はじめに
WPFでの階層構造(ネストの任意のレベル)の形式でのデータセットの表現は非常に簡単です。 原則として、これにはSystem.Windows.Controls.TreeViewクラスが使用され、結果は次のようになります。

このようなツリーを構築する2つのケースを示します。これらは、 データソースによって異なります。
- MS SQL Server 2008でホストされるデータベース
- XMLファイル。
アプリケーションは、あるデータソースを別のデータソースに動的に置き換える機能を実装します。
まず、情報を取得するデータソースを作成する必要があります。 これらのソースは次のとおりです。
- MyTestDb.mdfデータベース
- XML xmlfile1.xml
2つのプロジェクト(プロジェクト)で構成される単一のソリューションが作成されます。
- Linq2SqlProjectは、リレーショナルデータベース構造のオブジェクト投影であるクラスのセットを含むライブラリです。
- WpfGuiProject -WPFテクノロジーを使用して作成されたアプリケーションのグラフィカル部分(GUI)。 同じプロジェクトで、XMLデータの操作に関するコードが投稿されます。
このトピックで説明されているすべてのデータソースとの相互作用は、LINQテクノロジを使用して実装されます。
1.データベースの作成
この例では、データベースは2つのテーブルで構成されます-これは、前述の問題の解決策を示すのに十分です。

MySchema.Categoriesテーブルに次のエントリを追加しました。
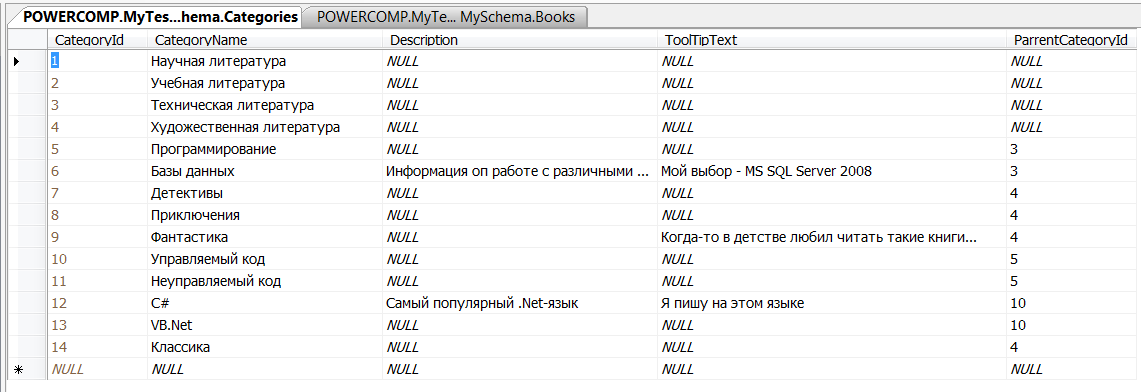
次のデータがMySchema.Booksテーブルに入力されます。

