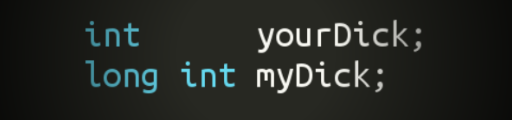
この種の記事はすでにいくつかあり、Unityのさまざまなトリックやトリックについて説明しています。 何かが非常に明白であり、初心者のみ、より高度な同志にとってのみでした。 ささやかな貢献をしたいです。
1.変数に関するヒント。
プロジェクトが小さい場合、これは関係ありませんが、プロジェクトが大きく、多くの人が作業している場合、エディターで設定したこの変数またはその変数が何を担当しているかを忘れることができます。 適切な命名は、短すぎるため、部分的にしか役立ちません。 確かにカスタムエディタを記述でき、そこで各タイプのヒントを作成できますが、各クラスでは高すぎます。 次の方法により、このような問題を解決できます。 これを行うために、2つのクラシックを作成します。
TooltipAttribute.cs
using UnityEngine; public class TooltipAttribute : PropertyAttribute { public readonly string text; public TooltipAttribute(string text) { this.text = text; } }
TooltipDrawer.cs
#if UNITY_EDITOR using UnityEditor; using UnityEngine; [CustomPropertyDrawer(typeof(TooltipAttribute))] public class TooltipDrawer : PropertyDrawer { public override void OnGUI(Rect position, SerializedProperty prop, GUIContent label) { var atr = (TooltipAttribute) attribute; var content = new GUIContent(label.text, atr.text); EditorGUI.PropertyField(position, prop, content); } } #endif
ここで、必要な変数の標準インスペクターにヒントが必要な場合は、次のようにします。
[Tooltip(" ")] public Color color; [Tooltip(" , - ")] public float speed;
結果:

スクリーンショットでは、何らかの理由で、マウスカーソルが表示されず、ホバーにヒントが表示されます。
完全主義者は、リリースコードにゴミを追加しないために、これを行うことができます。
#if UNITY_EDITOR [Tooltip(" , - ")] #endif public float speed;
私の意見では、これはすでに不要です。
これで、1〜2年でエディターで何かを修正する必要がある場合でも、それがどのような変数であるかをすぐに覚えることができます。
こちらがサムライPropertyDrawerコレクションです。 コメントは日本語ですが、基本的にはすべてが明確です。
2. Nullable型
変数に値があるかどうかを確認する必要がある場合があります。 さて、例えば、このように:
public class Character : MonoBehaviour { Vector3 targetPosition; void MoveTowardsTargetPosition() { if(targetPosition != Vector3.zero) { //Move towards the target position! } } public void SetTargetPosition(Vector3 newPosition) { targetPosition = newPosition; } }
しかし、ヒーローがポイント(0、0、0)に到達する必要がある場合はどうでしょうか? その後、このコードは機能しません。
nullable型を使用する必要があります。 「?」を追加するだけです 型の最後で、可用性を確認するにはHasValueを使用し、値を取得するにはValueを使用します。
public class Character : MonoBehaviour { //Notice the added "?" Vector3? targetPosition; void MoveTowardsTargetPosition() { if (targetPosition.HasValue) { //Move towards the target position! //use targetPosition.Value for the actual value } } public void SetTargetPosition(Vector3 newPosition) { targetPosition = newPosition; } }
3.個人ログ。
このヒントは、少なくとも私の場合はAIのデバッグに役立ちます。 ヒントの意味は非常に簡単です-巨大な一般的なログを掘らないように、各ユニットを個人用にします。この場合は文字列(文字列localLog)です。 私たちはそこにモンスターの生涯からのすべての重要なイベントを書きます、そしてあなたが見るためにただエディタでモンスターを選択する必要があります。 インスペクターに個人ログを表示するためのコード:
using UnityEngine; using System.Collections; using UnityEditor; [CustomEditor(typeof(Monster))] public class MonsterEditor : Editor { Vector2 scrollPos = new Vector2(0, Mathf.Infinity); public override void OnInspectorGUI() { serializedObject.Update(); Monster monster = (Monster)target; if (Application.isPlaying) { scrollPos = GUILayout.BeginScrollView ( scrollPos, GUILayout.Height (250)); GUILayout.Label (monster.localLog); GUILayout.EndScrollView (); if (GUILayout.Button ("Clear")) monster.localLog = ""; } serializedObject.ApplyModifiedProperties(); DrawDefaultInspector(); } }
それは、一般に、すべてです。 今、私たちは、モンスターが私たちや他のモンスターについて考えたすべてを見ることができます。 このような単純なヒントにより、デバッグが容易になります。
これが誰かを助けてくれたら嬉しいです。