Model-View-Presenterは、よく知られたデザインパターンです。 一見、すべてがシンプルに見えます。画面のすべてのビジネスロジックを含むモデルがあります。 特定のデータを表示する方法を知っているビュー/ビュー。 リンクである代表(プレゼンター)-ビューでのユーザーアクションに応答し、モデルを変更します。逆も同様です。
プロジェクトのフォームの数が複数になると、複雑さが始まります。
この記事では以下について説明します。
-少しの理論。
-WindowsフォームでのMVP(つまりパッシブビュー)の実装に関する一般的な問題。
-フォームとパラメーター転送、モーダルウィンドウ間の遷移の実装の機能。
-IoCコンテナと依存性注入の使用-DIテンプレート(コンストラクター注入)。
-MVPアプリケーションのテストの一部の機能(NUnitおよびNSubstituteを使用);
-これらはすべてミニプロジェクトの例で発生し、視覚的になろうとします。
記事の内容は次のとおりです。
-アダプタテンプレートの適用。
-Application Controllerテンプレートの単純な実装。
この記事の対象者
主に、聞いたことがあるが試したことがない、または試したが失敗したWindowsフォームの初心者向けです。 いくつかのトリックはWPFに、そしてWeb開発にも適用できると確信していますが。
問題の声明
3つの画面を実装する簡単なタスクを考え出します。
1)認証画面;
2)メイン画面。
3)ユーザー名を変更するためのモーダル画面。
次のようなものが得られるはずです。
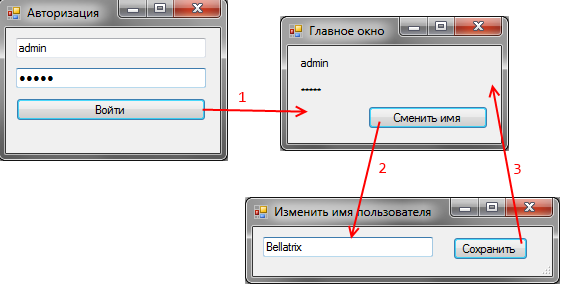
理論のビット
MVPは、その親であるMVC(Model-View-Controller)と同様に、ビジネスロジックを表示方法から分離する利便性のために考案されました。

インターネットでは、MVPの多くの実装を見つけることができます。 プレゼンテーションへのデータ配信の方法により、それらは3つのカテゴリに分類できます。
-パッシブビュー:ビューには、プリミティブデータ(文字列、数字)を表示するための最小限のロジックが含まれ、残りはプレゼンターによって行われます。
-プレゼンテーションモデル:ビューでは、プリミティブデータだけでなくビジネスオブジェクトも転送できます。
-監督コントローラー:Viewはモデルの可用性を認識し、モデルからデータを取得します。
次に、パッシブビューの変更が検討されます。 主な機能について説明します。
-データを表示するためのコントラクトを提供するプレゼンテーションインターフェイス(IView)。
-プレゼンテーションはIViewの特定の実装であり、特定のインターフェイス(Windows Forms、WPF、さらにはコンソール)で表示でき、それを制御するユーザーについては何も知りません。 私たちの場合、これらはフォームです。
-モデル-いくつかのビジネスロジックを提供します(例:データベース、リポジトリ、サービスへのアクセス)。 クラスとして、または再びインターフェイスと実装として表すことができます。
-担当者は、インターフェース(IView)を介してビューへのリンクを含み、それを管理し、イベントにサブスクライブし、入力されたデータの簡単な検証(検証)を実行します。 また、モデルまたはそのインターフェイスへのリンクが含まれており、Viewからデータを転送して更新を要求します。
典型的な代表的な実装
public class Presenter { private readonly IView _view; private readonly IService _service; public Presenter(IView view, IService service) { _view = view; _service = service; _view.UserIdChanged += () => UpdateUserInfo(); } private void UpdateUserInfo() { var user = _service.GetUser(_view.UserId); _view.Username = user.Username; _view.Age = user.Age; } }
小さなクラスの接続を使用することの利点は何ですか(インターフェイス、イベントを使用)?