例を複雑にしないために、クライアントアプリケーションがデータベースと連携するデータベースに一連のストアドプロシージャを作成しません(実際の作業ではもちろん、実行の可能性を避けるために、ストアドプロシージャを通じてデータベースと対話するだけですSQLインジェクション)。
ここから上記のデータベースをダウンロードし、DBMSに接続します。
2.オブジェクト指向のデータベースプロジェクションを作成する
1.新しい空のソリューション(ソリューション)を作成し、「TreeStructureBrowse」という名前を割り当てます。
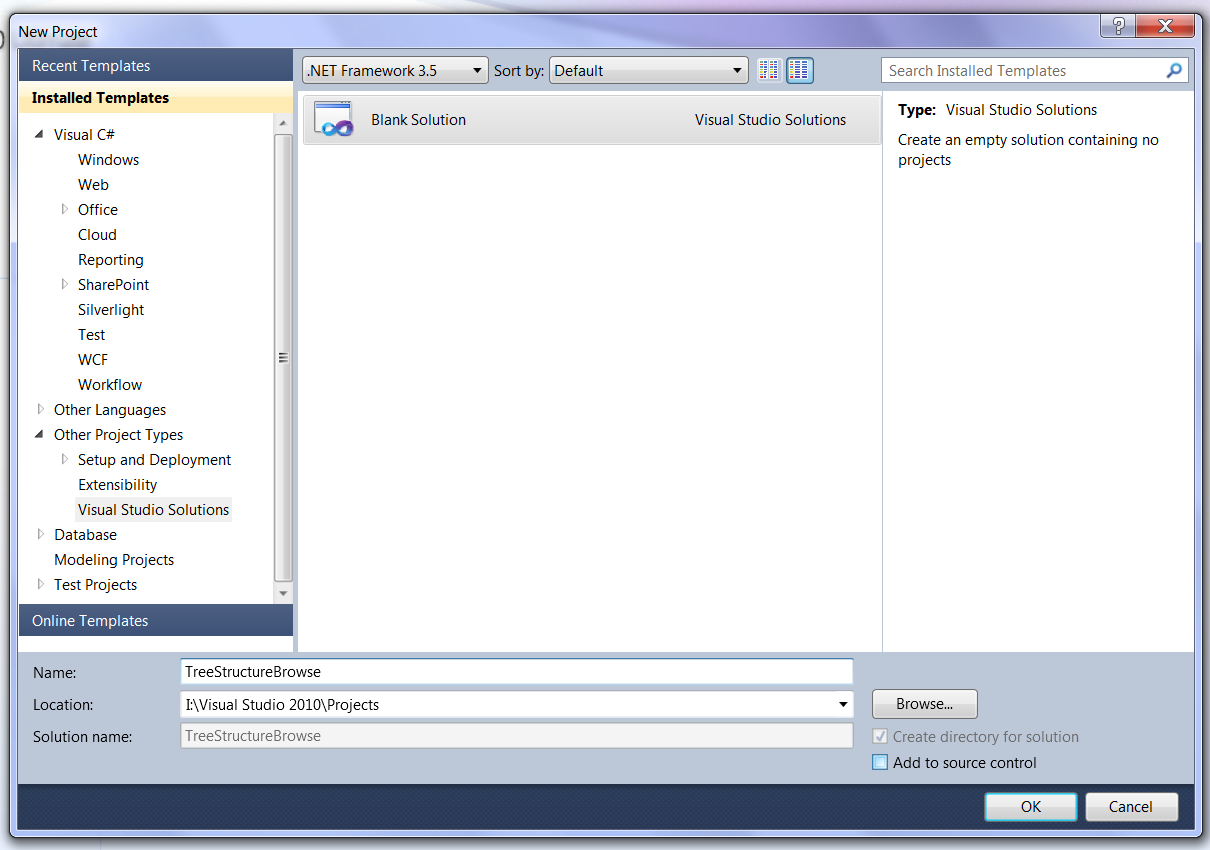
