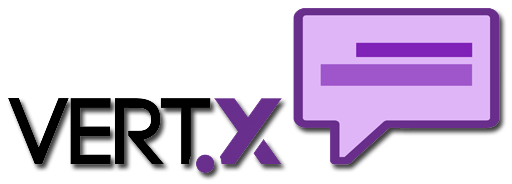
内容
Vert.xとは何ですか?
Vert.xは、JVM上で実行されるイベント駆動型フレームワークです。 現時点では、このフレームワークの最新バージョンは3.2です。 Vert.x 3は次の機能を提供します。
- 多言語主義 。 アプリケーションコンポーネントは、Java、JavaScript、Scala、Python、Ruby、Groovy、およびClojureで開発できます。
- 並行性 マルチスレッドプログラミングの手間を省く、非常に単純な同時実行モデル。
- 非同期性 。 ブロックしない非同期相互作用の単純なモデル。
- 分散イベントバス 。 クライアント側とサーバー側の両方を含みます。 チャットの主な役割を直接果たします。
- Java 8 。 Vert.x 3にはJavaバージョン8以降が必要です。
チャットの詳細
アプリケーションはサーバー上で実行され、展開後、匿名チャットのアドレスが公開されます。これは任意のブラウザーから参加できます。 このアドレスで、アプリケーションはすべてのユーザーからリアルタイムでメッセージをブロードキャストします。
行こう!
開発は、IntelliJ IDEA 15、十分なコミュニティバージョンで行われます。
プロジェクト構造
Mavenプロジェクトを作成します。 残念ながら、vert.x 3の既成のアーキタイプはありません(2の場合は存在します)ので、通常のMavenプロジェクトを生成します。 最終的な構造は次のとおりです。
構造
src +---main | +---java | | | Server.java | | | VerticleLoader.java | | | | | \---webroot | | date-format.js | | index.html | | vertx-eventbus.js | | | \---resources \---test \---java ChatTest.java
pom.xmlでは、次の依存関係を定義します。 vertx-core Verticlesがライブラリをサポートしている場合(詳細は何であるか、もう少し詳しく)、 vertx- web-ユニットテストにイベントハンドラー(だけでなく)とvertx-unitを使用できます。
pom.xml
<dependencies> <dependency> <groupId>io.vertx</groupId> <artifactId>vertx-core</artifactId> <version>3.0.0</version> </dependency> <dependency> <groupId>io.vertx</groupId> <artifactId>vertx-web</artifactId> <version>3.0.0</version> </dependency> <dependency> <groupId>io.vertx</groupId> <artifactId>vertx-unit</artifactId> <version>3.0.0</version> <scope>test</scope> </dependency> </dependencies>
サーバー
このフレームワークの特徴は、すべてのコンポーネントを頂点の形で提示する必要があることです。
Verticleは、サーブレットの類似物であり、デプロイメントの原子単位です。 開発者自身は、Verticleをアクターモデルのアクターに似たものとして説明しています。 実際、この設計により、Vert.xで有名な高度な並列性と非同期性を整理できます。 サーバー実装では、AbstractVerticle抽象クラスを継承します。
オーバーライドするstart()メソッドは、プログラムへのエントリポイントです。 最初に、アプリケーションがデプロイされます-deploy()関数、次にハンドラーがハングアップします-handle()メソッド。
Server.java
public class Server extends AbstractVerticle { private Logger log = LoggerFactory.getLogger(Server.class); private SockJSHandler handler = null; private AtomicInteger online = new AtomicInteger(0); // . @Override public void start() throws Exception { if (!deploy()) { log.error("Failed to deploy the server."); return; } handle(); } //... }
アプリケーションをデプロイするには、空きポートを取得する必要があります。取得できない場合は、hostPortに負の値があります。 次に、ルーターを作成し、その受信者のアドレスを指定して、ハンドラーを切断します。 最後に、アクセス可能なポートでHTTPサーバーを実行します。
Server.java
// . private boolean deploy() { int hostPort = getFreePort(); if (hostPort < 0) return false; Router router = Router.router(vertx); // . handler = SockJSHandler.create(vertx); router.route("/eventbus/*").handler(handler); router.route().handler(StaticHandler.create()); // -. vertx.createHttpServer().requestHandler(router::accept).listen(hostPort); try { String addr = InetAddress.getLocalHost().getHostAddress(); log.info("Access to \"CHAT\" at the following address: \nhttp://" + addr + ":" + hostPort); } catch (UnknownHostException e) { log.error("Failed to get the local address: [" + e.toString() + "]"); return false; } return true; }
