記事の残りの部分では、これらすべてがどのように見え、どのように実装されているかをより詳細に示します。
すべて次のようになります。

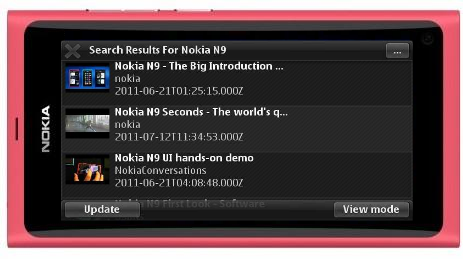

まず、JavaScriptでloadYouTube()関数を作成します。これは、HTTPリクエストを介してYouTubeからJSONオブジェクトを読み込み、解析します。 デフォルトでは、Nokia N9でビデオ検索rssModel.tagsのタグを設定し、表示されるコンテンツの数は32です。次は、この関数のコードです。
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
function loadYouTube(){ var doc = new XMLHttpRequest(); doc.onreadystatechange = function () { if (doc.readyState == XMLHttpRequest.DONE) { var jsresp = JSON.parse(doc.responseText); var entries = jsresp.feed.entry; var len = entries.length rssModel.clear(); var vi=1; for ( var i = 0; i < len; i++) { var obj = entries[i]; rssModel.append( { number: i + 1, url: ( function () { try { return (obj.id.$t.replace( "http://gdata.youtube.com/feeds/api/videos/" , "" ));} catch (e) { return ( "(no title)" ); } } )(), videoRTSP: ( function () { try { return (obj.media$group.media$content[1].url); } catch (e) { return ( "(no title)" ); }})(), videoHTTP: ( function () { try { return (obj.media$group.media$content[0].url); } catch (e) { return ( "(no title)" ); }})(), tags: ( function () { try { return (obj.yt$statistics.viewCount); } catch (e) { return ( "(no title)" ); }})(), photoType: ( function () { try { return (obj.category[0].term); } catch (e) { return ( "(no title)" ); }})(), description: ( function () { try { return (obj.content.$t); } catch (e) { return ( "(no title)" ); }})(), photoAuthor: ( function () { try { return (trimString(obj.author[0].name.$t,35)); } catch (e) { return ( "(no title)" ); }})(), photoHeight: ( function () { try { return (obj.media$group.media$thumbnail[0].height); } catch (e) { return ( "(no title)" ); }})(), photoWidth: ( function () { try { return (obj.media$group.media$thumbnail[0].width); } catch (e) { return ( "(no title)" ); }})(), photoDate: ( function () { try { return (obj.published.$t); } catch (e) { return ( "(no title)" ); }})(), imagePath: ( function () { try { return (obj.media$group.media$thumbnail[0].url); } catch (e) { return ( "(no title)" ); }})(), title: ( function () { try { return (trimString(obj.title.$t,35)); } catch (e) { return ( "(no title)" ); }})() }); } console.log( "Successfully" ) } } doc.open( "GET" , "http://gdata.youtube.com/feeds/api/videos?q=" +rssModel.tags+ "&orderby=viewCount&alt=json&max-results=32" ); doc.send(); }
YouTubeからダウンロードおよび解析されたすべてのデータは、QML ListModelに追加されます。
- ListModel {id:rssModel; プロパティ文字列タグ: "Nokia N9" ;}
上記のスクリーンショットに示されている形式でデータを表示するには、作成したモデルをグリッドビューおよびリストビュー要素のモデルプロパティに追加します。
- Mobile.GridDelegate {id:gridDelegate}
- GridView {
- id:photoGridView; モデル:rssModel; デリゲート :gridDelegate; cacheBuffer:100
- cellWidth:(parent.width-2)/ 4; cellHeight:cellWidth; 幅:parent.width; height:parent.height-1;
- }
- Mobile.ListDelegate {id:listDelegate}
- ListView {
- id:photoListView; モデル:rssModel; デリゲート :listDelegate;
- 幅:parent.width; height:parent.height; x:-(parent.width * 1.5); cacheBuffer:100;
- }
ユーザーが選択したアイテムをクリックすると、Qt.openUrlExternally()メソッドを呼び出します。これにより、デフォルトのプレーヤーでストリーミングビデオが開きます。
- MouseArea {
- id:listMouseArea
- anchors.fill:親
- onClicked:Qt.openUrlExternally(videoRTSP);
- }
タグと表示される要素の数を選択するためのメニュー、コンテンツを更新し、検索結果の表示方法を変更するためのボタンをアプリケーションに追加します-これでプレーヤーは準備完了です。