2.ソリューションエクスプローラーでソリューションの名前をクリックして取得したコンテキストメニューから、[追加] => [新しいプロジェクト...]を選択します。
3.プロジェクトタイプ「クラスライブラリ」を選択し、プロジェクトに「Linq2Sql」という名前を割り当てて、OKキーを押します。
4.手順3で追加したプロジェクトの名前をクリックして取得したコンテキストメニューから、[追加] => [新しいアイテム]を選択します...
5.要素「LINQ to SQL Classes」を選択し、ファイル名「MyTestDb」を割り当てます。
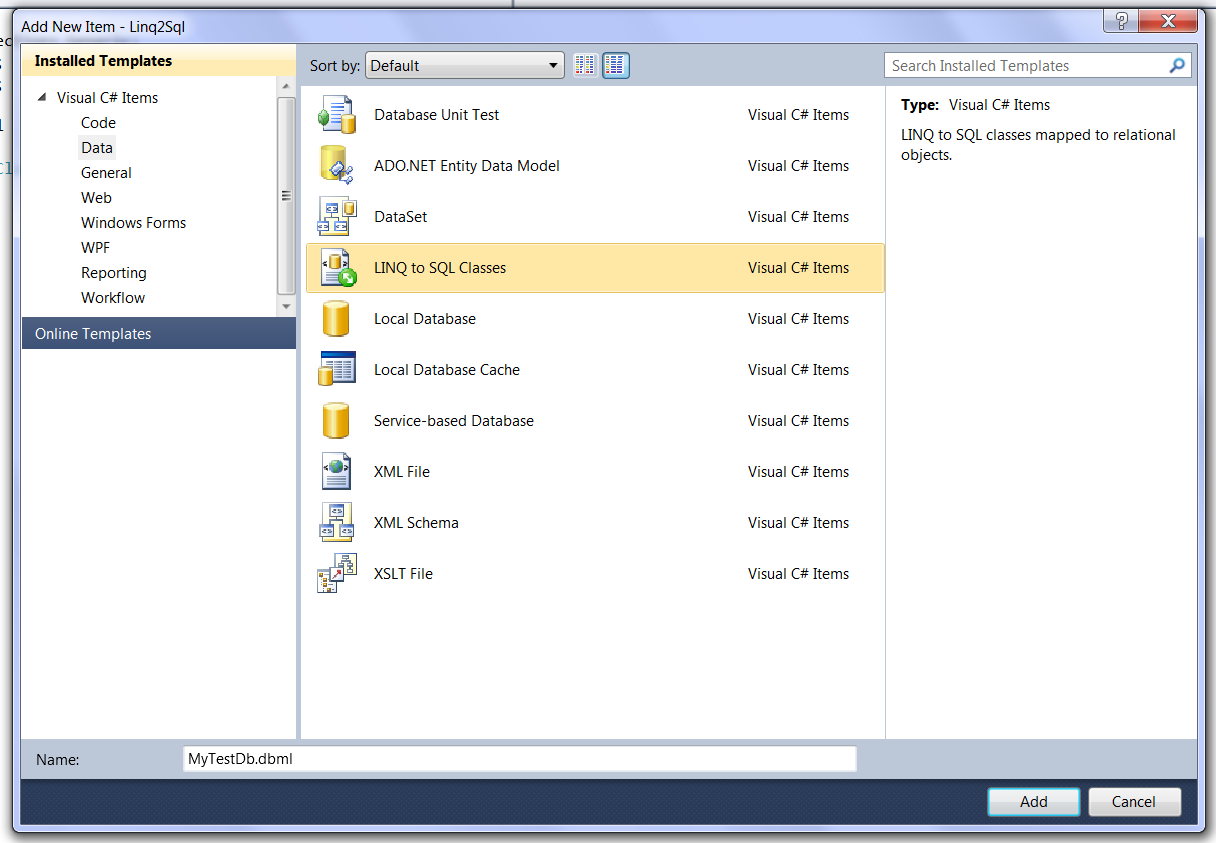
6. [表示]メニューから[サーバーエクスプローラー]を選択します。 表示されるウィンドウで、[データベースに接続]ボタンをクリックします。

7.接続文字列に必要なすべてのパラメーターを指定します(私の場合、Windows認証が使用されます)。

8. [接続テスト]ボタンをクリックして接続できることを確認し、[OK]ボタンをクリックして[接続の追加]ウィンドウを閉じます。
9. [サーバーエクスプローラー]ウィンドウに次の図が表示されます。

ps powercompは私のコンピューター名です
10. Shiftキーを押しながら、両方のテーブル(ブックとカテゴリ)を選択し、マウスでそれらをMyTestDb.dbml *タブにドラッグすると、次の図が表示されます。

11.プロジェクトを保存し、Build => Rebuild Solutionコマンドを実行します。
T.O. リレーショナルベースのオブジェクト指向モデルを作成しました。これは、問題を解決する際の最初のキーポイント(3つのうち)です。
3.グラフィカルパーツ(GUI)の作成、コンバーターの作成、テンプレートの作成
1.前のセクションで作成したソリューションでは、WpfGuiProjectという名前で設定して新しいプロジェクトを追加します。

2.ソリューションに追加したプロジェクトの名前を右クリックして表示されるコンテキストメニューから、「スタートアッププロジェクトとして設定」項目を選択し、GUIプロジェクトをデフォルトで実行します。
3. Linq2SqlプロジェクトへのリンクをWpfGuiProjectプロジェクトに追加します。

