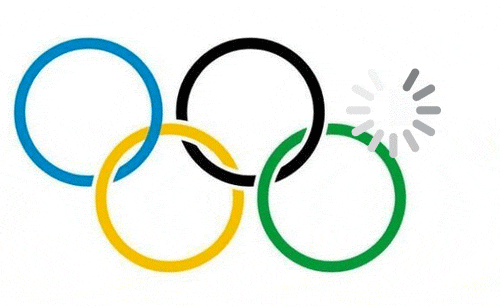
今日はasync / awaitの6つの機能に注目します。これにより、可能な限りマスターし使用するツールとして非同期コードを記述するための新しいアプローチを分類し、以前のものに置き換えることができます。
非同期/待機の基本
async / awaitが初めての人のために、先に進む前に知っておくべき基本的なことをいくつか紹介します。
- Async / awaitは、非同期コードを記述する新しい方法です。 以前は、コールバックとプロミスを使用して同様のコードが記述されていました。
- 「約束を忘れる」という私たちの提案は、新技術の観点からそれらが関連性を失ったことを意味するものではありません。 実際、async / awaitはpromiseに基づいています。 このメカニズムはコールバックでは使用できないことに注意してください。
- promiseなどのasync / awaitを使用して構築されたコンストラクトは、プログラム実行のメインスレッドをブロックしません。
- async / awaitのおかげで、非同期コードは同期に似たものになり、その動作には、さまざまな理由でプロミスを使用するのが不便だった状況で非常に役立つコードの機能があります。 しかし、以前は、彼らはそれができませんでした。 今、すべてが変更されました。 これが、非同期/待機の力のあるところです。
構文
,
getJSON
, , JSON-. , JSON-
done
.
:
const makeRequest = () => getJSON() .then(data => { console.log(data) return "done" }) makeRequest()
async/await:
const makeRequest = async () => { console.log(await getJSON()) return "done" } makeRequest()
, :
-
async
.await
,async
. , , , ,return
,done
.
- ,
await
async-, .
// // await makeRequest() // - makeRequest().then((result) => { // do something })
-
await getJSON()
,console.log
getJSON()
, , .
async/await ?
async/await .
▍1.
, , , async/await, . , , , , . ,
.then
, ,
data
, , , . , . , .
▍2.
async/await —
try/catch
.
try/catch
,
JSON.parse
, .
.catch()
.
console.log
.
const makeRequest = () => { try { getJSON() .then(result => { // JSON const data = JSON.parse(result) console.log(data) }) // // .catch((err) => { // console.log(err) // }) } catch (err) { console.log(err) }
, async/await.
catch
, JSON.
const makeRequest = async () => { try { // JSON const data = JSON.parse(await getJSON()) console.log(data) } catch (err) { console.log(err) } }
▍3.
, , , , , , , , , -. :
const makeRequest = () => { return getJSON() .then(data => { if (data.needsAnotherRequest) { return makeAnotherRequest(data) .then(moreData => { console.log(moreData) return moreData }) } else { console.log(data) return data } }) }
. ( 6 ), , , , .
, async/await.
const makeRequest = async () => { const data = await getJSON() if (data.needsAnotherRequest) { const moreData = await makeAnotherRequest(data); console.log(moreData) return moreData } else { console.log(data) return data } }
▍4.
, ,
promise1
, , ,
promise2
,
promise3
. , .
const makeRequest = () => { return promise1() .then(value1 => { // do something return promise2(value1) .then(value2 => { // do something return promise3(value1, value2) }) }) }
promise3
value1
, , , .
value1
value2
Promise.all
.
const makeRequest = () => { return promise1() .then(value1 => { // do something return Promise.all([value1, promise2(value1)]) }) .then(([value1, value2]) => { // do something return promise3(value1, value2) }) }
. ,
value1
value2
, .
async/await, , , , . , , - .
const makeRequest = async () => { const value1 = await promise1() const value2 = await promise2(value1) return promise3(value1, value2) }
▍5.
, , - .
const makeRequest = () => { return callAPromise() .then(() => callAPromise()) .then(() => callAPromise()) .then(() => callAPromise()) .then(() => callAPromise()) .then(() => { throw new Error("oops"); }) } makeRequest() .catch(err => { console.log(err); // // Error: oops at callAPromise.then.then.then.then.then (index.js:8:13)
, , , . , . , —
callAPromise
, (, , — , , ).
async/await, , .
const makeRequest = async () => { await callAPromise() await callAPromise() await callAPromise() await callAPromise() await callAPromise() throw new Error("oops"); } makeRequest() .catch(err => { console.log(err); // // Error: oops at makeRequest (index.js:7:9) })
, , . , , -, -. ,
makeRequest
, , , —
then
,
then
, -
then
…
▍6.
, , . async/await , , , . .
1. , ( ).

-
2.
.then
« » (step-over),
.then
, «» .
async/await , «» ,
await
, .

async/await
, async/await, . .
- . , , , ,
.then
, , async/await. , , C# , , , , .
- Node 7 — LTS-. , Node 8, , , .
, async/await — , JavaScript . . , async/await , .
! async/await? ?