最近、アイデアが思いつきました-最小限の行数で簡単なゲームを書くことです。 私の選択はテトリスにかかった。 この記事では、コードについて説明します。
そもそも、実装には基本的な機能のみが含まれていることに注意してください。
- 数字の左右の動き;
- 落下図;
- 数字の回転;
- 塗りつぶされた図を削除します。
- ゲームの終わり。
そのため、最初にPictureBoxをフォームに追加し、タイマーを作成します。
また、ゲームには次のものが必要です。
public const int width = 15, height = 25, k = 15; // public int[,] shape = new int[2, 4]; // ( 2 [0, i] [1, i] public int[,] field = new int[width, height]; // public Bitmap bitfield = new Bitmap(k * (width + 1) + 1, k * (height + 3) + 1); public Graphics gr; // PictureBox
端のフィールドを埋めます:
for (int i = 0; i < width; i++) field[i, height - 1] = 1; for (int i = 0; i < height; i++) { field[0, i] = 1; field[width - 1, i] = 1; }
図を埋める:
public void SetShape(){ Random x = new Random(DateTime.Now.Millisecond); switch (x.Next(7)){ // 1 7 case 0: shape = new int[,] { { 2, 3, 4, 5 }, { 8, 8, 8, 8 } }; break; case 1: shape = new int[,] { { 2, 3, 2, 3 }, { 8, 8, 9, 9 } }; break; case 2: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 9 } }; break; case 3: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 7 } }; break; case 4: shape = new int[,] { { 3, 3, 4, 4 }, { 7, 8, 8, 9 } }; break; case 5: shape = new int[,] { { 3, 3, 4, 4 }, { 9, 8, 8, 7 } }; break; case 6: shape = new int[,] { { 3, 4, 4, 4 }, { 8, 7, 8, 9 } }; break; } }
PictureBoxに「ガラス」を描く手順:
public void FillField(){ gr.Clear(Color.Black); // for (int i = 0; i < width; i++) for (int j = 0; j < height; j++) if (field[i, j] == 1){ // gr.FillRectangle(Brushes.Green, i * k, j * k, k, k); // gr.DrawRectangle(Pens.Black, i * k, j * k, k, k); } for (int i = 0; i < 4; i++){ // gr.FillRectangle(Brushes.Red, shape[1, i] * k, shape[0, i] * k, k, k); gr.DrawRectangle(Pens.Black, shape[1, i] * k, shape[0, i] * k, k, k); } FieldPictureBox.Image = bitfield; }
最初に図を移動してから、このオプションが可能かどうかを確認します。 ある場所でエラーが発生した場合(図がフィールドの外にあるか、すでにフィールドにある図に重ねられている)、図は元の場所に戻ります。 これを行うために、フィールドでエラーが見つかった場合はtrueを返し、エラーがない場合はfalseを返す関数を作成しました。
public bool FindMistake(){ for (int i = 0; i < 4; i++) if (shape[1, i] >= width || shape[0, i] >= height || shape[1, i] <= 0 || shape[0, i] <= 0 || field[shape[1, i], shape[0, i]] == 1) return true; return false; }
それでは、図に移りましょう。 コンストラクターでKeyDownイベントを作成します。
左に移動:
switch (e.KeyCode){ case Keys.A: for (int i = 0; i < 4; i++) shape[1, i]--; // 1 OX if (FindMistake()) // for (int i = 0; i < 4; i++) shape[1, i]++; // 1 break; ... }
同様に、右への移動:
case Keys.D: for (int i = 0; i < 4; i++) shape[1, i]++; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]--; break;
図の反転はもう少し複雑です:
case Keys.W: var shapeT = new int[2, 4]; Array.Copy(shape, shapeT, shape.Length); // , , , , int maxx = 0, maxy = 0; for (int i = 0; i < 4; i++){ if (shape[0, i] > maxy) maxy = shape[0, i]; if (shape[1, i] > maxx) maxx = shape[1, i]; } // X Y for (int i = 0; i < 4; i++) { int temp = shape[0, i]; shape[0, i] = maxy - (maxx - shape[1, i]) - 1; shape[1, i] = maxx - (3 - (maxy - temp)) + 1; } // . . if (FindMistake()) Array.Copy(shapeT, shape, shape.Length); break;
現在は、図を追加して線を削除するだけです。 これはすべて、TimerTickイベントで発生します。
private void TickTimer_Tick(object sender, System.EventArgs e){ if (field[8, 3] == 1) Environment.Exit(0); // , , . for (int i = 0; i < 4; i++) shape[0, i]++; // if (FindMistake()){ for (int i = 0; i < 4; i++) field[shape[1, i], --shape[0, i]]++; SetShape(); } // , 1 , field for (int i = height - 2; i > 2; i--){ var cross = (from t in Enumerable.Range(0, field.GetLength(0)).Select(j => field[j, i]).ToArray() where t == 1 select t).Count(); // if (cross == width) for (int k = i; k > 1; k--) for (int l = 1; l < width - 1; l++) field[l, k] = field[l, k - 1]; } // , , , , , 1 FillField(); // }
