If you have never edited the RouteServiceProvider file, welcome to cat.
If you are working with a small project and you have only a couple of routes, then there will be no problems. But when the number of routes is too large, working in one file becomes difficult, especially when you have pages for different users, administrators, etc.
Initially, laravel creates 4 files:
- api.php
- console.php
- channels.php
- web.php
Suppose you plan to create a project with ten pages for each type of user:
- Administrator [site settings, statistics, etc. ]
- User [registration, login, profile management, etc. ]
- Guests [blog, contacts, etc. ]
Create two directories inside routes :
- web - here are all the routes associated with the web interface.
- api - here are all API related routes
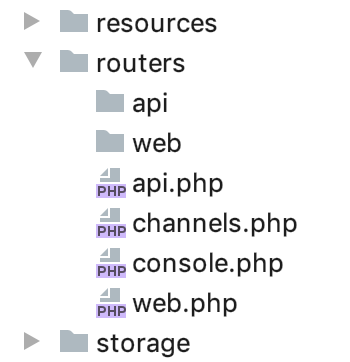
We move api.php from routes to the routes / api and web.php directory in routes / web , and leave the remaining console.php and channels.php in routes.
Create the admin.php file inside routes / web . This file will contain all of our web routes associated with the administrator, and then create user.php there, for user-related routes.

app / Providers / RouteServiceProvider.php - this file is responsible for downloading all the routes of our application. The map () method calls the mapApiRoutes () and mapWebRoutes () methods to load the web.php and api.php files that we have already moved, so let's fix the paths to the route files.
protected function mapWebRoutes() { Route::middleware('web') ->namespace($this->namespace) ->group(base_path('routes/web/web.php')); }
protected function mapApiRoutes() { Route::prefix('api') ->middleware('api') ->namespace($this->namespace) ->group(base_path('routes/api/api.php')); }
Now create new methods for routes / web / admin.php and routes / web / user.php inside RouteServiceProvider.php
protected function mapAdminWebRoutes() { Route::middleware('web') ->namespace($this->namespace) ->prefix('admin') ->group(base_path('routes/web/admin.php')); }
protected function mapUserWebRoutes() { Route::middleware('web') ->namespace($this->namespace) ->prefix('user') ->group(base_path('routes/web/user.php')); }
Please note that in this code it is possible to add namespace, middleware, prefix, etc. for paths.
Next, just call them from map ():
public function map() { $this->mapApiRoutes(); $this->mapWebRoutes(); $this->mapAdminWebRoutes(); $this->mapUserWebRoutes(); }
Last step:
Open routes / web / user.php and add a test route:
Route::get('/test', function () { return response(' ', 200); });
Go to site.local / user / test, you should see the text โTest Routeโ.