空きポートを取得するプロセスは、以下のコードスニペットに示されています。 最初に、静的フィールドPROCESS_ARGSでアプリケーションを起動するための引数がチェックされます。その引数の1つは、ユーザーが指定したアプリケーションデプロイメントポートです。 ポートが指定されなかった場合、デフォルトのポート8080が使用されます。
Server.java
// . private int getFreePort() { int hostPort = 8080; // , // . if (Starter.PROCESS_ARGS != null && Starter.PROCESS_ARGS.size() > 0) { try { hostPort = Integer.valueOf(Starter.PROCESS_ARGS.get(0)); } catch (NumberFormatException e) { log.warn("Invalid port: [" + Starter.PROCESS_ARGS.get(0) + "]"); } } // . if (hostPort < 0 || hostPort > 65535) hostPort = 8080; return getFreePort(hostPort); }
値が0のパラメーターがソケットを作成するためのコンストラクターの引数として指定されている場合、ランダムな空きポートが発行されます。
ポートがすでに使用されている場合(たとえば、ポート8080が別のアプリケーションによって既に使用されているが、同時に、現在のアプリケーションを起動する引数として示されている場合)、BindExceptionがスローされます。その場合、空きポートの取得が繰り返し試行されます。
Server.java
private int getFreePort(int hostPort) { try { ServerSocket socket = new ServerSocket(hostPort); int port = socket.getLocalPort(); socket.close(); return port; } catch (BindException e) { //, . if (hostPort != 0) return getFreePort(0); log.error("Failed to get the free port: [" + e.toString() + "]"); return -1; } catch (IOException e) { log.error("Failed to get the free port: [" + e.toString() + "]"); return -1; } }
展開が成功した場合、イベントバスのリスニングは、chat.to.server(着信イベント)およびchat.to.client(発信イベント)から始まります。
バス上の次のイベントを処理した後、このイベントを確認する必要があります。
Server.java
private void handle() { BridgeOptions opts = new BridgeOptions() .addInboundPermitted(new PermittedOptions().setAddress("chat.to.server")) .addOutboundPermitted(new PermittedOptions().setAddress("chat.to.client")); // . handler.bridge(opts, event -> { if (event.type() == PUBLISH) publishEvent(event); if (event.type() == REGISTER) registerEvent(event); if (event.type() == SOCKET_CLOSED) closeEvent(event); // , // . event.complete(true); }); }
バスで発生するイベントは、次の7つのタイプで表すことができます。
種類 | イベント |
---|---|
SOCKET_CREATED | ソケットの作成時に発生する |
SOCKET_CLOSED | ソケットを閉じるとき |
送信 | クライアントからサーバーにメッセージを送信しようとします |
公開する | サーバーのクライアント投稿 |
受信する | 配信されたメッセージに関するサーバーからの通知 |
登録する | ハンドラーを登録しよう |
登録解除 | 登録されたハンドラーをキャンセルしようとする |
このアプリケーションでは、PUBLISH、REGISTER、およびSOCKET_CLOSEDタイプのイベントのみを処理する必要があります。
タイプPUBLISHのイベントは、ユーザーの1人がチャットにメッセージを送信するとトリガーされます。
REGISTER-ユーザーがハンドラーを登録するとトリガーされます。 SOCKET_CREATEDを選ばないのはなぜですか? なぜなら、タイプSOCKET_CREATEDのイベントはREGISTERの前にあり、そしてもちろん、クライアントがハンドラーを登録するまで、イベントを受け取ることができないからです。
SOCKET_CLOSED-ユーザーがチャットを離れたとき、または予期しない状況が発生したときに発生します。
メッセージが公開されると、ハンドラーが起動し、publishEventメソッドを呼び出します。 宛先アドレスがチェックされ、それが正しい場合、メッセージが取得され、すべてのクライアント(送信者を含む)のイベントバスでチェックおよび公開されます。
Server.java
private boolean publishEvent(BridgeEvent event) { if (event.rawMessage() != null && event.rawMessage().getString("address").equals("chat.to.server")) { String message = event.rawMessage().getString("body"); if (!verifyMessage(message)) return false; String host = event.socket().remoteAddress().host(); int port = event.socket().remoteAddress().port(); Map<String, Object> publicNotice = createPublicNotice(host, port, message); vertx.eventBus().publish("chat.to.client", new Gson().toJson(publicNotice)); return true; } else return false; }