1.残りを壊すことなく、コンポーネントのロジックを比較的自由に変更できます。
2.単体テストの大きな機会。 TDDファンは興奮するはずです。
さあ始めましょう!
プロジェクトを整理する方法は?
ソリューションが4つのプロジェクトで構成されることに同意します。
-DomainModel-サービスとすべての種類のリポジトリ、つまりモデルが含まれます。
-プレゼンテーション-視覚的なプレゼンテーションに依存しないアプリケーションロジックが含まれています。 すべての代表者、プレゼンテーションインターフェイス、およびその他の基本クラス。
-UI-Windows Formsアプリケーション。フォーム(プレゼンテーションインターフェイスの実装)とスタートアップロジックのみが含まれます。
-テスト-単体テスト。
Main()に何を書くか?
Windowsフォームアプリケーションを起動する標準的な実装は次のようになります。
private static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); Application.Run(new MainForm()); // () }
しかし、代表者がビューを管理することに同意したため、コードは次のようになります。
private static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); var presenter = new LoginPresenter(new LoginForm(), new LoginService()); // Dependency Injection presenter.Run(); }
最初の画面を実装してみましょう。
基本的なインターフェース
// public interface IView { void Show(); void Close(); } // , public interface ILoginView : IView { string Username { get; } string Password { get; } event Action Login; // " " void ShowError(string errorMessage); } public interface IPresenter { void Run(); } // public interface ILoginService { bool Login(User user); // true - , false }
提出
public class LoginPresenter : IPresenter { private readonly ILoginView _view; private readonly ILoginService _service; public LoginPresenter(ILoginView view, ILoginService service) { _view = view; _service = service; _view.Login += () => Login(_view.Username, _view.Password); } public void Run() { _view.Show(); } private void Login(string username, string password) { if (username == null) throw new ArgumentNullException("username"); if (password == null) throw new ArgumentNullException("password"); var user = new User {Name = username, Password = password}; if (!_service.Login(user)) { _view.ShowError("Invalid username or password"); } else { // , (?) } } }
ILoginServiceの実装を記述するのと同様に、フォームを作成してILoginViewインターフェイスを実装することは難しくありません。 1つの機能のみに注意する必要があります。
public partial class LoginForm : Form, ILoginView { // ... public new void Show() { Application.Run(this); } }
この呪文により、アプリケーションを起動してフォームを表示し、フォームを閉じるとアプリケーションを正しく終了できます。 しかし、これに戻ります。
テストはありますか?
担当者(LoginPresenter)を書いた瞬間から、フォームまたはサービスを実装せずに、ユニットテストからすぐにテストすることが可能になります。
テストを書くために、私はNUnitおよびNSubstituteライブラリ(インターフェース、モックによってスタブクラスを作成するためのライブラリ)を使用しました。
LoginPresenterのテスト
[TestFixture] public class LoginPresenterTests { private ILoginView _view; [SetUp] public void SetUp() { _view = Substitute.For<ILoginView>(); // var service = Substitute.For<ILoginService>(); // service.Login(Arg.Any<User>()) // admin/password .Returns(info => info.Arg<User>().Name == "admin" && info.Arg<User>().Password == "password"); var presenter = new LoginPresenter(_view, service); presenter.Run(); } [Test] public void InvalidUser() { _view.Username.Returns("Vladimir"); _view.Password.Returns("VladimirPass"); _view.Login += Raise.Event<Action>(); _view.Received().ShowError(Arg.Any<string>()); // } [Test] public void ValidUser() { _view.Username.Returns("admin"); _view.Password.Returns("password"); _view.Login += Raise.Event<Action>(); _view.DidNotReceive().ShowError(Arg.Any<string>()); // } }
アプリケーション自体もそうですが、テストはかなり馬鹿げています。 しかし、とにかく、それらは正常に完了しました。
誰がどのようにパラメーターを使用して2番目の画面を起動しますか
お気づきかもしれませんが、承認が成功したことを示すコードは書きませんでした。 2番目の画面を起動するにはどうすればよいですか? 頭に浮かぶ最初のものは次のとおりです。
// LoginPresenter: var mainPresenter = new MainPresenter(new MainForm()); mainPresenter.Run(user);
しかし、代表者は、そのインターフェース以外の表現について何も知らないことに同意しました。 どうする?
