以下に、私の創造物を裁判所に提出します。 最初は、次の資料に依存していました。 私に関しては、非常に興味深いですが、単純なものではありますが、完全なアプリケーションを作成することは常に興味深いものです。 それで、何が起こったのか...
上記の方法のように、初期ベースは変更されていません。
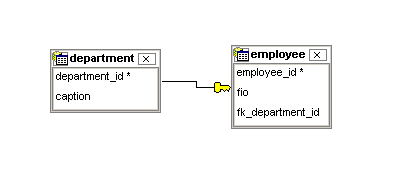
実装する必要がある操作のリストは次のようになります。
- 新しい部門を追加します。
- 部門データの編集。
- 部門を削除します。
- 部門データを表示します。
- コードで部門を見つけます。
- 新しい従業員を追加します。
- 部門別に従業員を表示します。
- 従業員を削除します。
最初のステップは、ベースへのマッピングの形成でした。 自分で調べた資料から、xmlファイルを使用する方法と注釈を使用する方法の2つがあることに気付きました。 認識を簡素化するために、従業員部門の私の例の個別のxmlファイルは次のようになります。
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="logic.Department" table="department" schema="joblist"> <id name="departmentId"> <column name="department_id" sql-type="int(11)"/> <generator class="increment"/> </id> <property name="caption"> <column name="caption" sql-type="text" not-null="true"/> </property> <set name="employies" lazy="false" cascade="all" inverse="true"> <key column="fk_department_id"/> <one-to-many class="logic.Employee"/> </set> </class> </hibernate-mapping>
すぐに最初の免責事項、ファイルにはヘッダーが含まれている必要があります... <?Xml version = '1.0' encoding = 'utf-8'?>など
次のような注釈を使用して同じことを実装しようとしました。
@Entity @Table(name="department") public class Departmant { private long id; private String name; public Department() { }
@で始まる行は注釈です。 正確に何を使用するかは、著者自身が決定します。 どちらのバージョンにも寿命があります。
従業員の2番目のマッピングは次のようになります。
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="logic.Employee" table="employee" schema="joblist"> <id name="employeeId"> <column name="employee_id" sql-type="int(11)"/> <generator class="increment"/> </id> <property name="fio"> <column name="fio" sql-type="text" not-null="true"/> </property> <many-to-one name="department" class="logic.Department" column="fk_department_id" not-null="true" cascade="save-update"/> </class> </hibernate-mapping>
すぐに関係の形成についてコメントします。 1-M通信が使用される(1対多)ため、基本エンティティ(部門)の側から、以下を示します。
<set name="employies" lazy="false" cascade="all" inverse="true">
従属テーブルとの遅延結合は削除されました(これは完全に正しいわけではないかもしれませんが、私はまだ見ていきます)。 DBMSを使用します)。
下位エンティティの側面から、以下を示します。
<many-to-one name="department" class="logic.Department" column="fk_department_id" not-null="true" cascade="save-update"/>
つまり、キーフィールド(外部キー)をゼロにすることはできず(データベースレベルで整合性を確保する)、カスケード操作を保存または更新することができます(そうでない場合、従業員が削除されると、彼のネイティブ部門も飛び立ちます)。
多くの例に示すように、DepartmentクラスとEmployeeクラスは典型的なPOJOです。
package logic; import java.util.HashSet; import java.util.Set; public class Department { private int departmentId; private String caption; public Department() { } public Department(String caption) { this.caption = caption; } public int getDepartmentId() { return departmentId; } public void setDepartmentId(int departmentId) { this.departmentId = departmentId; } public String getCaption() { return caption; } public void setCaption(String caption) { this.caption = caption; } Set<Employee> employies = new HashSet<Employee>(); public Set<Employee> getEmployies(){ return employies; } public void setEmployies(Set<Employee> employies) { this.employies = employies; } @Override public String toString() { String string = this.departmentId + " " + this.caption; return string; } }
package logic; public class Employee { private int employeeId; private String fio; private Department department; public Employee() { } public Employee(String fio) { this.fio = fio; } public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getFio() { return fio; } public void setFio(String fio) { this.fio = fio; } public Department getDepartment() { return department; } public void setDepartment(Department department){ this.department = department; } @Override public String toString() { String string = this.employeeId+ " " + this.fio; return string; } }
さらに続きます...クラスを作成する必要があります-ファクトリーは、構成の1回の実行と処理メソッド(インターフェース)のリストを実行します。 シングルトンとして実装されます。
package factory; import DAO.DepartmentDAO; import DAO.EmployeeDAO; import implement.ImplDepartment; import implement.ImplEmployee; public class Factory { private static DepartmentDAO departmentDAO = null; private static EmployeeDAO employeeDAO = null; private static Factory instance = null; public static synchronized Factory getInstance() { if (instance == null) { instance = new Factory(); } return instance; } public DepartmentDAO getDepartmentDAO() { if (departmentDAO == null) { departmentDAO = new ImplDepartment(); } return departmentDAO; } public EmployeeDAO getEmployeeDAO() { if (employeeDAO == null) { employeeDAO = new ImplEmployee(); } return employeeDAO; } }
Hibernate設定-hibernate.cfg.xmlファイルの構造は次のとおりです。
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.url">jdbc:mysql://localhost:3306/Joblist</property> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.username">root</property> <property name="connection.password">admin</property> <property name="connection.pool_size">1</property> <property name="show_sql">true</property> <property name="current_session_context_class">thread</property> <property name="dialect">org.hibernate.dialect.MySQL5InnoDBDialect</property> <mapping resource="logic/Employee.hbm.xml"/> <mapping resource="logic/Department.hbm.xml"/> <mapping class="logic.Department"/> <mapping class="logic.Employee"/> </session-factory> </hibernate-configuration>