転記の通知生成は次のとおりです。
Server.java
// . private Map<String, Object> createPublicNotice(String host, int port, String message) { Date time = Calendar.getInstance().getTime(); Map<String, Object> notice = new TreeMap<>(); notice.put("type", "publish"); notice.put("time", time.toString()); notice.put("host", host); notice.put("port", port); notice.put("message", message); return notice; }
チャットでのユーザーのログインとログアウトは、次の方法で処理されます。
Server.java
// - . private void registerEvent(BridgeEvent event) { if (event.rawMessage() != null && event.rawMessage().getString("address").equals("chat.to.client")) new Thread(() -> { Map<String, Object> registerNotice = createRegisterNotice(); vertx.eventBus().publish("chat.to.client", new Gson().toJson(registerNotice)); }).start(); } // . private Map<String, Object> createRegisterNotice() { Map<String, Object> notice = new TreeMap<>(); notice.put("type", "register"); notice.put("online", online.incrementAndGet()); return notice; } // - . private void closeEvent(BridgeEvent event) { new Thread(() -> { Map<String, Object> closeNotice = createCloseNotice(); vertx.eventBus().publish("chat.to.client", new Gson().toJson(closeNotice)); }).start(); } // . private Map<String, Object> createCloseNotice() { Map<String, Object> notice = new TreeMap<>(); notice.put("type", "close"); notice.put("online", online.decrementAndGet()); return notice; }
公開されたメッセージの確認は非常に原始的ですが、たとえばこれで十分です。 たとえば、メッセージやその他のハックの形でスクリプトの送信を確認することで、自分で複雑にすることができます。
Server.java
private boolean verifyMessage(String msg) { return msg.length() > 0 && msg.length() <= 140; }
JSON形式はデータ交換に使用されるため、pom.xmlファイルは次の依存関係を追加して更新する必要があります。
pom.xml
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.3.1</version> </dependency>
また、チャットでは、オンラインユーザー数のカウンターが表示されます。 アプリケーションはマルチスレッドであるため、スレッドセーフであることを保証する必要があります。したがって、カウンターをAtomicIntegerとして宣言する最も簡単な方法です 。
お客様
記事の冒頭の構造に示されているように、webrootセクションにindex.htmlを作成します。 サーバーと、またはイベントバスと通信するには、 vertx-eventbus.jsライブラリを使用します。
日付をフォーマットするには、かなりシンプルで便利なdate-format.jsライブラリを使用します 。 さらに、htmlデザインとして、vertx-eventbus.jsライブラリとjqueryバージョン1.11.3に必要なブートストラップバージョン3.3.5、sockjs.jsバージョン0.3.4を使用します。
クライアント側イベントバスハンドラは次のとおりです。
index.html
var online = 0; // -. var eb = new EventBus("/eventbus/"); // . eb.onopen = function() { // . eb.registerHandler("chat.to.client", eventChatProcessing); }; // . function eventChatProcessing(err, msg) { var event = jQuery.parseJSON(msg.body); if (event.type == 'publish') { //. var time = Date.parse(event.time); var formattedTime = dateFormat(time, "dd.mm.yy HH:MM:ss"); // . appendMsg(event.host, event.port, event.message, formattedTime); } else { // . //type: register close. online = event.online; $('#online').text(online); } };
