを紹介します。 接続する必要があるのは、要素に登録することだけです
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
-
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, –, :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
.setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() .render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? –rotation
0,02render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
;position
. , , . .Math.cos()
Math.sin()
step
. . ,step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
:this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – ,render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
-
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, –, :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
.setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() .render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? –rotation
0,02render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
;position
. , , . .Math.cos()
Math.sin()
step
. . ,step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
:this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – ,render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, – , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); … }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? – rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
– JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , – , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub