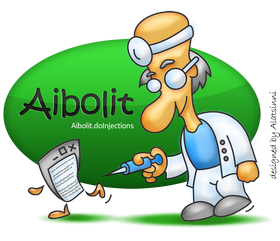
特徴
- ビューの初期化;
- ビューのイベントライサーを追加します。
- アプリケーションリソースの初期化:描画可能、文字列、アニメーション、ブール、次元、整数、配列、色、配列アダプター。
- システムサービスの初期化。
- アプリケーションのアプリケーションサービスの初期化。
使用例
依存関係を接続します。
repositories { maven { url 'https://dl.bintray.com/alexeydanilov/maven' } } dependencies { compile 'com.danikula:aibolit:1.0' }
注入する
public class AibolitChatActivity extends Activity { // annotate fields to be injected... @InjectView(R.id.messageEditText) private EditText messageEditText; @InjectView(R.id.historyListView) private ListView historyListView; @InjectResource(R.string.symbols_count) private String symbolsCountPattern; @InjectSystemService(Context.NOTIFICATION_SERVICE) private NotificationManager notificationManager; @InjectService private HttpManager httpManager; @InjectResource(R.layout.content) private View content; ... @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.chat_activity); // initialize annotated fields and methods Aibolit.doInjections(this); // or just Aibolit.setInjectedContentView(this); ... } // annotate event handlers... @InjectOnClickListener(R.id.sendButton) private void onSendButtonClick(View v) { // handle onClick event } @InjectOnClickListener(R.id.clearHistoryButton) private void onClearHistoryButtonClick(View v) { // handle onClick event } @InjectOnTextChangedListener(R.id.messageEditText) public void onMessageTextChanged(CharSequence s, int start, int before, int count) { // handle text changed event } ... }
コードは非常にシンプルで、findViewById、setOnClickListenerメソッドなどの呼び出しが完全に軽減されています。 代わりに:
- InjectViewアノテーションを使用して初期化する必要があるビューをマークします。
- イベントハンドラを定義し、対応するInjectOn *アノテーションでマークします。 この例では、ボタンをクリックしてテキストを変更するためのイベントハンドラーが定義されています。 Aibolitでは、すべての主要なイベントにハンドラーを追加できます。
OnClick、OnLongClick、OnTouch、OnKey、OnTextChanged、OnCheckedChange、OnFocusChange、OnItemClick、OnItemSelected、OnEditorAction、OnCreateContextMenu; -
Aibolit.doInjections(this);
メソッドを呼び出しAibolit.doInjections(this);
アクティベーション用のコンテンツをインストールした後。
2つのメソッドの呼び出し
setContentView(layoutId); Aibolit.doInjections(this);
1つに置き換えることができます:
Aibolit.setInjectedContentView(this);
Aibolitでは、上記の例で行われているように、アプリケーションアプリケーションサービスを注入することもできます。
@InjectService private HttpManager httpManager;
これについては、Aibolitクラスのドキュメントをご覧ください。
仕組み
コードソースは公開され 、文書化されています。 舞台裏で何が起こっていますか? Aibolitは、Inject *アノテーションでマークされたフィールドとメソッドのクラスを分析します。 各アノテーションには独自のインジェクタークラスがあり、1つまたは別のリソースでフィールドを初期化します。 以下は、ビューの初期化を担当するこのようなインジェクターの例です。
class ViewInjector extends AbstractFieldInjector<InjectView> { @Override public void doInjection(Object fieldOwner, InjectionContext injectionContext, Field field, InjectView annotation) { int viewId = annotation.value(); View view = injectionContext.getRootView().findViewById(viewId); if (view == null) { // throw exception... } if (!field.getType().isAssignableFrom(view.getClass())) { // throw exception... } try { field.setAccessible(true); field.set(fieldOwner, view); } catch (IllegalArgumentException e) { // throw exception... } catch (IllegalAccessException e) { // throw exception... } } }
性能
ホリバーの理由を述べないために、私はちょうど乾燥した数字を与えます。 測定はエミュレータで行われました。 35の要素(ビュー、ライセンシー、アプリケーションリソース)を初期化する必要がありました。 従来のアプローチを使用する場合、1つのアクティビティから別のアクティビティへの移行には40〜50ミリ秒が必要でしたが、aibolitの使用は90〜100ミリ秒でした。 予想どおり、リフレクションベースのアプローチは時間がかかりますが、得られた違いIMHOは重要ではありません。
Aibolitは、完成品としても、ソリューションを作成するための基礎としても使用できます。 ライブラリコードは公開されており、Apacheライセンスバージョン2.0の下で配布されています。
私はコメントと提案を喜んでいます。
UPD 0:
同様のライブラリ
ロボギス
androidannotations