4.プロジェクトにリソースディクショナリを追加します。プロジェクトの名前を右クリックして呼び出されるコンテキストメニューから、[追加] => [リソースディクショナリ...]を選択し、表示されるダイアログボックスで同じ名前の要素を選択します。

5.プロジェクトのコンテキストメニューから、「クラス」タイプの新しいファイルをプロジェクトに追加し、「MyConverters.cs」という名前を割り当てます。
6.アセンブリ「System.Data.Linq」をプロジェクトに接続します。
7. 5項で作成したファイルに、次のコードを記述します。
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
using System; using System.Collections. Generic ; using System.Linq; using System.Text; using System.Windows.Data; using Linq2Sql; using System.Xml.Linq; namespace WpfGuiProject { // Category. - Category. public sealed class CategoryValueConverter : IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is Category) { Category x = (Category) value ; return (x).Categories.Where(n => n.ParrentCategoryId == x.CategoryId).OrderBy(n => n.CategoryName); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } // XElement. - XElement, // Category. public sealed class XmlConverter :IValueConverter { public object Convert ( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { if ( value is XElement ) { XElement x = ( XElement ) value ; return x.Elements( "Category" ); } else { return null ; } } public object ConvertBack( object value , Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } } } * This source code was highlighted with Source Code Highlighter .
節7で作成されたものは、私たちの前にある課題を解決するための2番目(3つのうち)の重要なポイントです-データバインディングを使用して実装された情報の階層視覚表現 コードでは、必要な子を取得するためのロジックを決定します。これは、階層モデルの構築に参加する必要があります。
8.「Dictionary1.xaml」ファイルを編集します。
*このソースコードは、 ソースコードハイライターで強調表示されました。
- < ResourceDictionary xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
- xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
- xmlns:linq2Xml = "clr-namespace:System.Xml.Linq; assembly = System.Xml.Linq"
- xmlns:linq = "clr-namespace:Linq2Sql; assembly = Linq2Sql"
- xmlns:local = "clr-namespace:WpfGuiProject" >
- <!-データベースに接続する場合のTreeTableクラスのインスタンスの階層表示用テンプレート->
- < HierarchicalDataTemplate x:Key = "key1" DataType = "{x:Type linq:Category}" >
- <!-データソースを示し、それに基づいてパーティションツリーを形成する必要があります->
- < HierarchicalDataTemplate.ItemsSource >
- < バインディング パス = "。" >
- <!-これに関連して、カテゴリタイプの子要素のリストを取得できるコンバータを示します->
- < Binding.Converter >
- < ローカル:CategoryValueConverter />
- </ Binding.Converter >
- </ バインディング >
- </ HierarchicalDataTemplate.ItemsSource >
- <!-セクションツリーに表示される要素を視覚的に表現します->
- < DockPanel >
- < TextBlock Text = "{Binding Path = CategoryName}" >
- < TextBlock.ToolTip >
- < バインディング パス = "説明" />
- </ TextBlock.ToolTip >
- </ TextBlock >
- <!-セクションに直接配置された本の数->
- < TextBlock Name = "txtLeft" Text = "( " Grid。Column = "1" />
- < TextBlock Name = "txtCount" Text = "{Binding Path = Books.Count}" Grid 。 列 = "2" />
- < TextBlock Name = "txtRight" Text = ")" Grid 。 列 = "3" />
- </ DockPanel >
- </ HierarchicalDataTemplate >
- <!-データベースに接続する場合にBookクラスのインスタンスを表示するためのテンプレート->
- < DataTemplate DataType = "{x:タイプlinq:Book}" >
- < TextBlock Text = "{Binding Path = BookName}" >
- < TextBlock.ToolTip >
- < バインディング パス = "ToolTipText" />
- </ TextBlock.ToolTip >
- </ TextBlock >
- </ DataTemplate >
- <!-XMLファイルに接続する場合の階層データ表示用のテンプレート->
- < HierarchicalDataTemplate x:Key = "key2" DataType = "{x:タイプlinq2Xml:XElement}" >
- <!-データソースを示し、それに基づいてパーティションツリーを形成する必要があります->
- < HierarchicalDataTemplate.ItemsSource >
- < バインディング パス = "。" >
- <!-これに関連して、カテゴリタイプの子要素のリストを取得できるコンバータを示します->
- < Binding.Converter >
- < ローカル:XmlConverter />
- </ Binding.Converter >
- </ バインディング >
- </ HierarchicalDataTemplate.ItemsSource >