次のステップは、作業セッションを形成するクラスを作成することです。 多くの例で考慮されている通常のオプションは機能しますが、ステータスが「非推奨」になっています-使用は推奨されません。 その結果、接続を整理するためのユーティリティの外観は少し異なります。
package utils; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import org.hibernate.service.ServiceRegistryBuilder; public class HibernateUtil { private static SessionFactory sessionFactory; private static ServiceRegistry serviceRegistry; public static final SessionFactory createSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); serviceRegistry = new ServiceRegistryBuilder().applySettings( configuration.getProperties()).buildServiceRegistry(); sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; } }
クラス-テーブルで操作を整理するためのインターフェースは次のようになります。
package DAO; import logic.Department; import logic.Employee; import java.io.IOException; import java.sql.SQLException; import java.util.Collection; public interface DepartmentDAO { public void addDepartment(Department department) throws SQLException; public void editDepartment(Department department) throws SQLException; public void deleteDepartment(Integer department) throws SQLException, IOException; public Collection getDepartmentById(Integer department_id) throws SQLException; public Collection getAllDepartments() throws SQLException; public void addEmployee(Department department, Employee employee) throws SQLException; }
2番目のケースでは、すべてのメソッドが実装されたわけではなく、すぐに予約を行います...
package DAO; import logic.Employee; import java.sql.SQLException; import java.util.Collection; public interface EmployeeDAO { public void editEmployee (Employee employee) throws SQLException; public void deleteEmployee (Employee employee) throws SQLException; public Collection getDepartmentByEmployee (Employee employee) throws SQLException; public Collection getAllEmployies() throws SQLException; public void deleteEmployiesByName(String name) throws SQLException; public Collection getEmployiesByDepartment(Integer department) throws SQLException; }
さらに、メソッドがあるため、それらを実装する必要があります。
package implement; import DAO.DepartmentDAO; import logic.Department; import logic.Employee; import org.hibernate.HibernateException; import org.hibernate.Session; import org.hibernate.criterion.Restrictions; import utils.HibernateUtil; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.sql.SQLException; import java.util.*; public class ImplDepartment implements DepartmentDAO { @Override public void addDepartment(Department department) throws SQLException { Session session = null; try { session = HibernateUtil.createSessionFactory().openSession(); session.beginTransaction(); session.save(department); session.getTransaction().commit(); } catch (HibernateException exception) { System.out.println(" "); exception.printStackTrace(); } finally { if (session != null && session.isOpen()) { session.close(); } } } @Override public void editDepartment(Department department) throws SQLException { Session session = null; try { session = HibernateUtil.createSessionFactory().openSession(); session.beginTransaction(); session.update(department); session.getTransaction().commit(); } catch (HibernateException exception) { System.out.println(" "); exception.printStackTrace(); } finally { if (session != null && session.isOpen()) { session.close(); } } } @Override public void deleteDepartment(Integer id) throws SQLException, IOException { Session session = null; try { session = HibernateUtil.createSessionFactory().openSession(); session.beginTransaction(); Department department = (Department) session.load(Department.class, id); Employee[] employies = department.getEmployies().toArray(new Employee[]{}); if (employies.length > 0) { System.out.println(" , ?"); BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); String ch = reader.readLine(); if (ch.equals("Y") || (ch.equals("y"))) { session.delete(department); session.getTransaction().commit(); } } else { session.delete(department); session.getTransaction().commit(); } } catch (HibernateException exception) { System.out.println(" "); exception.printStackTrace(); } finally { if (session != null && session.isOpen()) { session.close(); } } } @Override public Collection getDepartmentById(Integer department_id) throws SQLException { Session session = null; List departments = new ArrayList<Department>(); try { session = HibernateUtil.createSessionFactory().openSession(); departments = session.createCriteria(Department.class).add(Restrictions.idEq(department_id)).list(); } catch (HibernateException exception) { System.out.println(" "); exception.printStackTrace(); } finally { if (session != null && session.isOpen()) { session.close(); } } return departments; } @Override public Collection getAllDepartments() throws SQLException { Session session = null; List departments = new ArrayList<Department>(); try { session = HibernateUtil.createSessionFactory().openSession(); departments = session.createCriteria(Department.class).list(); } catch (Exception e) { System.out.println(" "); } finally { if (session != null && session.isOpen()) { session.close(); } } return departments; } @Override public void addEmployee(Department department, Employee employee) throws SQLException { Session session = null; try { session = HibernateUtil.createSessionFactory().openSession(); session.beginTransaction(); department.getEmployies().add(employee); employee.setDepartment(department); session.saveOrUpdate(department); session.saveOrUpdate(employee); session.getTransaction().commit(); } catch (HibernateException exception) { exception.printStackTrace(); System.out.println(" "); } finally { if (session != null && session.isOpen()) { session.close(); } } } }
package implement; import DAO.EmployeeDAO; import logic.Department; import logic.Employee; import org.hibernate.HibernateException; import org.hibernate.Session; import org.hibernate.criterion.Restrictions; import utils.HibernateUtil; import java.sql.SQLException; import java.util.*; public class ImplEmployee implements EmployeeDAO { @Override public void editEmployee(Employee employee) throws SQLException { Session session = null; try { session = HibernateUtil.createSessionFactory().openSession(); session.beginTransaction(); session.update(employee); session.getTransaction().commit(); } catch (HibernateException exception) { System.out.println(" "); exception.printStackTrace(); } finally { if (session != null && session.isOpen()) { session.close(); } } } @Override public void deleteEmployee(Employee employee) throws SQLException { } @Override public Collection getDepartmentByEmployee(Employee employee) throws SQLException { return null; } @Override public Collection getAllEmployies() throws SQLException { return null; } @Override public void deleteEmployiesByName(String name) throws SQLException { Session session = null; List employies = new ArrayList<Employee>(); try { session = HibernateUtil.createSessionFactory().openSession(); session.beginTransaction(); employies = session.createCriteria(Employee.class).add(Restrictions.like("fio", name)).list(); Iterator iterator = employies.iterator(); while (iterator.hasNext()) { Employee data = (Employee) iterator.next(); String nameTemp = data.getFio(); if (nameTemp.equals(name)){ int id = data.getEmployeeId(); Object person = session.load(Employee.class, id); session.delete(person); } } session.getTransaction().commit(); } catch (HibernateException exception) { System.out.println(" "); exception.printStackTrace(); } finally { if (session != null && session.isOpen()) { session.close(); } } } @Override public Collection getEmployiesByDepartment(Integer department) throws SQLException { Session session = null; List departments = new ArrayList<Department>(); Collection employies = null; try { session = HibernateUtil.createSessionFactory().openSession(); departments = session.createCriteria(Department.class).add(Restrictions.idEq(department)).list(); Iterator iterator = departments.iterator(); while (iterator.hasNext()) { Department data = (Department) iterator.next(); employies = data.getEmployies(); } } catch (Exception e) { System.out.println(" "); } finally { if (session != null && session.isOpen()) { session.close(); } } return employies; } }
結果がNullのダミーは、まだ実装されていないメソッドです。 ただし、メインクラスからは要求されないため、これに問題はありません。いつでも終了できます。 そして原則として、方法の構成は状況に応じて変化します。
そして最後に、実装されたアイデアの正確性をチェックするメインモジュール。
package main; import factory.Factory; import logic.Department; import logic.Employee; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.sql.SQLException; import java.util.Collection; import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class Main { public static void addDepartment() throws IOException, SQLException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println(" : "); String data = reader.readLine(); Department department = new Department(); department.setCaption(data); Factory.getInstance().getDepartmentDAO().addDepartment(department); } public static void editDepartment() throws IOException, SQLException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println(" : "); int num = 0; String data = reader.readLine(); try { num = Integer.parseInt(data); Collection collection; collection = Factory.getInstance().getDepartmentDAO().getDepartmentById(num); Set<Department> set = new HashSet<>(collection); Iterator iterator = set.iterator(); while (iterator.hasNext()) { Department department = (Department) iterator.next(); Integer id = department.getDepartmentId(); String name; System.out.println(" "); name = reader.readLine(); Department temporal = new Department(); temporal.setDepartmentId(id); temporal.setCaption(name); Factory.getInstance().getDepartmentDAO().editDepartment(temporal); } } catch (NumberFormatException e) { System.out.println(" "); } } public