aiohttpアプリケーションの作成
簡単なものから始めましょう-aiohttpをインストールして実行します。 まず、仮想環境を作成します。 Python 3.5を使用することをお勧めします。これは、新しいasync defおよびawait構文を導入します。 非同期プログラミングをよりよく理解するために将来このアプリケーションを開発したい場合は、Python 3.6をすぐにインストールできます。 最後に、aiohttpをインストールします。
pip install aiohttp
from aiohttp import web
async def get_mars_photo(request):
return web.Response(text='A photo of Mars')
app = web.Application()
app.router.add_get('/', get_mars_photo, name='mars_photo')
- get_mars_photo — ; HTTP HTTP ( )
- app — ; , middleware ( )
- app.router.add_get — HTTP GET '/'
web.run_app(app, host='127.0.0.1', port=8080)
python nasa.py
, . aiohttp-devtools. runserver, , live reloading.
pip install aiohttp-devtools adev runserver -p 8080 nasa.py
API NASA
. API NASA. URL ( Curiosity api.nasa.gov/mars-photos/api/v1/rovers/curiosity/photos [1]). 2 :
- sol — , , ( rover/max_sol)
- API KEY — , NASA ( DEMO_KEY)
import random
from aiohttp import web, ClientSession
from aiohttp.web import HTTPFound
NASA_API_KEY = 'DEMO_KEY'
ROVER_URL = 'https://api.nasa.gov/mars-photos/api/v1/rovers/curiosity/photos'
async def get_mars_image_url_from_nasa():
while True:
sol = random.randint(0, 1722)
params = {'sol': sol, 'api_key': NASA_API_KEY}
async with ClientSession() as session:
async with session.get(ROVER_URL, params=params) as resp:
resp_dict = await resp.json()
if 'photos' not in resp_dict:
raise Exception
photos = resp_dict['photos']
if not photos:
continue
return random.choice(photos)['img_src']
async def get_mars_photo(request):
url = await get_mars_image_url_from_nasa()
return HTTPFound(url)
- sol ( Curiosity max_sol 1722)
- ClientSession , NASA API
- JSON resp.json()
- 'photos' ; , ,
- ,
- HTTPFound
NASA API
DEMO_KEY, NASA , , [2]. , .
:

, …
- . , . , . .
- . :
async def get_mars_photo_bytes():
while True:
image_url = await get_mars_image_url_from_nasa()
async with ClientSession() as session:
async with session.get(image_url) as resp:
image_bytes = await resp.read()
if await validate_image(image_bytes):
break
return image_bytes
async def get_mars_photo(request):
image = await get_mars_photo_bytes()
return web.Response(body=image, content_type='image/jpeg')
- url resp.read()
- , web.Response. — body text, content_type
(HTTPFound), .
. — .
Pillow:
pip install pillow
import io
from PIL import Image
async def validate_image(image_bytes):
image = Image.open(io.BytesIO(image_bytes))
return image.width >= 1024 and image.height >= 1024
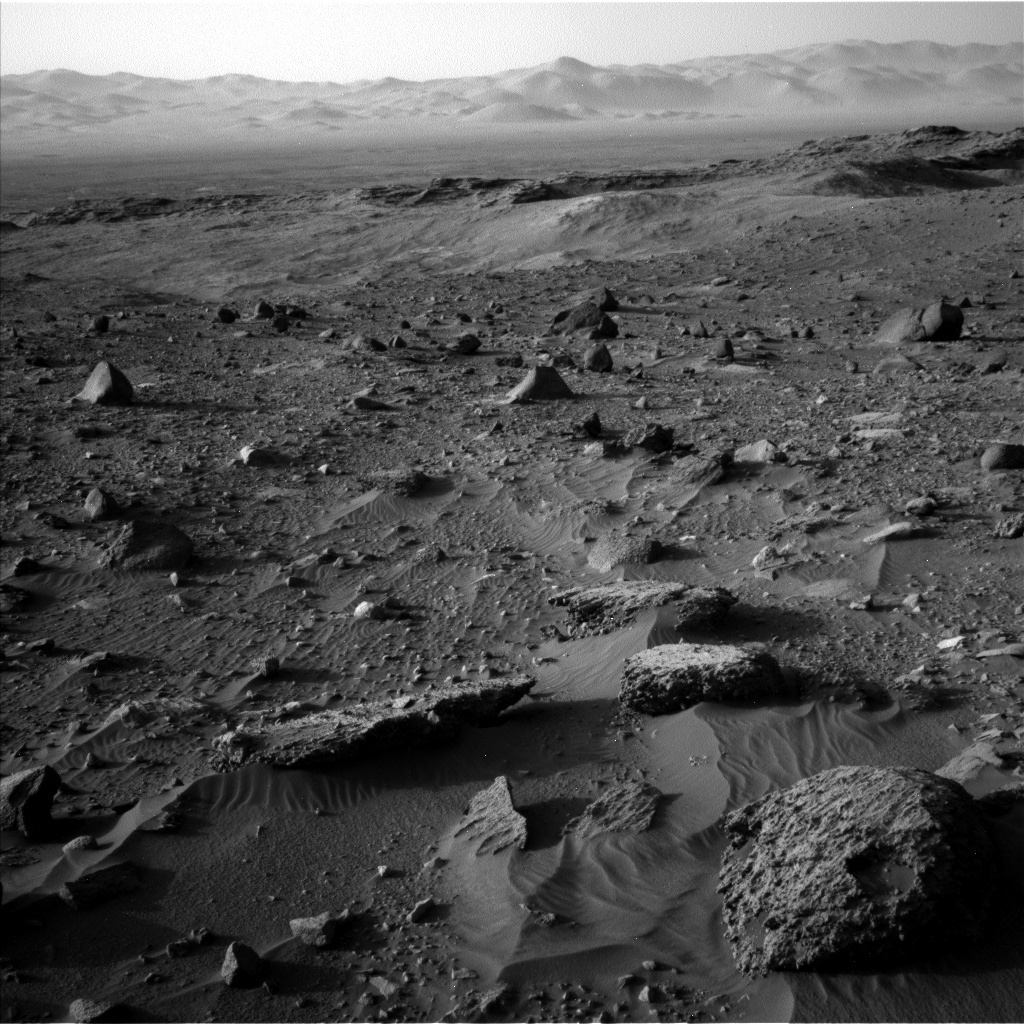
async def validate_image(image_bytes):
image = Image.open(io.BytesIO(image_bytes))
return image.width >= 1024 and image.height >= 1024 and image.mode != 'L'
:

:

#!/usr/bin/env python3
import io
import random
from aiohttp import web, ClientSession
from aiohttp.web import HTTPFound
from PIL import Image
NASA_API_KEY = 'DEMO_KEY'
ROVER_URL = 'https://api.nasa.gov/mars-photos/api/v1/rovers/curiosity/photos'
async def validate_image(image_bytes):
image = Image.open(io.BytesIO(image_bytes))
return image.width >= 1024 and image.height >= 1024 and image.mode != 'L'
async def get_mars_image_url_from_nasa():
while True:
sol = random.randint(0, 1722)
params = {'sol': sol, 'api_key': NASA_API_KEY}
async with ClientSession() as session:
async with session.get(ROVER_URL, params=params) as resp:
resp_dict = await resp.json()
if 'photos' not in resp_dict:
raise Exception
photos = resp_dict['photos']
if not photos:
continue
return random.choice(photos)['img_src']
async def get_mars_photo_bytes():
while True:
image_url = await get_mars_image_url_from_nasa()
async with ClientSession() as session:
async with session.get(image_url) as resp:
image_bytes = await resp.read()
if await validate_image(image_bytes):
break
return image_bytes
async def get_mars_photo(request):
image = await get_mars_photo_bytes()
return web.Response(body=image, content_type='image/jpeg')
app = web.Application()
app.router.add_get('/', get_mars_photo, name='mars_photo')
web.run_app(app, host='127.0.0.1', port=8080)
(, max_sol API, , URL), : .
, . , .
:
[1] 3, api.nasa.gov/mars-photos/api/v1/rovers/?API_KEY=DEMO_KEY.
[2] , -