- <!-セクションツリーに表示される要素を視覚的に表現します->
- < TextBlock Text = "{Binding Path = Attribute [CategoryName] .Value}" >
- < TextBlock.ToolTip >
- < バインディング パス = "属性[ToolTipText] .Value" />
- </ TextBlock.ToolTip >
- </ TextBlock >
- </ HierarchicalDataTemplate >
- <!-XMLファイルへの接続の場合にBookクラスのインスタンスを表示するためのテンプレート->
- < DataTemplate x:Key = "key3" DataType = "{x:タイプlinq2Xml:XElement}" >
- < グリッド >
- < Grid.RowDefinitions >
- < RowDefinition />
- < RowDefinition Height = "Auto" />
- </ Grid.RowDefinitions >
- < TextBlock Text = "{Binding Path = Attribute [BookName] .Value}" >
- < TextBlock.ToolTip >
- < バインディング パス = "属性[ToolTipText] .Value" />
- </ TextBlock.ToolTip >
- </ TextBlock >
- </ グリッド >
- </ DataTemplate >
- </ ResourceDictionary >
節8で作成されたxmlマークアップは3番目の重要なポイントです。 このマークアップでは、HierarchicalDataTemplateクラスを使用して、現在のテンプレートとの関係で子を取得するために使用するデータ表示テンプレートとコンバーターを定義しました。
次に、ウィンドウのグラフィカルな表現を形成し始めます。
9.「MainWindow.xaml」ファイルの内容を編集します。
*このソースコードは、 ソースコードハイライターで強調表示されました。
- < ウィンドウ x:クラス = "WpfGuiProject.MainWindow"
- xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
- xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
- xmlns:linq = "clr-namespace:Linq2Sql; assembly = Linq2Sql"
- xmlns:local = "clr-namespace:WpfGuiProject"
- Title = "バインディングを使用したデータの階層表示" Height = "350" Width = "525" >
- <!-リソースファイルを含める->
- < Window.Resources >
- < ResourceDictionary >
- < ResourceDictionary.MergedDictionaries >
- < ResourceDictionary Source = "Dictionary1.xaml" />
- </ ResourceDictionary.MergedDictionaries >
- </ ResourceDictionary >
- </ Window.Resources >
- <!-ウィンドウの視覚的なコンテンツを形成する->
- < グリッド >
- < Grid.ColumnDefinitions >
- < ColumnDefinition Width = "50 *" />
- < ColumnDefinition Width = "Auto" />
- < ColumnDefinition Width = "50 *" />
- </ Grid.ColumnDefinitions >
- < Grid.RowDefinitions >
- < RowDefinition Height = "Auto" />
- < RowDefinition Height = "100 *" />
- < RowDefinition Height = "Auto" />
- </ Grid.RowDefinitions >
- <!-ツリー構造を表示します->
- < TreeView Name = "treeStructure" Margin = "2" Grid 。 行 = "1" />
- <!-階層内の選択した要素のメモを表示します->
- < GroupBox Header = "Note" Margin = "2" Grid 。 行 = "2" >
- < TextBlock Name = "selectedNodeDescription" TextWrapping = "Wrap" Text = "{Binding ElementName = treeStructure、Path = SelectedItem.Description}" />
- </ GroupBox >
- < GridSplitter Width = "5" HorizontalAlignment = "Center" VerticalAlignment = "Stretch" Grid 。 列 = "1" グリッド 。 RowSpan = "3" />
- < ListBox Name = "listBooks" Margin = "2" Grid 。 列 = "2" グリッド 。 RowSpan = "2" ItemsSource = "{Binding ElementName = treeStructure、Path = SelectedItem.Books}" />
- < GroupBox Header = "Note" Margin = "2" Grid 。 列 = "2" グリッド 。 行 = "2" >
- < TextBlock Name = "selectedBookDescription" TextWrapping = "Wrap" Text = "{Binding ElementName = listBooks、Path = SelectedItem.Description}" />
- </ GroupBox >
- <!-対象のデータソースの選択をユーザーに提供します。 このブロックは、定義されているXAMLマークアップの後に配置する必要があります
- treeStructure要素。 この要件は、RadioButton要素のXAMLマークアップに登録が含まれているという事実によるものです。
- 本体には、treeStructure要素を使用するコードがあり、XAMLドキュメントの規則に従って、
- 決定される前->
- < GroupBox Header = "Data Source" Margin = "2" >
- < StackPanel Margin = "2" >
- < RadioButton 名 = "rbDatabase" コンテンツ = "MS SQL Server データベース " IsChecked = "True" Checked = "Change_DataSource" />
- < RadioButton Name = "rbXmlFile" Content = "XML file" IsChecked = "False" Checked = "Change_DataSource" />
- </ stackpanel >
- </ GroupBox >
- </ グリッド >
- </ ウィンドウ >
このマークアップはそのようなウィンドウを形成します:

