
最後のトピックで、 backbone.jsフレームワークを使用する基本的な原則を説明しましたが、今度は実践して何か役に立つことをすることを提案します。
挑戦する
私たちのサイトがしばしば異なる種類のポップアップを使用すると仮定します。 それらはすべて同様の機能を備えており、大量に開いたり、ドラッグアンドドロップしたりできます。 さらに、アクティブなポップアップと非アクティブなポップアップは区別され、アクティブなポップアップは残りの上部にあり、影付きではありません(うーん...これはウィンドウマネージャーのようなものです)。
Vobschemは次のようなものです。
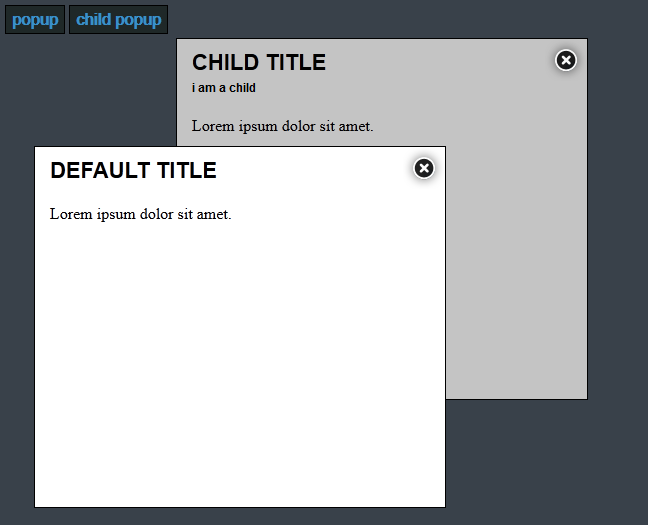
OOPの規約に従って、すべての人に共通の動作をするポップアップを継承し、好みに合わせて作成できるポップアップ用のクラスを開発しようとします。
Backbone.js 、 jquery 、 jquery ui 、 underscore.jsをダウンロードし、
index.html
まず、接続されたライブラリ、(まだ作成されていない)アプリケーションのファイル、およびテンプレートを使用してインデックスファイルを作成します。
<!doctype html> <html> <head> <title>Popup demo</title> <link rel="stylesheet" href="css/main.css" /> <!-- libs --> <script type="text/javascript" src="js/lib/jquery.js"></script> <script type="text/javascript" src="js/lib/jquery-ui.js"></script> <script type="text/javascript" src="js/lib/underscore.js"></script> <script type="text/javascript" src="js/lib/backbone.js"></script> <!-- app --> <script type="text/javascript" src="js/app/app.js"></script> <script type="text/javascript" src="js/app/views/PopupView.js"></script> <script type="text/javascript" src="js/app/views/ChildPopupView.js"></script> <!-- templates --> <script id="popup-template" type="text/template"> <div class="title"> <h1><%= title %></h1> </div> <div class="content"> <%= content %> </div> <div class="popup-close"> </div> </script> <script id="child-popup-template" type="text/template"> <div class="title"> <h1><%= title %></h1> <h3>i am a child</h3> </div> <div class="content"> <%= content %> </div> <div class="popup-close"> </div> </script> </head> <body> <button id="popup-button">popup</button> <button id="child-popup-button">child popup</button> </body> </html>
「body」には、ポップアップを作成するボタンが2つしかありません。
app.js
このファイルでは、ボタンをクリックするとポップアップが作成され、DOMに追加されます。
function getRandomInt(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } $(function () { $('#popup-button').click(function () { var popup = new PopupView(); $('body').append(popup.render().el); }); $('#child-popup-button').click(function () { var popup = new ChildPopupView(); $('body').append(popup.render().el); }); });
また、ランダムな整数を取得するための関数をここに詰め込みましたが、後で便利になります。
そして今、楽しい部分です!
Popupview.js
var PopupView = Backbone.View.extend({ className: 'popup', events: { 'click .popup-close': 'close', 'mousedown': 'raise' }, initialize: function () { this.template = $('#popup-template').html(); this.width = '400px'; this.height = '350px'; this.top = getRandomInt(100, 400) + 'px'; this.left = getRandomInt(200, 500) + 'px'; this.context = { title: 'Default Title', content: 'Lorem ipsum dolor sit amet.' } $(this.el).css({ 'width': this.width, 'height': this.height, 'top': this.top, 'left': this.left, 'position': 'absolute' }); $(this.el).draggable(); }, /** * . */ render: function () { $(this.el).html(_.template(this.template, this.context)); // $('.popup-active').removeClass('popup-active'); $(this.el).addClass('popup-active'); // z-index, var max_z = 100; $('.popup').each(function () { var curr_z = parseInt($(this).css('z-index')); if (curr_z > max_z) { max_z = curr_z; } }); $(this.el).css({ 'z-index': max_z + 10 }); return this; }, /** * * . */ raise: function (e) { if (!$(this.el).hasClass('popup-active')) { var max_z = 0; $('.popup-active').removeClass('popup-active'); $('.popup').each(function () { var curr_z = parseInt($(this).css('z-index')); if (curr_z > max_z) { max_z = curr_z; } }); $(this.el).css({ 'z-index': max_z + 10 }); $(this.el).addClass('popup-active') } }, /** * DOM. */ close: function () { $(this.el).remove(); var max_z = 0; var top = null; // $('.popup').each(function () { var curr_z = parseInt($(this).css('z-index')); if (curr_z > max_z) { max_z = curr_z; top = this; } }); // if (top) { $(top).addClass('popup-active'); } } });
ここでは、他のすべてのポップアップの親になるポップアップについて説明しました。これは、すべての一般的な機能を実装しています。
さて、いくつかの新しい動作を実装するか、別のテンプレートを使用する新しいポップアップを作成するには、数行のコードのみが必要です。
ChildPopupView.js
var ChildPopupView = PopupView.extend({ initialize: function () { ChildPopupView.__super__.initialize.call(this); this.template = $('#child-popup-template').html(); this.context = { title: 'Child Title', content: 'Lorem ipsum dolor sit amet.' } } });
この子孫では、別のテンプレートを接続し、ポップアップのタイトルを変更しました。 とても簡単です!
デモコード