イベントのタイプがパブリッシュ(つまり、メッセージのパブリケーション)の場合、イベントからのデータはタプルに形成され、メッセージテーブルに添付されます。 それ以外の場合、イベントの種類が新規または退去したユーザーに対応する場合、オンラインユーザーカウンターは単に更新されます。 メッセージを追加する機能は非常に簡単です。
index.html
// . function appendMsg(host, port, message, formattedTime) { var $msg = $('<tr bgcolor="#dff0d8"><td align="left">' + formattedTime + '</td><td align="left">' + host + ' [' + port + ']' + '</td><td>' + message + '</td></tr>'); var countMsg = $('#messages tr').length; if (countMsg == 0) $('#messages').append($msg); else $('#messages > tbody > tr:first').before($msg); }
メッセージを送信するとき、最初に「chat.to.server」で公開され、サーバーがそれを処理します。メッセージが検証に合格した場合、メッセージはすべてのクライアントに送信されます。 そして送信者に。
index.html
$(document).ready(function() { // . $('#chatForm').submit(function(evt) { evt.preventDefault(); var message = $('#message').val(); if (message.length > 0) { // . eb.publish("chat.to.server", message); $('#message').val("").focus(); countChar(); } }); });
そして最後に、条件によって、入力された文字数を処理する最後の方法は、ユーザーが140文字を超えるメッセージを入力できないことです。
index.html
// . function countChar() { var len = $('#message').val().length; if (len > 140) { var msg = $('#message').val().substring(0, 140); $('#message').val(msg); } else { $('#charNum').text(140 - len); var per = 100 / 140 * len; $('#charNumProgressBar').css('width', per + '%').attr('aria-valuenow', per); } };
マークアップを含むindex.htmlの完全版は、記事の最後にあります。
サーバーとクライアントの部分を作成した後、アプリケーションを起動しました。 起動と便利なデバッグのために、独自のVerticleローダーを作成することをお勧めしますが、より簡単な代替手段があります。これについては後で説明します。
dir変数を初期化する唯一の値は関連する必要があります 実際、そのようなパスが存在する必要があります。 また、verticleID変数は、起動されたバーティクルクラスの名前で初期化する必要があります。他のすべてのコードは変更できません。
VerticleLoader.java
public class VerticleLoader { private static Vertx vertx; public static Vertx getVertx() { return vertx; } public static void load() { load(null); } public static void load(Handler<AsyncResult<String>> completionHandler) { VertxOptions options = new VertxOptions().setClustered(false); // verticle-. String dir = "chat/src/main/java/"; try { File current = new File(".").getCanonicalFile(); if (dir.startsWith(current.getName()) && !dir.equals(current.getName())) { dir = dir.substring(current.getName().length() + 1); } } catch (IOException e) { } System.setProperty("vertx.cwd", dir); String verticleID = Server.class.getName(); Consumer<Vertx> runner = vertx -> { try { if (completionHandler == null) vertx.deployVerticle(verticleID); else vertx.deployVerticle(verticleID, completionHandler); } catch (Throwable t) { t.printStackTrace(); } }; if (options.isClustered()) { Vertx.clusteredVertx(options, res -> { if (res.succeeded()) { vertx = res.result(); runner.accept(vertx); } else { res.cause().printStackTrace(); } }); } else { vertx = Vertx.vertx(options); runner.accept(vertx); } } public static void main(String[] args) { load(); } }
ブートローダーの準備ができたら、起動構成を作成します。実行-構成の編集...-新しい構成の追加(Alt + Insert)-アプリケーション。 メインクラスをVerticleLoaderとして指定し、構成を保存して実行します。
構成イメージ
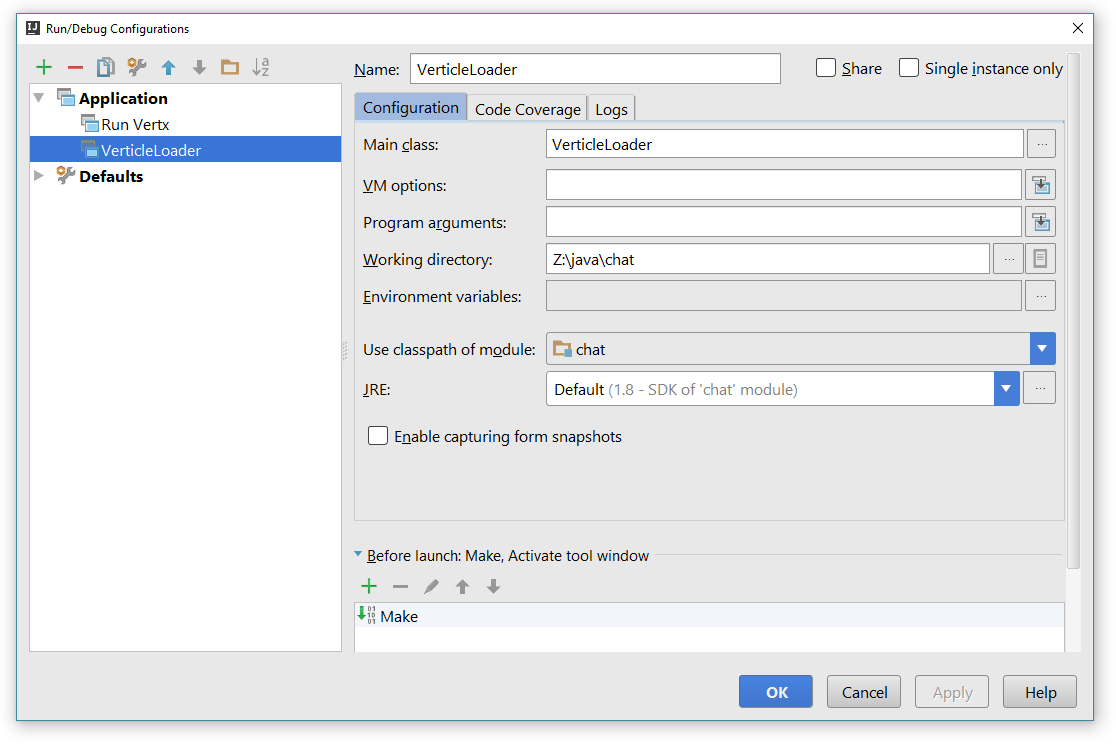
利益!