static void getDepartments() throws SQLException { Collection collection; collection = Factory.getInstance().getDepartmentDAO().getAllDepartments(); showDepartments(collection); } public static void findDepartments() throws SQLException, IOException { System.out.println(" "); BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); String data = reader.readLine(); try { int num = Integer.parseInt(data); Collection collection; collection = Factory.getInstance().getDepartmentDAO().getDepartmentById(num); showDepartments(collection); } catch (NumberFormatException e) { System.out.println(" "); } } public static void findEmployies() throws SQLException, IOException { System.out.println(" "); int num = 0; BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); try { String data = reader.readLine(); num = Integer.parseInt(data); Collection collection = Factory.getInstance().getDepartmentDAO().getDepartmentById(num); showDepartments(collection); if (collection.size() != 0) { Collection employies = Factory.getInstance().getEmployeeDAO().getEmployiesByDepartment(num); showEmployers(employies); } else System.out.println(" "); } catch (NumberFormatException e) { System.out.println(" "); } } public static void showDepartments(Collection collection) throws SQLException { Set<Department> set = new HashSet<Department>(collection); Iterator iterator = set.iterator(); System.out.println("===================================="); System.out.printf("%-20s%-20s%n", " ", " "); System.out.println("===================================="); while (iterator.hasNext()) { Department data = (Department) iterator.next(); System.out.printf("%-20s%-20s%n", data.getDepartmentId(), data.getCaption()); } } public static void showEmployers(Collection collection) throws SQLException { Set<Employee> set = new HashSet<Employee>(collection); Iterator iterator = set.iterator(); System.out.println("===================================="); System.out.printf("%-20s%n", " "); System.out.println("===================================="); while (iterator.hasNext()) { Employee data = (Employee) iterator.next(); System.out.printf("%-20s%n", data.getFio()); } } public static void deleteDepartment() throws SQLException, IOException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); int num = 0; System.out.println(" "); try { String data = reader.readLine(); num = Integer.parseInt(data); Factory.getInstance().getDepartmentDAO().deleteDepartment(num); } catch (NumberFormatException e) { System.out.println(" "); } } public static void deleteEmployies() throws SQLException, IOException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println(" "); String name = reader.readLine(); Collection collection; Factory.getInstance().getEmployeeDAO().deleteEmployiesByName(name); } public static void addEmployee() throws SQLException, IOException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println(" "); String id = reader.readLine(); try { int num = Integer.parseInt(id); Collection collection; collection = Factory.getInstance().getDepartmentDAO().getDepartmentById(num); showDepartments(collection); Set<Department> set = new HashSet<>(collection); if (set.size() != 0) { System.out.println(" "); String data = reader.readLine(); Employee employee = new Employee(data); Iterator iterator = set.iterator(); while (iterator.hasNext()) { Department department = (Department) iterator.next(); Factory.getInstance().getDepartmentDAO().addEmployee(department, employee); } } else System.out.println(" "); } catch (NumberFormatException e) { System.out.println(" "); } } static public void main(String[] args) throws IOException, SQLException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); while (true) { System.out.println("===================================="); System.out.println(" "); System.out.println("===================================="); System.out.println("1 - "); System.out.println("2 - "); System.out.println("3 - "); System.out.println("4 - "); System.out.println("5 - "); System.out.println("===================================="); System.out.println("6 - "); System.out.println("7 - "); System.out.println("8 - "); System.out.println("===================================="); System.out.println("0 - "); System.out.println("===================================="); String num = reader.readLine(); switch (num) { case "1": addDepartment(); break; case "2": editDepartment(); break; case "3": deleteDepartment(); break; case "4": getDepartments(); break; case "5": findDepartments(); break; case "6": addEmployee(); break; case "7": findEmployies(); break; case "8": deleteEmployies(); break; case "0": System.exit(0); break; } } } }
結論:あなたが注意を払う必要があるもの。 まず、マッピングとリンクの構成のための正しいファイル構造。 エラー結果はあなたを緊張させます。 第二に、セッションの新しい組織。 3番目に、自動モードでPOJOを形成することを提案する方法では、この場合、設計プロセス中にデータベースへの接続を確立する必要があります。 結果は注釈(クラス内のデータベースコンポーネントの説明)の形式になります。
この例は、単純ではありますが、第一印象を得るのに役立つことを願っています。