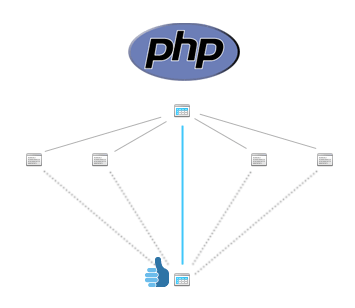
はじめに
ZendX_Console_Process_Unixを使用すると、開発者はプロセスを装ってオブジェクトを作成できるため、複数のタスクを並行して実行できます。 実装の仕様により、この機能は現在、Linux、Solaris、Mac / OSX、およびCLIまたはCGIモードの他のシステムなどのnixシステムでのみ利用可能です。 さらに、このコンポーネントが機能するには、 共有メモリ 、 プロセス制御、およびPOSIXのモジュールが必要です。 要件の1つが満たされない場合、例外がスローされます。
ZendX_Console_Process_Unixの使用の基本
ZendX_Console_Process_Unixは、ユーザーが拡張する必要がある抽象クラスです。 特定のデータを処理するために実装されたアルゴリズムを記述する必要がある唯一の抽象メソッド_run()が含まれています。 このクラスは、プロセスのステータスを確認し、メインプロセスと子プロセスの間で変数を交換するためのメソッドも定義します。
_run()メソッドとその内部で呼び出される各メソッドは、個別のプロセスで実行されます。 アプリケーションで呼び出される他のメソッドは、親プロセスで実行されます。
setVariable()およびgetVariable()メソッドを使用して、親プロセスと子プロセスの間で変数を渡すことができます。 子プロセスのステータスを監視するには、その内部で_setAlive()メソッドを短い間隔で呼び出す必要があります。これにより、親プロセスはgetLastAlive()メソッドを呼び出して子プロセスの状態に関する情報を取得できます。 子プロセスのPIDを取得するために、親はgetPid()を呼び出すことができます。
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
class MyProcess extends ZendX_Console_Process_Unix { protected function _run() { for ($i = 0; $i < 10; $i++) { // Doing something really important which can't wait: sleeping sleep(1); } } } // This part should last about 10 seconds, not 20. $process1 = new MyProcess(); $process1->start(); $process2 = new MyProcess(); $process2->start(); while ($process1->isRunning() && $process2->isRunning()) { sleep(1); } echo 'All processes completed' ; * This source code was highlighted with Source Code Highlighter .
この例では、プロセスは2つに並列化され、開始されます。 各子プロセスは10秒間実行されるため、親プロセスは20秒ではなく10秒以内に完了します。
PS
Zend Frameworkを非常に積極的に使用しており、このコンポーネントが16か月以上(2008年10月31日から)使用可能であるという事実にもかかわらず、私はその存在のみを知りました。