アプリケーションコントローラーパターン(単純化された方法で実装されています)が助けになります。内部には、インターフェイスを介して実装オブジェクトを取得する方法を知っているIoCコンテナーが含まれています。
コントローラーはコンストラクターパラメーター(各DI)によって各代表に渡され、およそ次のメソッドを実装します。
public interface IApplicationController { IApplicationController RegisterView<TView, TImplementation>() where TImplementation : class, TView where TView : IView; IApplicationController RegisterService<TService, TImplementation>() where TImplementation : class, TService; void Run<TPresenter>() where TPresenter : class, IPresenter; }
少しリファクタリングした後、アプリケーションの起動は次のようになり始めました。
private static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); // : var controller = new ApplicationController(new LightInjectAdapder()) .RegisterView<ILoginView, LoginForm>() .RegisterService<ILoginService, StupidLoginService>() .RegisterView<IMainView, MainForm>(); controller.Run<LoginPresenter>(); }
new ApplicationController(new LightInjectAdapder())
に関するいくつかの言葉。 IoCコンテナーとして、LightInjectライブラリを使用しましたが、直接ではなく、アダプター(アダプターパターン)を使用したため、コンテナーを別のアダプターに変更する必要がある場合は、コントローラーロジックを変更せずに別のアダプターを作成できます。 使用されるすべてのメソッドは、ほとんどのIoCライブラリにあり、問題は発生しません。
追加のインターフェースを実装します
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,
Application.Run(Form)
Windows . ,
ExitThread
Form.Closed
, .
, - :
Application.Run(ApplicationContext)
,
ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "
Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,
Application.Run(Form)
Windows . ,
ExitThread
Form.Closed
, .
, - :
Application.Run(ApplicationContext)
,
ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "
Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,
Application.Run(Form)
Windows . ,
ExitThread
Form.Closed
, .
, - :
Application.Run(ApplicationContext)
,
ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "
Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
-
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,Application.Run(Form)
Windows . ,ExitThread
Form.Closed
, .
, - :Application.Run(ApplicationContext)
,ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
-
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,Application.Run(Form)
Windows . ,ExitThread
Form.Closed
, .
, - :Application.Run(ApplicationContext)
,ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
-
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,Application.Run(Form)
Windows . ,ExitThread
Form.Closed
, .
, - :Application.Run(ApplicationContext)
,ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
-
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,Application.Run(Form)
Windows . ,ExitThread
Form.Closed
, .
, - :Application.Run(ApplicationContext)
,ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
-
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,Application.Run(Form)
Windows . ,ExitThread
Form.Closed
, .
, - :Application.Run(ApplicationContext)
,ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,
Application.Run(Form)
Windows . ,
ExitThread
Form.Closed
, .
, - :
Application.Run(ApplicationContext)
,
ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "
Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . ,
Application.Run(Form)
Windows . ,
ExitThread
Form.Closed
, .
, - :
Application.Run(ApplicationContext)
,
ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " "
Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter
IPresenter, , Run . .
, , , :
Controller.Run<MainPresener, User>(user); View.Close();
...
View.Close()
, , . , Application.Run(Form)
Windows . , ExitThread
Form.Closed
, .
, - : Application.Run(ApplicationContext)
, ApplicationContext.MainForm
. , (instance) ApplicationContext ( DI) . :
// LoginForm public new void Show() { _context.MainForm = this; Application.Run(_context); } // MainForm public new void Show() { _context.MainForm = this; base.Show(); }
. " " Controller.Run<ChangeUsernamePresenter, User>(user)
. - , ApplicationContext:
public new void Show() { ShowDialog(); }
, , Form.
... ?
, , :
, ( ). , , - . [] , , . [] . .
IoC- IContainer -.
c Github ( Nuget-).
, , - MVP, , , .
. , , !
, :
- ( );
- ( Event Aggregator);
-
,
, MVP
Passive View
Application Controller
Application Controller Event Aggregator
Application Controller
MVP ,
Presenter