So, we continue our journey to more F # types. This time we will look at the types of Records.
How do I create a post
When you create a new F # entry, it may remind you of anonymous objects in C #. This is how you create them. I think they are very similar to anonymous objects in C #, so if you used C #, the F # entries should not be complicated.
It all starts with creating a new type for recording. The type definition lists the name of the properties, as well as the type of properties, as can be seen below.
If you have a record type definition, you can associate a new instance with a value using Let. Again, an example of this can be seen below, where we bind a new record using the Let binding, and we also print the properties of the record instance into the console output.
type Person = { Age : int; Sex: string; Name:string; } .... .... .... .... let sam = { Age = 12; Sex="Male"; Name ="Sam" } printfn "let sam = { Age = 12; Sex=\"Male\"; Name=\"Sam\" }" printfn "Person with Age is %i and Sex is %s and Name is %s" sam.Age sam.Sex sam.Name
How can I change / clone a record
You can clone and modify the entry, which is usually done as follows:
let sam = { Age = 12; Sex="Male"; Name ="Sam" } let tom = { sam with Name="Tom" } printfn "let tom = { sam with Name=\"Tom\" }" printfn "Person with Age is %i and Sex is %s and Name is %s" tom.Age tom.Sex tom.Name
Notice how we used the with keyword when creating a new instance of Tom Person. This form of record expression is called “
copy and update record expression ”. Another option that you can use (again, we will talk about this in more detail in the next post) is to use a mutable property in your post type. Entries are
immutable by default; however, you can also explicitly specify a mutable field.
Here's an example, notice how I created a new type that has a mutable property called MutableName. By defining a mutable field, I am allowed to update the value of the MutableName property of a record, which you can do using the "<-" operator. Which just allows you to assign a new value.
type MutableNamePerson = { Age : int; Sex: string; mutable MutableName:string; } .... .... .... .... //create let sam = { Age = 12; Sex="Male"; MutableName ="Sam" } printfn "let sam = { Age = 12; Sex=\"Male\"; Name=\"Sam\" }" printfn "Person with Age is %i and Sex is %s and Name is %s" sam.Age sam.Sex sam.MutableName //update sam.MutableName <- "Name changed" printfn "sam.MutableName <- \"Name changed\"" printfn "Person with Age is %i and Sex is %s and Name is %s" sam.Age sam.Sex sam.MutableName
Comparison of Records
Record types are equal only if ALL properties are considered the same. Here is an example:
type Person = { Age : int; Sex: string; Name:string; } .... .... .... .... let someFunction p1 p2 = printfn "p1=%A, and p2=%A, are they equal %b" p1 p2 (p1=p2) let sam = { Age = 12; Sex = "Male"; Name = "Sam" } let john = { Age = 12; Sex = "Male"; Name = "John" } let april = { Age = 35; Sex = "Female"; Name = "April" } let sam2 = { Age = 12; Sex = "Male"; Name = "Sam" } do someFunction sam john do someFunction sam april do someFunction sam sam2
Record Comparison Patterns
Of course, you can use pattern matching (discussion the next day), which is the main F # method, to match record types. Here is an example:
type Person = { Age : int; Sex: string; Name:string; } ..... ..... ..... ..... let someFunction (somePerson :Person) = match somePerson with | { Name="Sam" } -> printfn "Sam is in the house" | _ -> printfn "you aint Sam, get outta here clown" let sam = { Age = 12; Sex="Male"; Name ="Sam" } let john = { Age = 12; Sex="Male"; Name ="John" } do someFunction sam do someFunction john
Methods and Properties
It is also possible to add additional members to the entries. Here is an example where we add the “Details” property to allow us to get complete information about the record using one property (as much as we could achieve by overriding the ToString () method, but more on OOP methods later)
Please note that if you try to add an item to the recording type definition, as shown in the screenshot, you will get a strange error
This is easy to solve by simply placing the record property definitions in a new line and making sure that the element also starts on a new line and observe this indentation (space), since all this is important in F #:
type Person = { Age : int; Sex: string; Name:string; } member this.Details = this.Age.ToString() + " " + this.Sex.ToString() + " " + this.Name .... .... .... .... let sam = { Age = 12; Sex = "Male"; Name = "Sam" } printfn "sam Details=%s" sam.Details
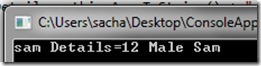