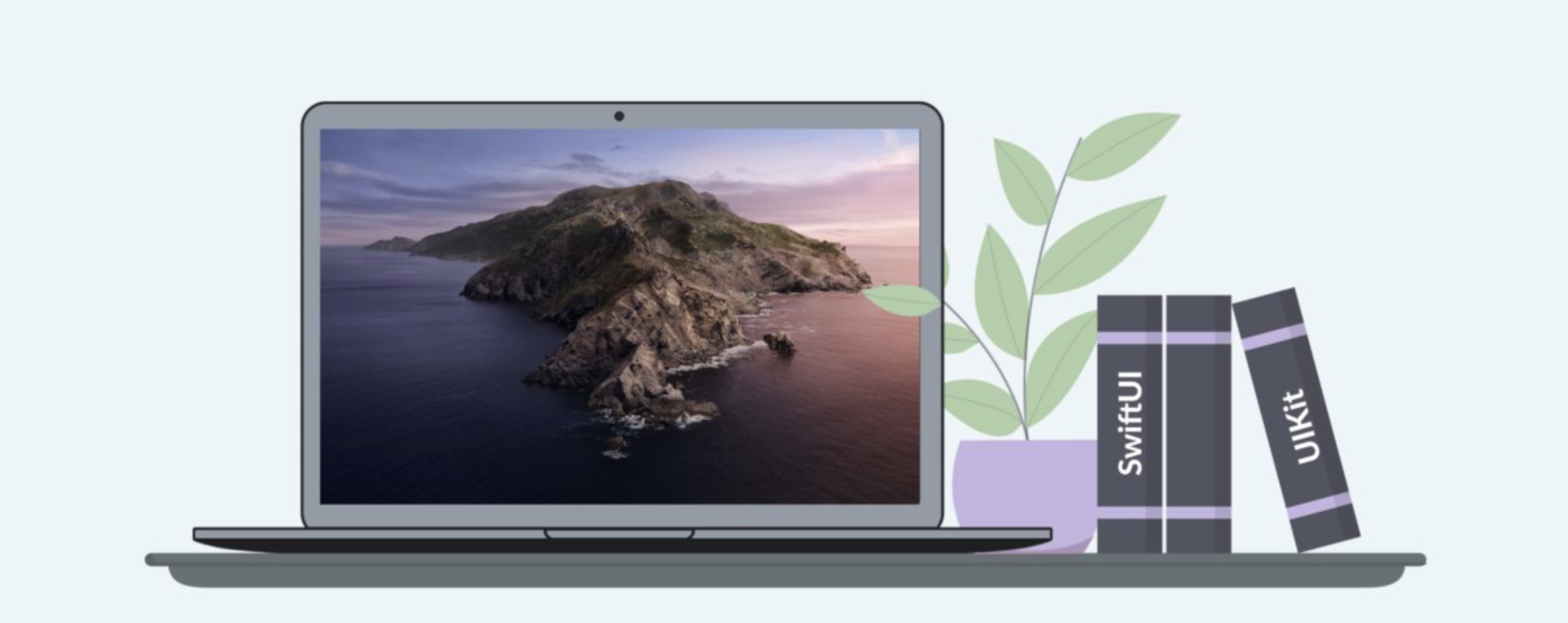
Last week, I saw that the community is trying to transfer proven development patterns from UIKit to SwiftUI. But I’m sure that the best way to write efficient code using SwiftUI is to forget everything about UIKit and completely change your thinking in terms of user interface development. This week I will talk about the main differences between development using UIKit and SwiftUI.
Difference Definition
UIKit is an imperative event-driven framework for creating user interfaces for the iOS platform. This means that you must handle all state changes during events such as loading a view, clicking a button, etc. A big disadvantage of this approach is the difficulty of synchronizing the user interface with its state. Once the state changes, you must manually add / delete / show / hide the views and synchronize it with the current state of the application.
SwiftUI is a declarative framework for creating user interfaces for all Apple platforms. The keyword in this case is declarative. Declarative means that the developer needs to declare what he wants to achieve, and the framework will take care of this. The framework knows the best way to render the user interface.
UI = f(state)
In SwiftUI, the user interface is a function of its state. This means that whenever the state of a view changes, it recounts its body property and generates a new view. Consider the following example.
struct ContentView: View { @ObservedObject var store: Store var body: some View { Group { if store.isLoading { Text("Loading...") .font(.subheadline) } else { Image("photo") .font(.largeTitle) } } } }
In the above example, there is a view that displays the text “Loading ...” and displays images at the end of the download. ObserverObject is the state of this view, and as soon as it changes, SwiftUI recalculates the body property and assigns a new view. During development using UIKit, it is necessary to manually hide / show the elements of the presentation hierarchy, but in SwiftUI there is no need to add / remove a loading indicator. There are several ways in SwiftUI to describe the state of a view, to learn more about them, take a look at “ Property Wrappers in SwiftUI ”.
Now we’ll take a closer look at what happens when a view changes state. SwiftUI has an imprint of the current hierarchy of views and, as soon as its state changes, it calculates a new view. SwiftUI uses comparison algorithms to understand the differences and automatically add / remove / update the necessary views. By default, SwiftUI uses the standard in / out transition image to show / hide views, but it is also possible to manually change the transition to any other animation. To learn more about transition and animations in SwiftUI, take a look at my article “ Animations in SwiftUI ”.
Presentation hierarchy
Let's talk about the hierarchy of views and how SwiftUI actually displays the structure of the views. The very first thing I want to mention is that SwiftUI does not display the structure of the view by one-to-one mapping. It is possible to use as many view containers as you like, but in the end, SwiftUI displays only those views that make sense for rendering. This means that you can extract their logic into small representations, and then compose and reuse them in the application. Do not worry, the application performance in this case will not suffer. To learn more about creating views in SwiftUI, take a look at this article .
The best way to understand the hierarchy of complex representations in SwiftUI is to display its type. SwiftUI uses a static type system to determine the difference quickly enough. First of all, it checks the type of view, and then checks the values of the components of the view. I am not a fan of using reflections in working code, but it is very useful in the learning process.
print(Mirror(reflecting: ContentView(store: .init()).body)) // Group<_ConditionalContent<Text, ModifiedContent<Image, _EnvironmentKe
Using the Mirror structure, you can display the actual type of the ContentView body and find out how SwiftUI works.
conclusions
This week, we learned the main difference between UIKit and SwiftUI and examined in detail the comparison algorithm in SwiftUI. I hope you enjoyed the article. Thanks for reading and see you next week!