10.ファイル「XMLFile1.xml」をプロジェクトに追加します。これは代替データソースになります。

作成したファイルに、xml形式で記述されたデータを入力します。
*このソースコードは、 ソースコードハイライターで強調表示されました。
- <? xml バージョン = "1.0" encoding = "utf-8" ? >
- < MyXmlDocument >
- < カテゴリ CategoryName = "コンピュータ文学" 説明 = "さまざまな コンピュータ文学 " ToolTipText = "マイセクション" >
- < カテゴリ CategoryName = "Windowsアプリケーション" Description = "このOSは最も人気があります" ToolTipText = "My axis" >
- < カテゴリ CategoryName = "MS Office 2007" Description = "Office Suite" ToolTipText = "My Office" />
- < カテゴリ CategoryName = "Autodesk Products" Description = "Various CAD" ToolTipText = "My Choice" >
- < カテゴリ CategoryName = "AutoCAD" Description = "最も一般的なCAD" ToolTipText = "My CAD" >
- < Book BookName = "AutoCAD 2010. Poleschuk N.N." 説明 =「このCADに関する私の本」 ToolTipText =「参照」 />
- < Book BookName = "AutoCAD 2007、ユーザーの聖書。(著者は覚えていません)" Description = "このCADシステムの別の 本 " ToolTipText = "古い本" />
- </ カテゴリー >
- < カテゴリ CategoryName = "Revit" Description = "新世代のCAD(BIM)" ToolTipText = "まだCADになっていない" />
- </ カテゴリー >
- </ カテゴリー >
- < カテゴリ CategoryName = "Linuxアプリケーション" Description = " フリーウェア OS" ToolTipText = "興味深いが、あまり一般的ではない" />
- </ カテゴリー >
- < カテゴリ CategoryName = "Classics" Description = "Classical Literature" ToolTipText = "一般的な開発に有用" >
- < Category CategoryName = "Tales and Stories" Description = "ロシアの著者による詩" ToolTipText = "For the soul" >
- < Book BookName = "Captain's daughter。A.S. Pushkin" Description = "School course" ToolTipText = "一度読んだ..." />
- </ カテゴリー >
- < カテゴリ CategoryName = "Poetry" Description = "国内作家の物語と物語" ToolTipText = "これも魂のためです" />
- </ カテゴリー >
- </ MyXmlDocument >
11.ファイル「MainWindow.xaml.cs」に変更を加えます。
*このソースコードは、 ソースコードハイライターで強調表示されました。
- システムを使用して ;
- System.Collections を使用します。 ジェネリック
- System.Linq を使用します。
- using System.Text;
- System.Windows を使用します。
- System.Windows.Controls を使用します。
- using System.Windows.Data;
- System.Windows.Documents を使用します。
- System.Windows.Input を使用します。
- System.Windows.Media を使用します。
- System.Windows.Media.Imaging を使用します。
- System.Windows.Navigation を使用します。
- System.Windows.Shapes を使用します。
- //必要な名前空間へのリンクを追加します
- Linq2Sql を使用 。
- System.Xml.Linq を使用します。
- 名前空間 WpfGuiProject
- {
- /// <summary>
- /// MainWindow.xamlの相互作用ロジック
- /// </ summary>
- パブリック 部分 クラス MainWindow:Window
- {
- XElement xml;
- パブリック MainWindow()
- {
- InitializeComponent();
- xml = XElement .Load( @ ".. \ .. \ XMLFile1.xml" );
- }
- //このメソッドには、ウィンドウに表示されるデータソースを示すためのすべてのロジックが含まれます
- private void Change_DataSource( オブジェクト送信者、RoutedEventArgs e)
- {
- if (rbDatabase.IsChecked == true ) //データベースがデータソースとして選択されます
- {
- listBooks.ItemTemplate = null ;
- treeStructure.ItemsSource = new MyTestDbDataContext()。Categories.Where(n => n.ParrentCategoryId == null );
- treeStructure.ItemTemplate =(HierarchicalDataTemplate)FindResource( " key1 " );
- //メモのバインドを設定します
- DescriptionBinding(selectedNodeDescription、 「SelectedItem.Description」 、treeStructure);
- DescriptionBinding(selectedBookDescription、 "SelectedItem.Description" 、listBooks);
- //ブックの表示バインディングを設定します
- バインドbind = new Binding( "SelectedItem.Books" ){Source = treeStructure};
- listBooks.SetBinding(ItemsControl.ItemsSourceProperty、バインド);
- }
- else //データソースとしてxmlファイルが選択されます
- {
- treeStructure.ItemsSource = xml.Elements( "Category" );
- treeStructure.ItemTemplate =(HierarchicalDataTemplate)FindResource( "key2" );
- //メモのバインドを設定します
- DescriptionBinding(selectedNodeDescription、 「SelectedItem.Attribute [Description] .Value」 、treeStructure);
- DescriptionBinding(selectedBookDescription、 「SelectedItem.Attribute [Description] .Value」 、listBooks);
- //ブックの表示バインディングを設定します
- listBooks.ItemTemplate =(DataTemplate)FindResource( "key3" );
- バインドbind = new Binding( "SelectedItem.Elements [Book]" ){Source = treeStructure};
- listBooks.SetBinding(ItemsControl.ItemsSourceProperty、バインド);
- }
- }
- /// <summary>
- ///データ表示バインディングを構成する
- /// </ summary>
- /// <param name = "textBlock">ノートテキストを表示するテキストオブジェクト</ param>
- /// <param name = "pathValue">パスバインディングの値</ param>
- /// <param name = "source"> Pathプロパティを通じてデータが読み取られるソースオブジェクトへの参照</ param>
- void DescriptionBinding(TextBlock textBlock、 string pathValue、コントロールソース)
- {
- textBlock.SetBinding(TextBlock.TextProperty、 新しいバインディング(pathValue){ソース=ソース});
- }
- }
- }
これで、アプリケーションを実行して実行し、作業の結果を楽しむことができます... F5キーを押して、何があるかを確認します...
A.ソースはデータベースです。
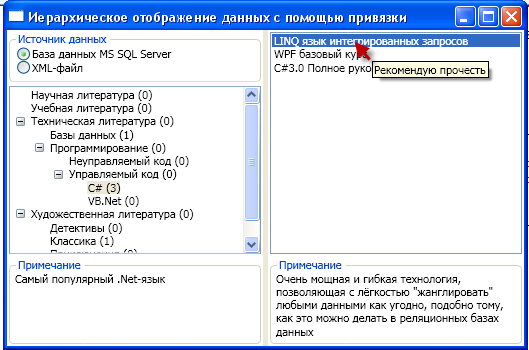
B.ソースはxmlファイルです。

MS Visual Studio 2010形式のソリューション(ソリューション)のソースコードは、 ここからダウンロードできます 。