約束された代替案。
代替構成
図に示すように、起動構成を作成する必要があります。 実際、Starterクラスはメインクラスであり、アプリケーションのエントリポイントであるmainメソッドが含まれています。
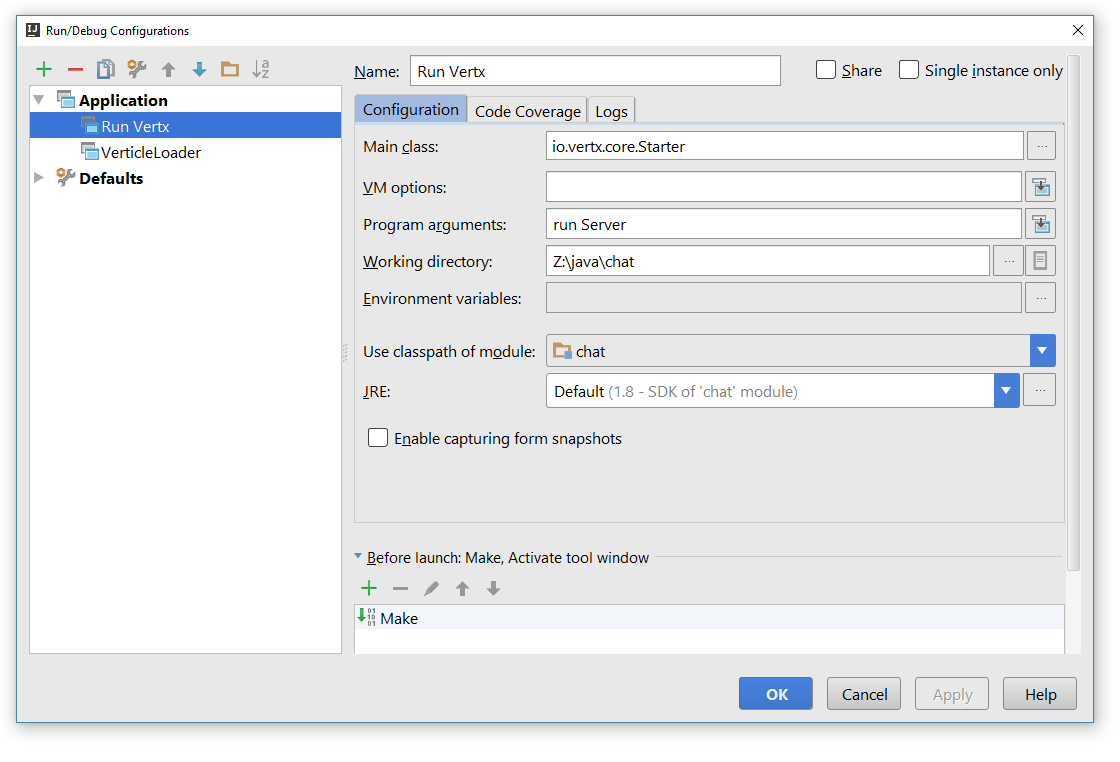
テスト中
開発したアプリケーションをテストしましょう。 JUnitを使用してこれを行うため、pom.xmlを再度開き、次の依存関係を追加する必要があります。
pom.xml
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency>
setUpでは、Vertxをインスタンス化し、Verticleをデプロイします。 従来のJUnitメソッドとは異なり、現在のすべてのメソッドは別のTestContextを取得します。 このオブジェクトのタスクは、テストの非同期性を観察することです。
TestContextオブジェクトのtearDown()メソッドでは、asyncAssertSuccess()が呼び出され、Verticleのシャットダウン中に問題があると失敗します。
ChatTest.java
@RunWith(VertxUnitRunner.class) public class ChatTest { private Vertx vertx; private int port = 8080; private Logger log = LoggerFactory.getLogger(ChatTest.class); //@Ignore @Before public void setUp(TestContext context) throws IOException { VerticleLoader.load(context.asyncAssertSuccess()); vertx = VerticleLoader.getVertx(); } //@Ignore @After public void tearDown(TestContext context) { vertx.close(context.asyncAssertSuccess()); } //... }
loadVerticleTestメソッドで、アプリケーションの読み込みを確認します。 クライアントを作成し、指定されたアドレスにデプロイされたアプリケーションが利用可能であることを確認しようとします。 成功すると、ステータスコード200を受け取ります。
次に、ページのコンテンツの取得を試みます。ページのタイトルには「チャット」というテキストを含める必要があります。
要求と応答は非同期操作であるため、テストの完了時に何らかの方法で通知を制御および受信する必要があります。これには非同期オブジェクトが使用され、常にcomplete()メソッドを呼び出してテストを完了します。
ChatTest.java
@Test public void loadVerticleTest(TestContext context) { log.info("*** loadVerticleTest ***"); Async async = context.async(); vertx.createHttpClient().getNow(port, "localhost", "/", response -> { context.assertEquals(response.statusCode(), 200); context.assertEquals(response.headers().get("content-type"), "text/html"); response.bodyHandler(body -> { context.assertTrue(body.toString().contains("<title>Chat</title>")); async.complete(); }); }); }
eventBusTestメソッドでは、イベントバスクライアントが作成され、ハンドラーがハングします。 クライアントがバス上のイベントを待っている間、メッセージが発行されます。 ハンドラーはこれに応答し、着信イベントの本文の等価性をチェックします;検証が成功した場合、テストはasync.complete()の呼び出しで終了します。
ChatTest.java
@Test public void eventBusTest(TestContext context) { log.info("*** eventBusTest ***"); Async async = context.async(); EventBus eb = vertx.eventBus(); eb.consumer("chat.to.server").handler(message -> { String getMsg = message.body().toString(); context.assertEquals(getMsg, "hello"); async.complete(); }); eb.publish("chat.to.server", "hello"); }
テストを実行します。
方法を見る...