そして最後に、フォームを作成するときに、次を呼び出す必要があります。
SetShape();
最終コード:
using System; using System.Linq; using System.Drawing; using System.Windows.Forms; namespace LittleTetris{ public partial class Form1 : Form{ public const int width = 15, height = 25, k = 15; public int[,] shape = new int[2, 4]; public int[,] field = new int[width, height]; public Bitmap bitfield = new Bitmap(k * (width + 1) + 1, k * (height + 3) + 1); public Graphics gr; public Form1(){ InitializeComponent(); gr = Graphics.FromImage(bitfield); for (int i = 0; i < width; i++) field[i, height - 1] = 1; for (int i = 0; i < height; i++) { field[0, i] = 1; field[width - 1, i] = 1; } SetShape(); } public void FillField(){ gr.Clear(Color.Black); for (int i = 0; i < width; i++) for (int j = 0; j < height; j++) if (field[i, j] == 1){ gr.FillRectangle(Brushes.Green, i * k, j * k, k, k); gr.DrawRectangle(Pens.Black, i * k, j * k, k, k); } for (int i = 0; i < 4; i++){ gr.FillRectangle(Brushes.Red, shape[1, i] * k, shape[0, i] * k, k, k); gr.DrawRectangle(Pens.Black, shape[1, i] * k, shape[0, i] * k, k, k); } FieldPictureBox.Image = bitfield; } private void TickTimer_Tick(object sender, System.EventArgs e){ if (field[8, 3] == 1) Environment.Exit(0); for (int i = 0; i < 4; i++) shape[0, i]++; for (int i = height - 2; i > 2; i--){ var cross = (from t in Enumerable.Range(0, field.GetLength(0)).Select(j => field[j, i]).ToArray() where t == 1 select t).Count(); if (cross == width) for (int k = i; k > 1; k--) for (int l = 1; l < width - 1; l++) field[l, k] = field[l, k - 1];} if (FindMistake()){ for (int i = 0; i < 4; i++) field[shape[1, i], --shape[0, i]]++; SetShape();} FillField(); } private void Form1_KeyDown(object sender, KeyEventArgs e){ switch (e.KeyCode){ case Keys.A: for (int i = 0; i < 4; i++) shape[1, i]--; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]++; break; case Keys.D: for (int i = 0; i < 4; i++) shape[1, i]++; if (FindMistake()) for (int i = 0; i < 4; i++) shape[1, i]--; break; case Keys.W: var shapeT = new int[2, 4]; Array.Copy(shape, shapeT, shape.Length); int maxx = 0, maxy = 0; for (int i = 0; i < 4; i++){ if (shape[0, i] > maxy) maxy = shape[0, i]; if (shape[1, i] > maxx) maxx = shape[1, i]; } for (int i = 0; i < 4; i++) { int temp = shape[0, i]; shape[0, i] = maxy - (maxx - shape[1, i]) - 1; shape[1, i] = maxx - (3 - (maxy - temp)) + 1; } if (FindMistake()) Array.Copy(shapeT, shape, shape.Length); break; } } public void SetShape(){ Random x = new Random(DateTime.Now.Millisecond); switch (x.Next(7)){ case 0: shape = new int[,] { { 2, 3, 4, 5 }, { 8, 8, 8, 8 } }; break; case 1: shape = new int[,] { { 2, 3, 2, 3 }, { 8, 8, 9, 9 } }; break; case 2: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 9 } }; break; case 3: shape = new int[,] { { 2, 3, 4, 4 }, { 8, 8, 8, 7 } }; break; case 4: shape = new int[,] { { 3, 3, 4, 4 }, { 7, 8, 8, 9 } }; break; case 5: shape = new int[,] { { 3, 3, 4, 4 }, { 9, 8, 8, 7 } }; break; case 6: shape = new int[,] { { 3, 4, 4, 4 }, { 8, 7, 8, 9 } }; break; } } public bool FindMistake(){ for (int i = 0; i < 4; i++) if (shape[1, i] >= width || shape[0, i] >= height || shape[1, i] <= 0 || shape[0, i] <= 0 || field[shape[1, i], shape[0, i]] == 1) return true; return false; } } }
その結果、FindMistakeで閉じ括弧とペイントされた状態の行をカウントしない場合、95行になります。
実行中のプログラムのスクリーンショットは次のとおりです。
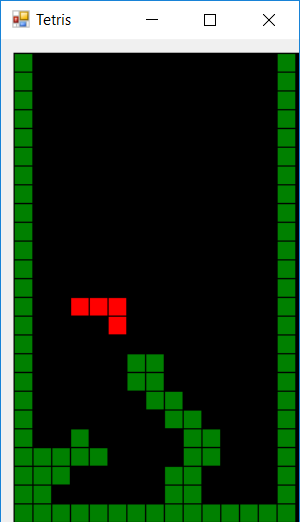