タブMavenプロジェクト-ライフサイクル-テスト-実行[テスト]。
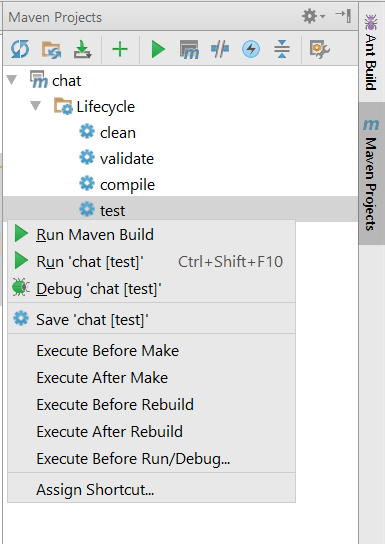
実行可能モジュールをビルドして実行する
これを行うには、pom.xmlにmaven-shade-pluginプラグインを追加します。 私たちの場合、Main-VerticleがServerクラスを指す場所。
pom.xml
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <version>2.3</version> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> <configuration> <transformers> <transformer implementation="org.apache.maven.plugins.shade.resource.ManifestResourceTransformer"> <manifestEntries> <Main-Class>io.vertx.core.Starter</Main-Class> <Main-Verticle>Server</Main-Verticle> </manifestEntries> </transformer> </transformers> <artifactSet/> <outputFile>${project.build.directory}/${project.artifactId}-${project.version}-fat.jar</outputFile> </configuration> </execution> </executions> </plugin>
Run Maven Buildコマンドを実行すると、chat-1.0-fat.jarがターゲットディレクトリに表示されます。 アプリケーションを実行するには、実行可能モジュールとwebrootフォルダーが同じディレクトリにある必要があります。 ポート12345でアプリケーションをデプロイするには、次のコマンドを実行する必要があります。
java -jar chat-1.0-fat.jar 12345
それだけです 頑張って!
完全なソースコード
Server.java
import com.google.gson.Gson; import io.vertx.core.AbstractVerticle; import io.vertx.core.Starter; import io.vertx.core.logging.Logger; import io.vertx.core.logging.LoggerFactory; import io.vertx.ext.web.Router; import io.vertx.ext.web.handler.StaticHandler; import io.vertx.ext.web.handler.sockjs.BridgeEvent; import io.vertx.ext.web.handler.sockjs.BridgeOptions; import io.vertx.ext.web.handler.sockjs.PermittedOptions; import io.vertx.ext.web.handler.sockjs.SockJSHandler; import java.io.IOException; import java.net.BindException; import java.net.InetAddress; import java.net.ServerSocket; import java.net.UnknownHostException; import java.util.*; import java.util.concurrent.atomic.AtomicInteger; import static io.vertx.ext.web.handler.sockjs.BridgeEvent.Type.*; public class Server extends AbstractVerticle { private Logger log = LoggerFactory.getLogger(Server.class); private SockJSHandler handler = null; private AtomicInteger online = new AtomicInteger(0); // . @Override public void start() throws Exception { if (!deploy()) { log.error("Failed to deploy the server."); return; } handle(); } // . private boolean deploy() { int hostPort = getFreePort(); if (hostPort < 0) return false; Router router = Router.router(vertx); // . handler = SockJSHandler.create(vertx); router.route("/eventbus/*").handler(handler); router.route().handler(StaticHandler.create()); // -. vertx.createHttpServer().requestHandler(router::accept).listen(hostPort); try { String addr = InetAddress.getLocalHost().getHostAddress(); log.info("Access to \"CHAT\" at the following address: \nhttp://" + addr + ":" + hostPort); } catch (UnknownHostException e) { log.error("Failed to get the local address: [" + e.toString() + "]"); return false; } return true; } // . private int getFreePort() { int hostPort = 8080; // , // . if (Starter.PROCESS_ARGS != null && Starter.PROCESS_ARGS.size() > 0) { try { hostPort = Integer.valueOf(Starter.PROCESS_ARGS.get(0)); } catch (NumberFormatException e) { log.warn("Invalid port: [" + Starter.PROCESS_ARGS.get(0) + "]"); } } // . if (hostPort < 0 || hostPort > 65535) hostPort = 8080; return getFreePort(hostPort); } // 0, // . private int getFreePort(int hostPort) { try { ServerSocket socket = new ServerSocket(hostPort); int port = socket.getLocalPort(); socket.close(); return port; } catch (BindException e) { //, . if (hostPort != 0) return getFreePort(0); log.error("Failed to get the free port: [" + e.toString() + "]"); return -1; } catch (IOException e) { log.error("Failed to get the free port: [" + e.toString() + "]"); return -1; } } private void handle() { BridgeOptions opts = new BridgeOptions() .addInboundPermitted(new PermittedOptions().setAddress("chat.to.server")) .addOutboundPermitted(new PermittedOptions().setAddress("chat.to.client")); // . handler.bridge(opts, event -> { if (event.type() == PUBLISH) publishEvent(event); if (event.type() == REGISTER) registerEvent(event); if (event.type() == SOCKET_CLOSED) closeEvent(event); // , // . event.complete(true); }); } // - . private boolean publishEvent(BridgeEvent event) { if (event.rawMessage() != null && event.rawMessage().getString("address").equals("chat.to.server")) { String message = event.rawMessage().getString("body"); if (!verifyMessage(message)) return false; String host = event.socket().remoteAddress().host(); int port = event.socket().remoteAddress().port(); Map<String, Object> publicNotice = createPublicNotice(host, port, message); vertx.eventBus().publish("chat.to.client", new Gson().toJson(publicNotice)); return true; } else return false; } // . private Map<String, Object> createPublicNotice(String host, int port, String message) { Date time = Calendar.getInstance().getTime(); Map<String, Object> notice = new TreeMap<>(); notice.put("type", "publish"); notice.put("time", time.toString()); notice.put("host", host); notice.put("port", port); notice.put("message", message); return notice; } // - . private void registerEvent(BridgeEvent event) { if (event.rawMessage() != null && event.rawMessage().getString("address").equals("chat.to.client")) new Thread(() -> { Map<String, Object> registerNotice = createRegisterNotice(); vertx.eventBus().publish("chat.to.client", new Gson().toJson(registerNotice)); }).start(); } // . private Map<String, Object> createRegisterNotice() { Map<String, Object> notice = new TreeMap<>(); notice.put("type", "register"); notice.put("online", online.incrementAndGet()); return notice; } // - . private void closeEvent(BridgeEvent event) { new Thread(() -> { Map<String, Object> closeNotice = createCloseNotice(); vertx.eventBus().publish("chat.to.client", new Gson().toJson(closeNotice)); }).start(); } // . private Map<String, Object> createCloseNotice() { Map<String, Object> notice = new TreeMap<>(); notice.put("type", "close"); notice.put("online", online.decrementAndGet()); return notice; } // , // , // ;) private boolean verifyMessage(String msg) { return msg.length() > 0 && msg.length() <= 140; } }
VerticleLoader.java
import io.vertx.core.AsyncResult; import io.vertx.core.Handler; import io.vertx.core.Vertx; import io.vertx.core.VertxOptions; import io.vertx.core.impl.StringEscapeUtils; import java.io.File; import java.io.IOException; import java.util.function.Consumer; public class VerticleLoader { private static Vertx vertx; public static Vertx getVertx() { return vertx; } public static void load() { load(null); } public static void load(Handler<AsyncResult<String>> completionHandler) { VertxOptions options = new VertxOptions().setClustered(false); // verticle-. String dir = "chat/src/main/java/"; try { File current = new File(".").getCanonicalFile(); if (dir.startsWith(current.getName()) && !dir.equals(current.getName())) { dir = dir.substring(current.getName().length() + 1); } } catch (IOException e) { } System.setProperty("vertx.cwd", dir); String verticleID = Server.class.getName(); Consumer<Vertx> runner = vertx -> { try { if (completionHandler == null) vertx.deployVerticle(verticleID); else vertx.deployVerticle(verticleID, completionHandler); } catch (Throwable t) { t.printStackTrace(); } }; if (options.isClustered()) { Vertx.clusteredVertx(options, res -> { if (res.succeeded()) { vertx = res.result(); runner.accept(vertx); } else { res.cause().printStackTrace(); } }); } else { vertx = Vertx.vertx(options); runner.accept(vertx); } } public static void main(String[] args) { load(); } }
ChatTest.java
import io.vertx.core.Vertx; import io.vertx.core.eventbus.EventBus; import io.vertx.core.logging.Logger; import io.vertx.core.logging.LoggerFactory; import io.vertx.ext.unit.Async; import io.vertx.ext.unit.TestContext; import io.vertx.ext.unit.junit.VertxUnitRunner; import org.junit.After; import org.junit.Before; import org.junit.Test; import org.junit.runner.RunWith; import java.io.IOException; @RunWith(VertxUnitRunner.class) public class ChatTest { private Vertx vertx; private int port = 8080; private Logger log = LoggerFactory.getLogger(ChatTest.class); //@Ignore @Before public void setUp(TestContext context) throws IOException { // Verticle. VerticleLoader.load(context.asyncAssertSuccess()); vertx = VerticleLoader.getVertx(); } //@Ignore @After public void tearDown(TestContext context) { vertx.close(context.asyncAssertSuccess()); } //@Ignore @Test public void loadVerticleTest(TestContext context) { log.info("*** loadVerticleTest ***"); Async async = context.async(); vertx.createHttpClient().getNow(port, "localhost", "/", response -> { // . context.assertEquals(response.statusCode(), 200); context.assertEquals(response.headers().get("content-type"), "text/html"); // . response.bodyHandler(body -> { context.assertTrue(body.toString().contains("<title>Chat</title>")); async.complete(); }); }); } //@Ignore @Test public void eventBusTest(TestContext context) { log.info("*** eventBusTest ***"); Async async = context.async(); EventBus eb = vertx.eventBus(); // . eb.consumer("chat.to.server").handler(message -> { String getMsg = message.body().toString(); context.assertEquals(getMsg, "hello"); async.complete(); }); // . eb.publish("chat.to.server", "hello"); } }
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Chat</title> <meta charset="windows-1251"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <script src="//cdn.jsdelivr.net/sockjs/0.3.4/sockjs.min.js"></script> <link rel="stylesheet" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script> <script src="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/js/bootstrap.min.js"></script> <script src="date-format.js"></script> <script src="vertx-eventbus.js"></script> <style type="text/css"> body { padding-top: 40px; padding-bottom: 40px; background-color: #f5f5f5; } .received{ width: 160px; font-size: 10px; } input[type=text]:focus, textarea:focus{ box-shadow: 0 0 5px #4cae4c; border: 1px solid #4cae4c; } .tab-content{ padding:5px } </style> <script> var online = 0; // -. var eb = new EventBus("/eventbus/"); // . eb.onopen = function() { // . eb.registerHandler("chat.to.client", eventChatProcessing); }; // . function eventChatProcessing(err, msg) { var event = jQuery.parseJSON(msg.body); if (event.type == 'publish') {//. var time = Date.parse(event.time); var formattedTime = dateFormat(time, "dd.mm.yy HH:MM:ss"); // . appendMsg(event.host, event.port, event.message, formattedTime); } else { // . //type: register close. online = event.online; $('#online').text(online); } }; // . function appendMsg(host, port, message, formattedTime){ var $msg = $('<tr bgcolor="#dff0d8"><td align="left">' + formattedTime + '</td><td align="left">' + host + ' [' + port + ']' + '</td><td>' + message + '</td></tr>'); var countMsg = $('#messages tr').length; if (countMsg == 0) $('#messages').append($msg); else $('#messages > tbody > tr:first').before($msg); } $(document).ready(function() { // . $('#chatForm').submit(function(evt) { evt.preventDefault(); var message = $('#message').val(); if (message.length > 0) { // . eb.publish("chat.to.server", message); $('#message').val("").focus(); countChar(); } }); }); // . function countChar() { var len = $('#message').val().length; if (len > 140) { var msg = $('#message').val().substring(0, 140); $('#message').val(msg); } else { $('#charNum').text(140 - len); var per = 100 / 140 * len; $('#charNumProgressBar').css('width', per+'%').attr('aria-valuenow', per); } }; </script> </head> <body> <div class="container chat-wrapper"> <form id="chatForm"> <h2 align="center" class="alert alert-success">CHAT ROOM</h2> <fieldset> <div class="input-group input-group-lg"> <span class="input-group-addon" id="onlineIco"> <span class="glyphicon glyphicon-eye-open"></span> </span> <span class="input-group-addon" id="online"> <span class="glyphicon glyphicon-option-horizontal"></span> </span> <input type="text" maxlength="141" autocomplete="off" class="form-control" placeholder="What's new?" id="message" aria-describedby="sizing-addon1" onkeyup="countChar()"/> <span class="input-group-btn"> <button class="btn btn-success" type="submit"> <span class="glyphicon glyphicon-send"></span> </button> </span> </div> </fieldset> <h3 id="charNum">140</h3> <div class="progress"> <div id="charNumProgressBar" class="progress-bar progress-bar-success active" role="progressbar" aria-valuenow="0" aria-valuemin="0" aria-valuemax="100" style="width: 0%"> <span class="sr-only">100% Complete</span> </div> </div> <div class="panel panel-success"> <div class="panel-heading"><h3>New messages</h3></div> <table id="messages" class="table table-hover" width="100%"> <colgroup> <col style="width:10%"> <col style="width:10%"> <col style="width:10%"> </colgroup> </table> </div> </form> </div> </body> </html>